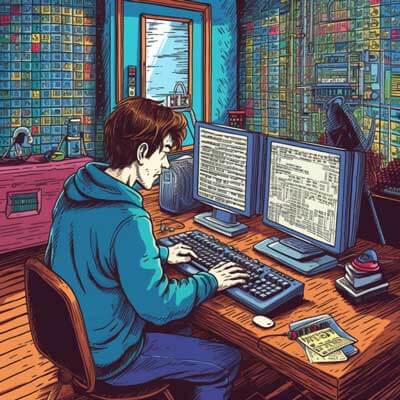
Table of Contents
Overview of Reduction Methods
Reduction is a fundamental concept in computer programming that involves reducing a collection of elements to a single value. Python provides several methods for performing reduction operations. These methods allow us to process and extract meaningful information from large datasets. In this guide, we will explore different reduction techniques in Python and understand how they can be applied in real-world scenarios.
Related Article: Python Sort Dictionary Tutorial
Comparison of Reduction in Python and Other Languages
Python's reduction methods offer a wide range of functionality and flexibility, making it a popular choice among developers. Compared to other programming languages, such as Java or C++, Python provides concise syntax and built-in functions that simplify the reduction process. Additionally, Python's extensive library ecosystem, including NumPy and Pandas, further enhances its capabilities for reduction operations.
Real-world Applications of Reduction
Reduction techniques find applications in various domains, including data analysis, machine learning, and optimization problems. For instance, in data analysis, reduction methods can be used to calculate summary statistics like mean, median, and standard deviation. In machine learning, reduction can be applied to extract important features from high-dimensional datasets, reducing the complexity of the problem. Furthermore, reduction techniques are commonly used in optimization problems to minimize or maximize objective functions.
Code Snippet: Minimization Techniques
To showcase the application of reduction techniques, let's consider a scenario where we have a list of numbers and we want to find the minimum value using Python. The following code snippet demonstrates how the built-in min()
function can be used for this purpose:
numbers = [5, 2, 9, 1, 7] minimum = min(numbers) print(f"The minimum value is {minimum}")
In this example, the min()
function takes a list of numbers as input and returns the smallest value. By applying this reduction method, we can easily find the minimum value without writing complex code.
Related Article: Python Ceiling Function Explained
Code Snippet: Optimization Strategies
Optimization problems often involve finding the maximum or minimum value of a function over a given range. Python provides several optimization libraries, such as SciPy and PyOpt, which offer useful tools for solving such problems. Here is an example of using the scipy.optimize.minimize()
function to minimize a mathematical function:
from scipy.optimize import minimize def objective(x): return x**2 + 3*x + 2 result = minimize(objective, 0) minimum_value = result.fun print(f"The minimum value is {minimum_value}")
In this code snippet, the minimize()
function takes the objective function and an initial guess as input and returns the optimal solution. By leveraging optimization strategies, we can efficiently find the minimum or maximum value of complex functions.
Code Snippet: Simplification Approaches
In certain scenarios, we may need to simplify or aggregate data to extract meaningful insights. Python provides various techniques for data simplification, such as grouping and summarizing data. The following code snippet demonstrates how we can use the groupby()
function from the Pandas library to simplify data by grouping:
import pandas as pd data = {'Name': ['John', 'Alice', 'Bob', 'John', 'Alice'], 'Age': [25, 30, 35, 28, 32], 'Salary': [50000, 60000, 70000, 55000, 65000]} df = pd.DataFrame(data) grouped_data = df.groupby('Name').mean() print(grouped_data)
In this example, we have a dataset containing information about employees. By grouping the data by the "Name" column and calculating the mean of other columns, we can simplify the data and gain insights about the average age and salary for each employee.
Code Snippet: Streamlining Examples
Streamlining examples is a common use case for reduction in Python. It involves processing a large collection of elements and selecting specific elements that meet certain criteria. The following code snippet demonstrates how we can streamline a list of numbers to select only the even numbers using a list comprehension:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = [x for x in numbers if x % 2 == 0] print(even_numbers)
In this example, we iterate over the list of numbers and select only the elements that are divisible by 2 (i.e., even numbers). By using list comprehension, we can streamline the process of selecting specific elements from a collection.
Code Snippet: Shrinking Methods
Shrinking methods involve reducing the size or dimensionality of data while preserving important information. Principal Component Analysis (PCA) is a popular technique for dimensionality reduction in Python. The following code snippet demonstrates how we can apply PCA using the sklearn.decomposition.PCA
class:
from sklearn.decomposition import PCA import numpy as np data = np.random.rand(100, 10) # Random data of shape (100, 10) pca = PCA(n_components=3) reduced_data = pca.fit_transform(data) print(reduced_data.shape)
In this example, we generate random data of shape (100, 10) and apply PCA to reduce its dimensionality to 3. By using shrinking methods like PCA, we can effectively reduce the dimensionality of high-dimensional datasets while retaining important information.
Related Article: How to Use Regex to Match Any Character in Python
Additional Resources
- Python reduce() function