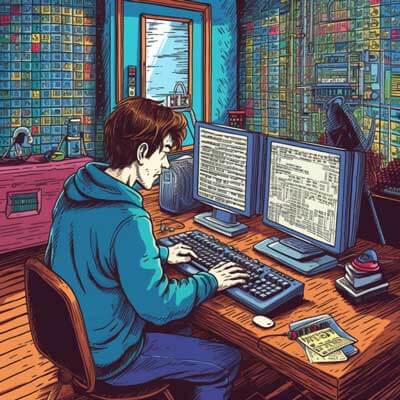
Table of Contents
In today's globalized world, it is essential for software applications to support multiple languages and handle different character encodings. Elixir, a useful and functional programming language, provides a robust framework called Phoenix for building web applications. In this article, we will explore how Phoenix handles internationalization and encoding, best practices for managing encodings in Elixir, and how to utilize Gettext for Phoenix application localization. We will also discuss Unicode and its impact on encoding in Elixir, common challenges in internationalization of Elixir applications, available libraries and tools for handling encodings, ensuring proper encoding support in Phoenix applications, and serving content dynamically based on user's language preferences.
How does Phoenix handle localization?
Phoenix provides built-in support for localization through its useful localization library called Gettext. Gettext allows developers to easily extract and manage translation strings in their application. Phoenix uses the concept of "gettext domains" to organize translations and handle different languages.
To enable localization in a Phoenix application, you need to follow these steps:
1. Install the gettext
package by adding it to your mix.exs
file:
defp deps do [ {:gettext, "~> 0.17"} ] end
2. Run mix deps.get
to fetch the dependency.
3. Configure the gettext
library in your config/config.exs
file:
config :gettext, default_locale: "en", default_domain: "my_app", translations: [ MyApp.Gettext ]
4. Create a Gettext
module that defines the translation strings for your application. For example, if you have a module called MyApp.User
, you can define translations in the Gettext
module as follows:
defmodule MyApp.Gettext do use Gettext, otp_app: :my_app def translations do [ "Hello" => "Bonjour", "Sign In" => "Se connecter" ] end end
5. Start using translations in your views and templates. For example:
6. Generate the translation files by running mix gettext.extract
and mix gettext.merge
.
With these steps, Phoenix handles localization by using the Gettext library and providing a seamless way to translate strings in your application.
Related Article: Exploring Phoenix: Umbrella Project Structures,Ecto & More
What are the best practices for managing encodings in Elixir?
When dealing with encodings in Elixir, there are several best practices to follow to ensure proper handling of data:
1. Use UTF-8 as the default encoding: UTF-8 is a widely supported and recommended encoding for handling Unicode characters. It can represent almost all characters in any language. Make sure to set UTF-8 as the default encoding in your Elixir application.
2. Validate and sanitize user input: When accepting user input, especially from web forms, it is crucial to validate and sanitize the data to prevent any potential encoding issues or security vulnerabilities.
3. Normalize text: Normalization is the process of transforming text into a canonical form. This can help in handling different encodings and ensuring consistency in your data. Elixir provides the String.normalize/2
function for normalizing Unicode strings.
4. Avoid mixing encodings: Mixing different encodings within the same application or data can lead to encoding-related issues. It is recommended to stick to a single encoding, preferably UTF-8, throughout your application.
5. Use proper encoding conversion functions: When converting between different encodings, make sure to use the appropriate conversion functions provided by Elixir, such as String.to_charlist/1
, String.to_string/1
, and String.downcase/1
.
How to utilize Gettext for Phoenix application localization?
Gettext is a useful localization library in Elixir that allows developers to easily manage translations in their Phoenix applications. It provides a seamless way to extract, update, and use translation strings. Here's how you can utilize Gettext for Phoenix application localization:
1. Define translation strings: In your Phoenix application, you can define translation strings using the Gettext
module. This module acts as a container for all your translation strings. For example:
defmodule MyApp.Gettext do use Gettext, otp_app: :my_app def translations do [ "Hello" => "Bonjour", "Sign In" => "Se connecter" ] end end
2. Extract translation strings: Gettext provides a mix task called mix gettext.extract
that automatically extracts translation strings from your source code and generates translation files. Run this task to extract the translation strings:
mix gettext.extract
3. Update translation files: Gettext generates translation files for each language defined in your application. These files are located in the priv/gettext
directory. You can manually update these files with the translated strings or use a translation management tool to streamline the translation process.
4. Use translations in your views and templates: Once the translation files are updated, you can use the translation strings in your views and templates by calling the gettext/1
function. For example:
Gettext will automatically choose the translation based on the current locale defined in your application.
What is Unicode and how does it affect encoding in Elixir?
Unicode is a character encoding standard that aims to represent every character from all writing systems in the world. It provides a unique code point for each character, allowing software applications to handle text in different languages and scripts.
In Elixir, Unicode plays a crucial role in encoding and manipulating strings. Elixir uses UTF-8 as the default encoding, which is a variable-width encoding that can represent any Unicode character. This means that Elixir can handle text in different languages, including non-Latin scripts such as Chinese, Arabic, and Russian.
When working with Unicode in Elixir, you can perform various operations on strings, such as concatenation, slicing, and transformation. Elixir provides a rich set of functions in the String
module for working with Unicode strings. For example:
- String.length/1
: Returns the number of Unicode code points in a string.
- String.slice/3
: Extracts a substring from a string based on Unicode code point indices.
- String.upcase/1
and String.downcase/1
: Converts a string to uppercase or lowercase, respectively, based on Unicode rules.
Unicode in Elixir allows developers to handle text in a consistent and reliable manner, regardless of the language or script used.
Related Article: Deployment Strategies and Scaling for Phoenix Apps
What are some common challenges in internationalization of Elixir applications?
Internationalizing Elixir applications can come with its own set of challenges. Some common challenges include:
1. Handling different encodings: Elixir applications need to handle text in various encodings to support different languages and scripts. It is important to ensure proper encoding support and handle any potential encoding-related issues.
2. Translating and managing large sets of strings: Translating a large number of strings and managing them can be a complex task. It requires careful organization, coordination, and efficient processes to handle translations effectively.
3. Pluralization and grammatical rules: Different languages have different rules for pluralization and grammatical forms. Handling these variations can be challenging, especially when building dynamic strings that depend on variables or context.
4. Date and time formats: Date and time formats vary across different cultures and regions. Elixir provides the Timex
library, which offers comprehensive support for handling date and time values in a localized manner.
5. Right-to-left languages: Elixir applications may need to support right-to-left (RTL) languages such as Arabic and Hebrew. Handling RTL text layout and bidirectional text can be challenging, requiring special attention to ensure proper rendering and user experience.
To overcome these challenges, it is important to follow best practices, use appropriate libraries and tools, and thoroughly test internationalized features in your Elixir applications.
Are there any libraries or tools available for handling encodings in Elixir?
Yes, Elixir provides several libraries and tools for handling encodings and working with Unicode. Some of the popular ones are:
1. String
: Elixir's standard library provides a rich set of functions in the String
module for manipulating and working with Unicode strings. These functions allow you to perform various operations such as concatenation, slicing, case conversion, and normalization.
2. Gettext
: Gettext is a useful localization library in Elixir that allows developers to easily manage translations, including handling different encodings. It provides functions for translating strings and managing translation files.
3. Timex
: Timex is a comprehensive library for handling date and time values in Elixir. It offers extensive support for localized date and time formatting, including support for different date and time formats used in various cultures.
4. Unicode
: The Unicode library provides functions for working with Unicode code points, characters, and properties. It allows you to perform operations such as character classification, normalization, and case mapping.
These libraries and tools provide developers with the necessary functionality to handle encodings, work with Unicode, and build internationalized applications in Elixir.
How can I ensure proper encoding support in my Phoenix application?
To ensure proper encoding support in your Phoenix application, you can follow these best practices:
1. Set the default encoding to UTF-8: UTF-8 is a widely supported and recommended encoding for handling Unicode characters. Make sure to set UTF-8 as the default encoding in your Phoenix application. You can do this by configuring the encoding in your application's configuration file, such as config/config.exs
:
config :my_app, :encoding, "utf8"
2. Validate and sanitize user input: When accepting user input, especially from web forms, it is crucial to validate and sanitize the data to prevent any potential encoding issues or security vulnerabilities. Use validation libraries or functions provided by Phoenix, such as changeset/2
and cast/3
, to ensure the integrity of the input data.
3. Use appropriate database settings: If your application interacts with a database, make sure to configure the database connection with the correct encoding settings. For example, in a PostgreSQL database, you can set the encoding in the config/config.exs
file:
config :my_app, MyApp.Repo, adapter: Ecto.Adapters.Postgres, username: "postgres", password: "password", database: "my_app", encoding: "utf8"
4. Normalize text: Normalization is the process of transforming text into a canonical form. This can help in handling different encodings and ensuring consistency in your data. Elixir provides the String.normalize/2
function for normalizing Unicode strings.
What are the key features of Elixir for application development?
Elixir is a useful and functional programming language that offers a wide range of features for application development. Some key features of Elixir include:
1. Concurrency and scalability: Elixir is built on the Erlang virtual machine (BEAM), which is known for its lightweight processes and excellent support for concurrency. Elixir provides constructs such as processes, message passing, and supervision trees, making it easy to build highly concurrent and scalable applications.
2. Functional programming: Elixir is a functional programming language, which means it emphasizes immutability and pure functions. This makes it easier to reason about code, write testable software, and build robust applications.
3. Metaprogramming: Elixir has useful metaprogramming capabilities that allow developers to write code that generates code. This enables advanced features such as macros, which can be used to extend the language and provide domain-specific abstractions.
4. Pattern matching and destructuring: Elixir provides useful pattern matching and destructuring capabilities. This allows developers to write expressive and concise code, making it easier to handle complex data structures and control flow.
5. Fault-tolerant and hot-swappable code: Elixir applications can be designed to be fault-tolerant using the supervision tree mechanism. This allows for the automatic restart of failed processes, ensuring high availability and minimal downtime. Elixir also supports hot code swapping, allowing you to upgrade your application without stopping it.
6. Interoperability with other languages: Elixir can seamlessly interoperate with other languages such as Erlang, C, and JavaScript. This allows you to leverage existing libraries and resources, making it easier to integrate Elixir into existing systems.
These key features of Elixir make it a useful language for building web applications, distributed systems, and real-time applications.
Related Article: Implementing Enterprise Features with Phoenix & Elixir
How can content be dynamically served based on user's language preferences in a Phoenix application?
Phoenix provides built-in support for dynamically serving content based on a user's language preferences. This allows you to provide a localized experience to your users. Here's how you can achieve this in a Phoenix application:
1. Set up language negotiation: Phoenix uses the accept-language
header sent by the client to determine the user's preferred languages. By default, Phoenix extracts this information and stores it in the conn
struct under conn.private.accepted_languages
. You can access this information in your controller actions or views.
2. Define translations for each language: In your Gettext module, define translations for each language that your application supports. For example, if your application supports English and French, you can define translations as follows:
defmodule MyApp.Gettext do use Gettext, otp_app: :my_app def translations do [ "Hello" => "Bonjour", "Sign In" => "Se connecter" ] end end
3. Use translations in your views and templates: Once you have defined translations for each language, you can use them in your views and templates. Phoenix provides a helper function called gettext/2
that takes the translation key and the user's preferred languages as arguments. It automatically chooses the appropriate translation based on the user's preferences. For example:
4. Set the language for the user: Phoenix provides a put_private/3
function that allows you to store data in the conn
struct's private
field. You can use this function to set the user's preferred language for future requests. For example:
conn = put_private(conn, :preferred_language, "fr")
5. Create language switcher: To allow users to change their preferred language, you can create a language switcher in your application. This can be a dropdown menu or a list of available languages. When the user selects a different language, you can update the conn.private.accepted_languages
field and set the user's preferred language accordingly.