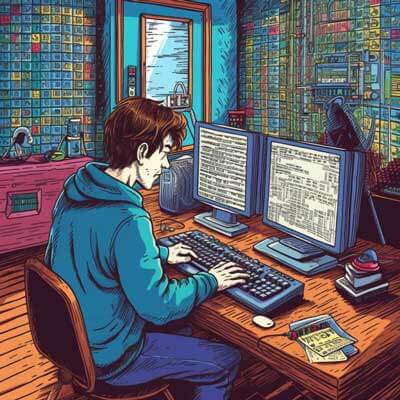
- What are some considerations for handling large volume data with Gin?
- How can data streaming be implemented in a Gin application?
- What are some pagination techniques for handling large datasets in Gin?
- How can bulk data operations be performed in a Gin application?
- What is background processing and how is it useful in Gin applications?
- What are asynchronous tasks and how are they used in Gin?
- How can ORM configurations be optimized for handling large datasets in Gin?
- What are some best practices for working with large datasets in Gin?
- Additional Resources
What are some considerations for handling large volume data with Gin?
When dealing with large volume data in a Gin application, there are several considerations to keep in mind to ensure optimal performance and efficient resource utilization. Some of these considerations include:
1. Data storage: Choosing the right data storage solution is crucial when dealing with large volume data. Depending on the requirements of the application, options such as relational databases, NoSQL databases, distributed file systems, or data lakes may be suitable.
2. Data streaming: Streaming data can be an effective approach to handle large volume data in real-time. By processing data in small chunks or streams, it reduces the memory footprint and allows for continuous processing without overwhelming system resources.
3. Pagination: When retrieving large datasets from a database or API, pagination can help improve performance by fetching data in smaller chunks. This not only reduces the memory and processing requirements but also allows for faster response times and better user experience.
4. Bulk data operations: Performing operations on large datasets can be time-consuming and resource-intensive. Implementing bulk data operations, such as bulk inserts, updates, or deletions, can significantly improve the efficiency and speed of data processing.
5. Background processing and asynchronous tasks: Offloading time-consuming tasks to background processes or asynchronous tasks can help free up system resources and improve the responsiveness of the application. This is especially important when dealing with large volume data that requires time-consuming operations, such as data processing, transformations, or calculations.
6. Optimizing ORM configurations: If an Object-Relational Mapping (ORM) library is used in the Gin application, optimizing its configurations can help improve the performance and efficiency of data access operations. This includes tuning settings such as connection pooling, lazy loading, and caching to ensure optimal performance when working with large datasets.
Related Article: Intergrating Payment, Voice and Text with Gin & Golang
How can data streaming be implemented in a Gin application?
Data streaming is a technique that allows for the processing of large volume data in small, manageable chunks or streams. In a Gin application, data streaming can be implemented using the following steps:
1. Define a route handler: Create a route handler in the Gin application to handle the streaming request. This handler will be responsible for retrieving and processing the data in small chunks.
func streamData(c *gin.Context) { // Set the response headers to indicate that the response will be streamed c.Header("Content-Type", "application/octet-stream") c.Header("Transfer-Encoding", "chunked") // Retrieve the data in small chunks and stream it to the client for dataChunk := range getDataChunks() { c.Writer.Write(dataChunk) c.Writer.Flush() } }
2. Retrieve data in chunks: Implement a function, such as getDataChunks()
, that retrieves the data in small chunks from the data source. This function should use techniques like pagination or streaming APIs to fetch data in manageable portions.
func getDataChunks() <-chan []byte { dataChunks := make(chan []byte) go func() { // Retrieve data in chunks from the data source // Process the data and send it to the dataChunks channel for _, chunk := range fetchData() { dataChunks <- chunk } close(dataChunks) }() return dataChunks }
3. Stream data to the client: In the route handler, use the c.Writer
object to stream the data to the client. Write each data chunk to the writer and flush it to ensure that the data is sent immediately to the client.
4. Handle client-side processing: On the client-side, handle the streamed data using techniques like event-driven processing or chunked processing. This allows the client to process and display the data as it is received, rather than waiting for the entire dataset to be loaded.
What are some pagination techniques for handling large datasets in Gin?
Pagination is a common technique used to retrieve and display large datasets in smaller, manageable portions. In a Gin application, there are several pagination techniques that can be used to handle large datasets effectively:
1. Offset and limit pagination: This technique involves using the OFFSET
and LIMIT
clauses in the database query to retrieve a specific range of rows. The OFFSET
specifies the number of rows to skip, while the LIMIT
specifies the maximum number of rows to return.
func getPaginatedData(c *gin.Context) { // Retrieve the page number and items per page from the query parameters page := c.Query("page") perPage := c.Query("perPage") // Calculate the offset and limit based on the page number and items per page offset := (page - 1) * perPage limit := perPage // Query the database with the offset and limit result := db.Offset(offset).Limit(limit).Find(&data) // Return the paginated data as the API response c.JSON(http.StatusOK, result) }
2. Cursor-based pagination: Cursor-based pagination uses a cursor, typically an encoded string representing the last retrieved record, to determine the starting point for the next set of records. This technique ensures that the data is returned consistently and avoids issues like duplicate or missing records.
func getCursorPaginatedData(c *gin.Context) { // Retrieve the cursor from the query parameters cursor := c.Query("cursor") // Query the database with the cursor result := db.Where("id > ?", cursor).Limit(perPage).Find(&data) // Return the paginated data as the API response c.JSON(http.StatusOK, result) }
3. Keyset pagination: Keyset pagination relies on the ordering of a specific column or set of columns in the dataset. By using the values of the last retrieved record(s) in the ordering column(s) as the starting point, subsequent records can be retrieved efficiently. This technique is particularly useful when dealing with large datasets that are frequently updated.
func getKeysetPaginatedData(c *gin.Context) { // Retrieve the last retrieved record's keyset values from the query parameters lastKeyset1 := c.Query("lastKeyset1") lastKeyset2 := c.Query("lastKeyset2") // Query the database with the keyset values result := db.Where("keyset1 > ? OR (keyset1 = ? AND keyset2 > ?)", lastKeyset1, lastKeyset1, lastKeyset2).Limit(perPage).Find(&data) // Return the paginated data as the API response c.JSON(http.StatusOK, result) }
These pagination techniques provide flexibility in handling large datasets and allow for efficient retrieval and display of data in a Gin application.
How can bulk data operations be performed in a Gin application?
Performing bulk data operations, such as bulk inserts, updates, or deletions, can significantly improve the efficiency and speed of data processing in a Gin application. Here are some ways to perform bulk data operations:
1. Batch inserts: Instead of performing individual database inserts, bulk inserts can be used to insert multiple rows in a single database query. This reduces the overhead of executing multiple queries and improves the overall performance.
func bulkInsertData(c *gin.Context) { // Retrieve the data to be inserted from the request body var data []Data if err := c.BindJSON(&data); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } // Perform bulk insert using the database library db.Create(&data) c.JSON(http.StatusOK, gin.H{"message": "Bulk insert successful"}) }
2. Bulk updates: Instead of updating records individually, bulk updates can be used to update multiple records in a single database query. This reduces the number of round trips to the database and improves the performance.
func bulkUpdateData(c *gin.Context) { // Retrieve the updated data from the request body var data []Data if err := c.BindJSON(&data); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } // Perform bulk update using the database library db.Model(&Data{}).Updates(data) c.JSON(http.StatusOK, gin.H{"message": "Bulk update successful"}) }
3. Bulk deletions: Instead of deleting records individually, bulk deletions can be used to delete multiple records in a single database query. This reduces the overhead of executing multiple delete queries and improves the overall performance.
func bulkDeleteData(c *gin.Context) { // Retrieve the IDs of the records to be deleted from the request body var ids []int if err := c.BindJSON(&ids); err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": err.Error()}) return } // Perform bulk delete using the database library db.Where("id IN (?)", ids).Delete(&Data{}) c.JSON(http.StatusOK, gin.H{"message": "Bulk delete successful"}) }
Performing bulk data operations can significantly improve the efficiency and speed of data processing in a Gin application, especially when dealing with large datasets. It is important to ensure proper validation and error handling to maintain data integrity.
Related Article: Integrating Payment, Voice and Text with Beego & Golang
What is background processing and how is it useful in Gin applications?
Background processing refers to the execution of time-consuming or resource-intensive tasks in a separate process or thread, allowing the main application to continue running without being blocked. In a Gin application, background processing can be useful for handling tasks that require significant processing time, such as file uploads, image processing, email sending, or data processing.
There are several ways to implement background processing in a Gin application, including:
1. Goroutines: Goroutines are lightweight threads in Go that allow concurrent execution of functions. By launching a goroutine to handle time-consuming tasks, the main application can continue processing other requests without waiting for the task to complete.
func handleRequest(c *gin.Context) { // Start a goroutine to handle the time-consuming task go performBackgroundTask() // Continue processing the request or send a response to the client c.JSON(http.StatusOK, gin.H{"message": "Request received"}) } func performBackgroundTask() { // Perform the time-consuming task here }
2. Message queues: Message queues, such as RabbitMQ or Apache Kafka, can be used to decouple the Gin application from the background processing tasks. The Gin application can publish messages to a message queue, and a separate worker process can consume these messages and perform the tasks asynchronously.
func handleRequest(c *gin.Context) { // Publish a message to the message queue messageQueue.Publish("taskQueue", "taskData") // Continue processing the request or send a response to the client c.JSON(http.StatusOK, gin.H{"message": "Request received"}) } // Worker process func worker() { // Consume messages from the message queue for message := range messageQueue.Consume("taskQueue") { // Perform the time-consuming task here } }
3. Task scheduling: Task scheduling libraries, such as cron or Celery, can be used to schedule and execute time-consuming tasks at specific intervals or times. The Gin application can define the tasks and their schedules, and the task scheduler will ensure that they are executed in the background.
func main() { // Define a task schedule using a task scheduler library taskScheduler.Schedule("0 0 * * *", performBackgroundTask) // Start the Gin application router.Run(":8080") } func performBackgroundTask() { // Perform the time-consuming task here }
What are asynchronous tasks and how are they used in Gin?
Asynchronous tasks, also known as non-blocking tasks, are tasks that are executed independently of the main application flow. In a Gin application, asynchronous tasks can be used to handle time-consuming or resource-intensive operations without blocking the main application’s event loop.
In a Gin application, asynchronous tasks can be implemented using goroutines and channels. Here’s an example of how asynchronous tasks can be used in a Gin application:
func handleRequest(c *gin.Context) { // Start an asynchronous task go performAsyncTask(c) // Continue processing the request or send a response to the client c.JSON(http.StatusOK, gin.H{"message": "Request received"}) } func performAsyncTask(c *gin.Context) { // Perform the time-consuming or resource-intensive task asynchronously result := performTask() // Send the result to the main application using a channel resultChannel <- result } func main() { // Start a goroutine to listen for results from the asynchronous tasks go listenForResult() // Start the Gin application router.Run(":8080") } func listenForResult() { // Listen for results from the asynchronous tasks for result := range resultChannel { // Handle the result as needed fmt.Println(result) } }
In this example, when a request is received in the Gin application, an asynchronous task is started using a goroutine. The asynchronous task performs the time-consuming or resource-intensive operation and sends the result back to the main application using a channel. The main application continues processing other requests or operations and listens for results from the asynchronous tasks in a separate goroutine.
How can ORM configurations be optimized for handling large datasets in Gin?
When working with large datasets in a Gin application, optimizing ORM (Object-Relational Mapping) configurations can significantly improve performance and efficiency. Here are some techniques to optimize ORM configurations for handling large datasets:
1. Connection pooling: Connection pooling allows for reusing existing database connections instead of creating new connections for each request. By configuring an appropriate connection pool size and reusing connections, the overhead of creating and tearing down connections can be reduced, improving performance.
// Example configuration for connection pooling using GORM db, err := gorm.Open("postgres", "user=postgres password=secret dbname=mydb host=localhost port=5432 sslmode=disable") db.DB().SetMaxIdleConns(10) db.DB().SetMaxOpenConns(100)
2. Lazy loading: Lazy loading is a technique where related data is fetched only when accessed, rather than eagerly loading all the related data upfront. This can help reduce the amount of data fetched from the database and improve query performance.
// Example configuration for lazy loading using GORM type User struct { gorm.Model Name string Email string Orders []Order `gorm:"ForeignKey:UserID"` } type Order struct { gorm.Model UserID uint Amount float64 } // Load user data without fetching orders db.Preload("Orders", false).Find(&users)
3. Caching: Caching can be used to store frequently accessed data in memory, reducing the need to fetch data from the database. By configuring a caching layer, such as Redis or Memcached, ORM queries can be cached, resulting in faster response times and reduced database load.
// Example configuration for caching using GORM and Redis import "github.com/go-redis/redis" redisClient := redis.NewClient(&redis.Options{ Addr: "localhost:6379", Password: "", DB: 0, }) db, err := gorm.Open("postgres", "user=postgres password=secret dbname=mydb host=localhost port=5432 sslmode=disable") db.Use(redisCache.New(redisClient))
4. Batch processing: When working with large datasets, executing queries in batches can help reduce the overhead of individual queries. Batch processing involves grouping multiple records or operations into a single query, reducing the number of round trips to the database and improving performance.
// Example configuration for batch processing using GORM var users []User // Fetch users in batches of 100 db.Find(&users).Limit(100).Scan(&users)
5. Indexing: Proper indexing of database tables can significantly improve query performance, especially when working with large datasets. Analyze query patterns and create indexes on columns that are frequently used in WHERE clauses or JOIN operations.
// Example configuration for indexing using GORM type User struct { gorm.Model Name string `gorm:"index:idx_name"` Email string `gorm:"index:idx_email"` } // Create indexes on name and email columns db.AutoMigrate(&User{}) db.Model(&User{}).AddIndex("idx_name", "name") db.Model(&User{}).AddIndex("idx_email", "email")
Related Article: Integrating Beego & Golang Backends with React.js
What are some best practices for working with large datasets in Gin?
Working with large datasets in a Gin application requires careful consideration and adherence to best practices to ensure optimal performance and scalability. Here are some best practices for working with large datasets in Gin:
1. Use pagination: When retrieving large datasets from a database or API, implement pagination to fetch data in smaller chunks. This reduces the memory and processing requirements and allows for faster response times.
2. Optimize database queries: Analyze and optimize database queries to ensure they are efficient and performant. Use techniques like indexing, query optimization, and database profiling to improve query performance.
3. Implement caching: Use a caching layer, such as Redis or Memcached, to cache frequently accessed data. This reduces the need to fetch data from the database and improves response times.
4. Use asynchronous processing: Offload time-consuming or resource-intensive tasks to background processes or asynchronous tasks. This frees up system resources and improves the responsiveness of the application.
5. Optimize resource utilization: Monitor and optimize resource utilization, such as CPU, memory, and disk usage. Implement techniques like connection pooling, lazy loading, and batch processing to optimize resource usage.
6. Monitor and tune performance: Regularly monitor and analyze the performance of the Gin application using tools like profiling, monitoring, and logging. Identify bottlenecks and areas for improvement and fine-tune the application accordingly.
7. Implement data streaming: Use data streaming techniques to process large volume data in real-time. Streaming data reduces the memory footprint and allows for continuous processing without overwhelming system resources.
8. Scale horizontally: When dealing with extremely large datasets, consider scaling horizontally by distributing the workload across multiple servers or instances. Use load balancing techniques to distribute requests evenly and ensure high availability.
9. Implement fault tolerance: Ensure the Gin application is resilient to failures and can recover gracefully. Implement techniques like retry mechanisms, error handling, and fault tolerance patterns to handle failures in a robust manner.
10. Benchmark and test: Perform benchmarking and load testing to identify performance bottlenecks and validate the scalability of the Gin application. Use tools like Apache JMeter or Gatling to simulate high loads and measure performance metrics.
Additional Resources
– How does the Gin framework work?
– Golang Data Streaming with io.Pipe