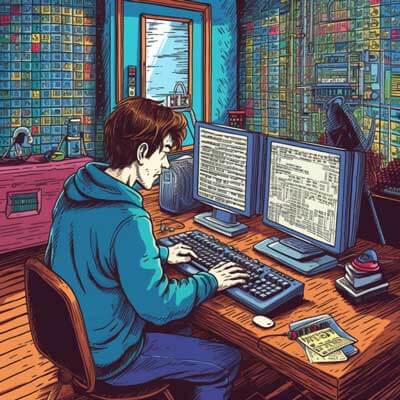
Table of Contents
How ReactJS State Works
ReactJS is a popular JavaScript library for building user interfaces. One of its key features is the concept of state, which allows components to store and manage data. In React, state is an object that holds the data that changes over time and affects the behavior and appearance of the component.
State in ReactJS is immutable, meaning it cannot be modified directly. Instead, you use the setState
method provided by React to update the state. When the state is updated, React re-renders the component to reflect the changes.
Here's an example of how to define and use state in a React component:
import React, { useState } from 'react';function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> );}
In this example, the useState
hook is used to create a state variable count
with an initial value of 0. The setCount
function is used to update the value of count
when the button is clicked. The value of count
is then displayed in the component.
Related Article: Enhancing React Applications with Third-Party Integrations
Handling State Persistence in ReactJS After Refresh
When a ReactJS component is refreshed, the state is typically lost. This is because React components are designed to be stateless, meaning they don't persist data between page reloads or component unmounts.
However, there are several strategies you can use to handle state persistence in ReactJS after a refresh:
1. Using browser storage: You can store the state data in browser storage, such as local storage or session storage, and retrieve it when the component is re-rendered. Here's an example:
import React, { useState, useEffect } from 'react';function Counter() { const [count, setCount] = useState(() => { const storedCount = localStorage.getItem('count'); return storedCount ? parseInt(storedCount) : 0; }); const increment = () => { setCount(count + 1); }; useEffect(() => { localStorage.setItem('count', count); }, [count]); return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> );}
In this example, the initial value of count
is retrieved from local storage using the getItem
method. The useEffect
hook is used to save the updated value of count
to local storage whenever it changes.
2. Using server-side persistence: If you need to persist state data across multiple users or devices, you can store the data on a server and retrieve it when the component is re-rendered. This can be done using APIs or databases. Here's an example using an API:
import React, { useState, useEffect } from 'react';function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; useEffect(() => { fetch('/api/count') .then(response => response.json()) .then(data => setCount(data.count)) .catch(error => console.error(error)); }, []); useEffect(() => { fetch('/api/count', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ count }), }) .then(response => response.json()) .catch(error => console.error(error)); }, [count]); return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> );}
In this example, the initial value of count
is fetched from the server using the fetch
API. The useEffect
hook is used to make a POST request to the server whenever count
changes, updating the server-side state.
What Happens When a ReactJS Component is Refreshed
When a ReactJS component is refreshed, the entire component tree is re-rendered. This means that all the child components of the refreshed component also get re-rendered, even if their props or state haven't changed.
Refreshing a ReactJS component can happen in several ways, such as manually refreshing the browser page or using the window.location.reload()
method in JavaScript. When the component is refreshed, the state of the component is lost, as React components are designed to be stateless.
However, React provides ways to handle state persistence and prevent data loss on refresh, as explained in the previous section.
Understanding ReactJS Lifecycle Methods
ReactJS provides a set of lifecycle methods that allow you to hook into different stages of a component's life cycle. These methods can be used to perform actions or side effects at specific points in the component's life cycle, such as when it is mounted, updated, or unmounted.
Here are the main lifecycle methods in ReactJS:
- componentDidMount
: This method is called after the component has been mounted (i.e., added to the DOM). It is commonly used to perform initialization tasks, such as fetching data from a server or setting up event listeners.
- componentDidUpdate
: This method is called whenever the component's props or state have changed and the component is re-rendered. It is commonly used to perform side effects based on the updated props or state.
- componentWillUnmount
: This method is called right before the component is unmounted (i.e., removed from the DOM). It is commonly used to clean up any resources or event listeners created in the componentDidMount
method.
React also provides other lifecycle methods, such as shouldComponentUpdate
and getSnapshotBeforeUpdate
, which give you more fine-grained control over the rendering and updating process of a component.
Here's an example that demonstrates the usage of lifecycle methods in a React component:
import React, { Component } from 'react';class Timer extends Component { constructor(props) { super(props); this.state = { seconds: 0 }; } componentDidMount() { this.intervalId = setInterval(() => { this.setState(prevState => ({ seconds: prevState.seconds + 1, })); }, 1000); } componentWillUnmount() { clearInterval(this.intervalId); } render() { return <p>Seconds: {this.state.seconds}</p>; }}
In this example, the componentDidMount
method is used to start a timer that increments the seconds
state every second. The componentWillUnmount
method is used to clear the interval when the component is unmounted, preventing memory leaks.
Related Article: How Component Interaction Works in ReactJS
How ReactJS Handles Rendering
ReactJS uses a virtual DOM (VDOM) to efficiently update and render components. The virtual DOM is a lightweight representation of the actual DOM and is used to determine the minimal set of changes required to update the real DOM.
When a component's state or props change, React reconciles the virtual DOM with the real DOM and updates only the necessary parts of the DOM. This process is known as diffing and is one of the key features of React that makes it highly performant.
React uses a diffing algorithm to compare the previous virtual DOM with the new virtual DOM and determine the differences. It then applies those differences to the real DOM, making the necessary updates.
Here's an example to illustrate how React handles rendering:
import React, { useState } from 'react';function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> );}
In this example, when the button is clicked, the count
state is updated using the setCount
function. React then reconciles the virtual DOM to determine the changes needed to update the real DOM. It updates only the parts of the DOM that have changed, in this case, the value of the Count
paragraph.
This efficient rendering process is one of the reasons why React is widely used for building complex user interfaces.
Exploring ReactJS Hooks
ReactJS Hooks are a feature introduced in React version 16.8 that allows you to use state and other React features in functional components, without the need for class components.
Hooks provide a way to reuse stateful logic between components and simplify the codebase. They also make it easier to write and test components.
Here are some commonly used React Hooks:
- useState
: This hook allows functional components to use state. It returns an array with two elements: the current state value and a function to update the state. Here's an example:
import React, { useState } from 'react';function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> );}
In this example, the useState
hook is used to create a state variable count
with an initial value of 0. The setCount
function is used to update the value of count
when the button is clicked.
- useEffect
: This hook allows functional components to perform side effects, such as fetching data from a server or subscribing to an event listener. It takes a callback function as an argument and runs it after every render. Here's an example:
import React, { useState, useEffect } from 'react';function Timer() { const [seconds, setSeconds] = useState(0); useEffect(() => { const intervalId = setInterval(() => { setSeconds(prevSeconds => prevSeconds + 1); }, 1000); return () => { clearInterval(intervalId); }; }, []); return <p>Seconds: {seconds}</p>;}
In this example, the useEffect
hook is used to start a timer that updates the seconds
state every second. The callback function also returns a cleanup function that clears the interval when the component is unmounted.
React Hooks provide a more concise and intuitive way to work with state and side effects in functional components, making React development more straightforward and efficient.
Updating State in ReactJS using setState
In ReactJS, state is updated using the setState
method, which is a built-in method provided by the React library. This method is used to update the state of a component and trigger a re-rendering of the component.
The setState
method can be called with an object that represents the updated state, or a callback function that receives the previous state as an argument and returns the updated state.
Here's an example that demonstrates how to update the state using setState
:
import React, { useState } from 'react';function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; const reset = () => { setCount(0); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> <button onClick={reset}>Reset</button> </div> );}
In this example, the increment
function updates the count
state by adding 1 to its current value using the setCount
function. The reset
function sets the count
state back to 0.
It's important to note that the setState
method is asynchronous, meaning that React may batch multiple state updates for performance reasons. To ensure that you have the most up-to-date state when updating based on the previous state, you can pass a callback function to setState
instead of an object.
import React, { useState } from 'react';function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(prevCount => prevCount + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> );}
In this example, the increment
function updates the count
state by incrementing the previous count value. This ensures that the update is based on the most recent state value.
Understanding ReactJS Context and its Usage
ReactJS Context is a feature that allows you to share data between components without explicitly passing it through props. It provides a way to pass data down the component tree without manually passing props at every level.
React Context consists of two parts: the context provider and the context consumer. The provider is responsible for creating a context and making the data available to its child components. The consumer is used to access the data provided by the context.
Here's an example that demonstrates the usage of React Context:
import React, { createContext, useContext } from 'react';const MyContext = createContext();function Parent() { return ( <MyContext.Provider value="Hello from Context"> <Child /> </MyContext.Provider> );}function Child() { const valueFromContext = useContext(MyContext); return <p>{valueFromContext}</p>;}function App() { return <Parent />;}
In this example, the MyContext
object is created using the createContext
function. The Parent
component wraps the Child
component with the MyContext.Provider
component and provides the value "Hello from Context" through the value
prop.
The Child
component uses the useContext
hook to access the value provided by the context. In this case, the value "Hello from Context" is displayed in the paragraph.
React Context is useful when you have data that needs to be accessed by multiple components at different levels in the component tree. It eliminates the need to pass props through intermediate components, making the code more readable and maintainable.
Related Article: How to Implement Custom CSS in ReactJS
Working with Props in ReactJS
In ReactJS, props are used to pass data from a parent component to its child components. Props are immutable and cannot be changed by the child components.
To pass props to a child component, you simply include the prop as an attribute when rendering the child component. The child component can then access the props using the props
object.
Here's an example that demonstrates how to work with props in ReactJS:
import React from 'react';function Greeting(props) { return <p>Hello, {props.name}!</p>;}function App() { return <Greeting name="John" />;}
In this example, the Greeting
component receives the name
prop and displays a greeting message using the prop value. The App
component renders the Greeting
component and passes the name
prop with the value "John".
Props can also include functions that can be used as callbacks in child components. Here's an example:
import React from 'react';function Button(props) { return <button onClick={props.onClick}>Click me</button>;}function App() { const handleClick = () => { console.log('Button clicked'); }; return <Button onClick={handleClick} />;}
In this example, the Button
component receives the onClick
prop, which is a function. When the button is clicked, the function specified in the onClick
prop is called.
Props allow you to pass data and behavior between components in a React application, making it easier to build reusable and modular components.
Understanding ReactJS Virtual DOM
The virtual DOM (VDOM) is a concept in ReactJS that represents a lightweight copy of the actual DOM. It is a JavaScript object that mirrors the structure of the real DOM and can be updated efficiently.
React uses the virtual DOM to determine the minimal set of changes required to update the real DOM when the state or props of a component change. This process is known as reconciliation or diffing.
When a component's state or props change, React creates a new virtual DOM tree and compares it with the previous virtual DOM tree. It identifies the differences between the two trees and updates only the necessary parts of the real DOM.
The virtual DOM allows React to perform updates in an efficient and optimized manner. Instead of directly manipulating the real DOM, which can be slow and resource-intensive, React calculates the minimal set of changes required and applies them to the real DOM.
Here's an example to illustrate how the virtual DOM works in React:
import React, { useState } from 'react';function Counter() { const [count, setCount] = useState(0); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> );}
In this example, when the button is clicked, the count
state is updated using the setCount
function. React creates a new virtual DOM tree and compares it with the previous virtual DOM tree. It identifies that only the value of the Count
paragraph needs to be updated and applies the necessary changes to the real DOM.
Preventing State Loss on Refresh in ReactJS
1. Using browser storage: You can store the state data in browser storage, such as local storage or session storage, and retrieve it when the component is re-rendered. This strategy was explained in detail in the "Handling State Persistence in ReactJS After Refresh" section.
2. Using server-side persistence: If you need to persist state data across multiple users or devices, you can store the data on a server and retrieve it when the component is re-rendered. This strategy was also explained in detail in the "Handling State Persistence in ReactJS After Refresh" section.
3. Using URL parameters: You can include the state data as URL parameters when the component is refreshed and retrieve it from the URL when the component is re-rendered. This can be done using libraries like react-router
or by manually parsing the URL parameters.
Here's an example that demonstrates how to use URL parameters to prevent state loss on refresh:
import React, { useState, useEffect } from 'react';import { useParams } from 'react-router-dom';function Counter() { const [count, setCount] = useState(0); const { countParam } = useParams(); useEffect(() => { if (countParam) { setCount(parseInt(countParam)); } }, [countParam]); const increment = () => { setCount(count + 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> );}
In this example, the useParams
hook from the react-router-dom
library is used to retrieve the countParam
from the URL. If the countParam
is present, it is used to set the initial value of count
. This allows the component to maintain its state even when refreshed.
These strategies provide different levels of state persistence depending on your specific requirements. Choose the strategy that best suits your application's needs to prevent state loss on refresh in ReactJS.