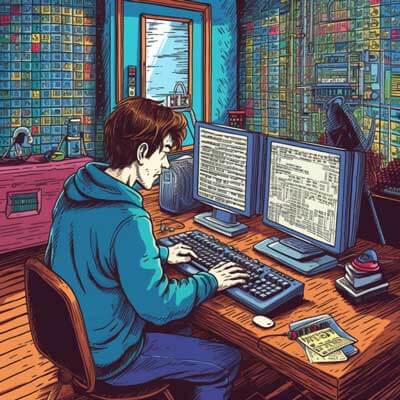
Table of Contents
Laravel WebSockets is a package that allows you to build real-time features in your Laravel applications using WebSockets. WebSockets provide a persistent connection between the client and the server, allowing for real-time communication. With Laravel WebSockets, you can easily implement features such as chat rooms, notifications, and real-time dashboards.
To get started with Laravel WebSockets, you'll need to install the package and configure it in your Laravel application. Here's a step-by-step guide on how to do that:
1. Install the Laravel WebSockets package using Composer:
composer require beyondcode/laravel-websockets
2. Publish the package's configuration file:
php artisan vendor:publish --provider="BeyondCode\LaravelWebSockets\WebSocketsServiceProvider" --tag="config"
3. Configure your broadcasting driver to use WebSockets. Open the config/broadcasting.php
file and set the default
value to pusher
:
'default' => env('BROADCAST_DRIVER', 'pusher'),
4. Update your .env
file with the necessary WebSocket configuration:
BROADCAST_DRIVER=pusher PUSHER_APP_ID=your-app-id PUSHER_APP_KEY=your-app-key PUSHER_APP_SECRET=your-app-secret PUSHER_APP_CLUSTER=your-app-cluster
5. Run the WebSocket server using Artisan:
php artisan websockets:serve
Now that you have Laravel WebSockets set up, you can start building real-time features in your Laravel application.
Example 1: Building a Real-time Chat Room
Let's say you want to add a real-time chat room feature to your Laravel application using Laravel WebSockets. Here's how you can do it:
1. Create a new channel for the chat room in the routes/channels.php
file:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('chat-room', function ($user) { return $user; });
2. Create an event class that will be broadcasted when a new message is sent in the chat room. You can generate the event class using the make:event
Artisan command:
php artisan make:event NewChatMessage
3. In the generated app/Events/NewChatMessage.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class NewChatMessage implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat-room'); } }
4. In your controller or wherever you handle sending messages, broadcast the NewChatMessage
event:
use App\Events\NewChatMessage; public function sendMessage(Request $request) { // Validate and store the message $message = $request->input('message'); // Broadcast the event event(new NewChatMessage($message)); return response()->json(['message' => 'Message sent.']); }
5. In your JavaScript code, listen for the NewChatMessage
event and update the chat room UI with the new message:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Update the chat room UI with the new message console.log(event.message); });
With these steps, you now have a real-time chat room feature in your Laravel application using Laravel WebSockets.
Related Article: How to Delete An Element From An Array In PHP
Example 2: Real-time Dashboard with Live Data Updates
Another common use case for real-time features is building a dashboard that displays live data updates. Here's how you can do it using Laravel WebSockets:
1. Create a new channel for the dashboard updates in the routes/channels.php
file:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('dashboard-updates', function ($user) { return $user; });
2. Create an event class that will be broadcasted when there's a data update. You can generate the event class using the make:event
Artisan command:
php artisan make:event DataUpdate
3. In the generated app/Events/DataUpdate.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class DataUpdate implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $data; public function __construct($data) { $this->data = $data; } public function broadcastOn() { return new Channel('dashboard-updates'); } }
4. In your code where you update the dashboard data, broadcast the DataUpdate
event:
use App\Events\DataUpdate; public function updateData() { // Update the data $data = ['value' => rand(1, 100)]; // Broadcast the event event(new DataUpdate($data)); return response()->json(['message' => 'Data updated.']); }
5. In your JavaScript code, listen for the DataUpdate
event and update the dashboard UI with the new data:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('dashboard-updates') .listen('DataUpdate', (event) => { // Update the dashboard UI with the new data console.log(event.data); });
With these steps, you now have a real-time dashboard that displays live data updates in your Laravel application using Laravel WebSockets.
Benefits of Using Laravel Echo for Building Chat Rooms, Notifications, and Real-time Dashboards
Laravel Echo is a JavaScript library that makes it easy to work with WebSockets and real-time features in your Laravel applications. It provides a simple and intuitive API for subscribing to channels, listening for events, and broadcasting events. Here are some benefits of using Laravel Echo for building chat rooms, notifications, and real-time dashboards:
1. Easy integration with Laravel WebSockets: Laravel Echo seamlessly integrates with Laravel WebSockets, making it easy to build real-time features in your Laravel applications.
2. Simplified event listening: Laravel Echo provides a clean and intuitive API for listening to events. You can easily subscribe to channels and listen for specific events, making it straightforward to handle real-time updates in your application.
3. Broadcasting events: Laravel Echo allows you to broadcast events from your Laravel application to the client-side JavaScript code. This makes it easy to send real-time notifications, updates, and messages to your users.
4. Real-time data synchronization: With Laravel Echo, you can keep your client-side data in sync with the server-side data in real-time. This is especially useful for chat rooms, where you want messages to appear instantly for all participants.
5. Scalability: Laravel Echo and Laravel WebSockets are built to handle high loads and scale with your application. They provide the infrastructure needed to handle thousands of concurrent connections and deliver real-time updates efficiently.
Example 1: Subscribing to a Chat Room Channel
To demonstrate the benefits of using Laravel Echo, let's see how you can subscribe to a chat room channel and listen for new messages:
1. Initialize Laravel Echo in your JavaScript code:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, });
2. Subscribe to the chat room channel and listen for new messages:
window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Update the chat room UI with the new message console.log(event.message); });
With Laravel Echo, subscribing to a chat room channel and listening for new messages is as simple as these two steps. Laravel Echo takes care of handling the WebSocket connection and delivering the events to your JavaScript code.
Related Article: How To Fix the “Array to string conversion” Error in PHP
Example 2: Broadcasting a Notification
Another benefit of using Laravel Echo is the ability to broadcast notifications to your users in real-time. Here's an example of how you can broadcast a notification using Laravel Echo:
1. In your Laravel application, define a notification class that extends the Illuminate\Notifications\Notification
class:
use Illuminate\Notifications\Notification; use Illuminate\Notifications\Messages\BroadcastMessage; class NewMessageNotification extends Notification { protected $message; public function __construct($message) { $this->message = $message; } public function via($notifiable) { return ['broadcast']; } public function toBroadcast($notifiable) { return new BroadcastMessage([ 'message' => $this->message, ]); } }
2. In your code where you want to send the notification, use the Notification
facade to send the notification:
use App\Notifications\NewMessageNotification; use Illuminate\Support\Facades\Notification; Notification::send($user, new NewMessageNotification('You have a new message.'));
3. In your JavaScript code, listen for the notification event and display the notification to the user:
window.Echo.private(`user.${userId}`) .notification((notification) => { // Display the notification to the user console.log(notification.message); });
With Laravel Echo, broadcasting a notification and handling it on the client-side is straightforward. You can easily send real-time notifications to your users and provide them with instant updates.
Optimizing Laravel for Long-polling and Other Real-time Strategies
While Laravel WebSockets and Laravel Echo provide a useful solution for implementing real-time features in your Laravel applications, there are other strategies you can use to optimize Laravel for real-time communication, such as long-polling. Long-polling is a technique where the client sends a request to the server and waits for a response until new data is available.
To optimize Laravel for long-polling and other real-time strategies, you can follow these steps:
1. Configure your Laravel application to use long-polling as the broadcasting driver. Open the config/broadcasting.php
file and set the default
value to longpolling
:
'default' => env('BROADCAST_DRIVER', 'longpolling'),
2. Update your .env
file with the necessary long-polling configuration:
BROADCAST_DRIVER=longpolling
3. Implement your long-polling logic in your Laravel application. This typically involves creating a route that waits for new data and returns a response when data is available.
Here's an example of how you can implement long-polling in Laravel:
use Illuminate\Http\Request; Route::get('/poll', function (Request $request) { $timeout = $request->input('timeout', 30); // Timeout in seconds $start = time(); $end = $start + $timeout; while (true) { // Check if new data is available if (/* new data is available */) { return response()->json(['data' => /* new data */]); } // Check if the timeout has been reached if (time() >= $end) { return response()->json(['timeout' => true]); } // Sleep for a short period of time usleep(500000); // Sleep for 0.5 seconds } });
With this implementation, the client can make a request to the /poll
route and wait for new data to be available. The server will keep the connection open until either new data is available or the timeout period is reached.
Example 1: Implementing Long-polling in Laravel
To demonstrate how to implement long-polling in Laravel, let's see an example where we implement a simple chat application using long-polling:
1. Create a new route for the long-polling endpoint in your routes/web.php
file:
use Illuminate\Http\Request; Route::get('/poll', function (Request $request) { $timeout = $request->input('timeout', 30); // Timeout in seconds $start = time(); $end = $start + $timeout; while (true) { // Check if new messages are available if (/* new messages are available */) { return response()->json(['messages' => /* new messages */]); } // Check if the timeout has been reached if (time() >= $end) { return response()->json(['timeout' => true]); } // Sleep for a short period of time usleep(500000); // Sleep for 0.5 seconds } });
2. In your JavaScript code, make a request to the long-polling endpoint and wait for new messages:
function poll() { fetch('/poll') .then(response => response.json()) .then(data => { if (data.messages) { // Display the new messages in the chat UI console.log(data.messages); } else if (data.timeout) { // Handle the timeout console.log('Timeout reached.'); } // Poll again poll(); }) .catch(error => { // Handle the error console.error(error); // Poll again poll(); }); } // Start polling poll();
With this implementation, the client will continuously poll the server for new messages and update the chat UI when new messages are available.
Example 2: Using Long-polling for Real-time Notifications
Another use case for long-polling is implementing real-time notifications in your Laravel application. Here's an example of how you can use long-polling to provide real-time notifications to your users:
1. Create a new route for the long-polling endpoint in your routes/web.php
file:
use Illuminate\Http\Request; Route::get('/poll', function (Request $request) { $timeout = $request->input('timeout', 30); // Timeout in seconds $start = time(); $end = $start + $timeout; while (true) { // Check if new notifications are available if (/* new notifications are available */) { return response()->json(['notifications' => /* new notifications */]); } // Check if the timeout has been reached if (time() >= $end) { return response()->json(['timeout' => true]); } // Sleep for a short period of time usleep(500000); // Sleep for 0.5 seconds } });
2. In your JavaScript code, make a request to the long-polling endpoint and wait for new notifications:
function poll() { fetch('/poll') .then(response => response.json()) .then(data => { if (data.notifications) { // Display the new notifications to the user console.log(data.notifications); } else if (data.timeout) { // Handle the timeout console.log('Timeout reached.'); } // Poll again poll(); }) .catch(error => { // Handle the error console.error(error); // Poll again poll(); }); } // Start polling poll();
With this implementation, the client will continuously poll the server for new notifications and display them to the user when they are available.
Related Article: How to Write to the Console in PHP
Understanding Pusher and Its Relation to Real-time Features in Laravel
Pusher is a hosted service that provides real-time messaging capabilities for web and mobile applications. It allows you to easily implement real-time features in your Laravel applications by providing a simple API for sending and receiving real-time events.
In the context of Laravel, Pusher is often used as the broadcasting driver for real-time features. Laravel provides built-in support for Pusher through the pusher
broadcasting driver. With Pusher, you can easily broadcast events from your Laravel application to the client-side JavaScript code, enabling real-time communication.
To use Pusher with Laravel, you'll need to sign up for a Pusher account and obtain your Pusher credentials. Here's how you can configure Laravel to use Pusher as the broadcasting driver:
1. Install the Pusher PHP library using Composer:
composer require pusher/pusher-php-server
2. Update your .env
file with the necessary Pusher credentials:
BROADCAST_DRIVER=pusher PUSHER_APP_ID=your-app-id PUSHER_APP_KEY=your-app-key PUSHER_APP_SECRET=your-app-secret PUSHER_APP_CLUSTER=your-app-cluster
3. Configure your broadcasting driver to use Pusher. Open the config/broadcasting.php
file and set the pusher
configuration options:
'pusher' => [ 'driver' => 'pusher', 'key' => env('PUSHER_APP_KEY'), 'secret' => env('PUSHER_APP_SECRET'), 'app_id' => env('PUSHER_APP_ID'), 'options' => [ 'cluster' => env('PUSHER_APP_CLUSTER'), 'encrypted' => true, ], ],
With Pusher configured as the broadcasting driver, you can now use Laravel's broadcasting features to send and receive real-time events.
Example 1: Broadcasting a Real-time Event with Pusher
To demonstrate how you can use Pusher to broadcast a real-time event from your Laravel application, let's see an example where we broadcast a new message event to a chat room channel:
1. Create a new channel for the chat room in the routes/channels.php
file:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('chat-room', function ($user) { return $user; });
2. Create an event class that will be broadcasted when a new message is sent in the chat room. You can generate the event class using the make:event
Artisan command:
php artisan make:event NewChatMessage
3. In the generated app/Events/NewChatMessage.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class NewChatMessage implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat-room'); } }
4. In your controller or wherever you handle sending messages, broadcast the NewChatMessage
event:
use App\Events\NewChatMessage; public function sendMessage(Request $request) { // Validate and store the message $message = $request->input('message'); // Broadcast the event event(new NewChatMessage($message)); return response()->json(['message' => 'Message sent.']); }
5. In your JavaScript code, listen for the NewChatMessage
event and update the chat room UI with the new message:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Update the chat room UI with the new message console.log(event.message); });
With these steps, you can use Pusher to broadcast a real-time event from your Laravel application and handle it on the client-side.
Example 2: Receiving Real-time Notifications with Pusher
Another use case for Pusher is receiving real-time notifications in your Laravel application. Here's an example of how you can use Pusher to receive real-time notifications:
1. In your Laravel application, define a notification class that extends the Illuminate\Notifications\Notification
class:
use Illuminate\Notifications\Notification; use Illuminate\Notifications\Messages\BroadcastMessage; class NewMessageNotification extends Notification { protected $message; public function __construct($message) { $this->message = $message; } public function via($notifiable) { return ['broadcast']; } public function toBroadcast($notifiable) { return new BroadcastMessage([ 'message' => $this->message, ]); } }
2. In your code where you want to send the notification, use the Notification
facade to send the notification:
use App\Notifications\NewMessageNotification; use Illuminate\Support\Facades\Notification; Notification::send($user, new NewMessageNotification('You have a new message.'));
3. In your JavaScript code, listen for the notification event and display the notification to the user:
window.Echo.private(`user.${userId}`) .notification((notification) => { // Display the notification to the user console.log(notification.message); });
With Pusher, you can easily send and receive real-time events in your Laravel application, enabling real-time communication and providing instant updates to your users.
Socket.IO and Its Usage in Laravel for Real-time Communication
Socket.IO is a JavaScript library that enables real-time, bidirectional communication between the client and the server. It provides a WebSocket-like API for building real-time applications, with features such as event-based communication, room support, and automatic reconnection.
In the context of Laravel, you can use Socket.IO to enhance the real-time communication capabilities of your Laravel applications. Laravel provides built-in support for Socket.IO through the laravel-echo-server
package, which is included with Laravel Echo.
To use Socket.IO with Laravel, you'll need to install the laravel-echo-server
package and configure it in your Laravel application. Here's how you can do that:
1. Install the laravel-echo-server
package globally using npm:
npm install -g laravel-echo-server
2. Generate a laravel-echo-server.json
configuration file in your Laravel application's root directory:
laravel-echo-server init
3. Configure the laravel-echo-server.json
file with your Socket.IO and Laravel Echo settings. Make sure to set the authHost
option to your Laravel application's URL:
{ "authHost": "http://your-app-url", "authEndpoint": "/broadcasting/auth", "clients": [ { "appId": "your-app-id", "key": "your-app-key" } ], "database": "redis", "databaseConfig": { "redis": {}, "sqlite": { "databasePath": "/database/laravel-echo-server.sqlite" } }, "devMode": true, "host": "your-host", "port": "your-port", "protocol": "http", "socketio": {}, "sslCertPath": "", "sslKeyPath": "" }
4. Start the laravel-echo-server
process:
laravel-echo-server start
With Socket.IO and Laravel Echo configured, you can now use Socket.IO to enhance the real-time communication capabilities of your Laravel applications.
Related Article: How to Fix the 404 Not Found Error in Errordocument in PHP
Example 1: Subscribing to a Socket.IO Channel
To demonstrate how you can use Socket.IO to subscribe to a channel and receive real-time updates in your Laravel application, let's see an example where we subscribe to a chat room channel:
1. Install the Socket.IO client library using npm:
npm install socket.io-client
2. In your JavaScript code, initialize Socket.IO and subscribe to the chat room channel:
import io from 'socket.io-client'; const socket = io('http://your-app-url'); socket.on('connect', () => { console.log('Connected to Socket.IO server.'); // Subscribe to the chat room channel socket.emit('subscribe', 'chat-room'); }); socket.on('new-message', (message) => { // Handle the new message console.log(message); }); socket.on('disconnect', () => { console.log('Disconnected from Socket.IO server.'); });
With this implementation, you can use Socket.IO to subscribe to a channel and receive real-time updates in your Laravel application.
Example 2: Broadcasting a Socket.IO Event
Another use case for Socket.IO is broadcasting events from your Laravel application to the client-side JavaScript code. Here's an example of how you can use Socket.IO to broadcast a new message event to a chat room channel:
1. In your Laravel application, create a new route for handling the Socket.IO events in your routes/web.php
file:
use Illuminate\Http\Request; use Illuminate\Support\Facades\Broadcast; Broadcast::routes(['middleware' => 'auth']); Route::post('/broadcasting/auth', function (Request $request) { return Broadcast::auth($request); });
2. In your Laravel application, define an event class that will be broadcasted when a new message is sent in the chat room. You can generate the event class using the make:event
Artisan command:
php artisan make:event NewChatMessage
3. In the generated app/Events/NewChatMessage.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class NewChatMessage implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat-room'); } }
4. In your controller or wherever you handle sending messages, broadcast the NewChatMessage
event:
use App\Events\NewChatMessage; public function sendMessage(Request $request) { // Validate and store the message $message = $request->input('message'); // Broadcast the event event(new NewChatMessage($message)); return response()->json(['message' => 'Message sent.']); }
With these steps, you can use Socket.IO to broadcast a real-time event from your Laravel application and handle it on the client-side.
PubSub and Enabling Real-time Data Updates in Laravel
PubSub (Publish-Subscribe) is a messaging pattern that allows for real-time data updates by enabling publishers to send messages to subscribers. In the context of Laravel, you can use PubSub to enable real-time data updates in your Laravel applications.
Laravel provides built-in support for PubSub through the Illuminate\Broadcasting\BroadcastManager
class. The BroadcastManager allows you to configure different broadcasting drivers, such as Pusher, Redis, and more. By using a PubSub driver, you can send real-time events to subscribers and keep their data in sync with the server-side data.
To enable real-time data updates in your Laravel application using PubSub, you'll need to configure a PubSub driver and implement the necessary event broadcasting logic. Here's how you can do that:
1. Choose a PubSub driver that suits your needs. Laravel supports various PubSub drivers out of the box, such as Pusher, Redis, and more. Configure the driver in your .env
file. For example, to use Pusher as the PubSub driver, add the following configuration options:
BROADCAST_DRIVER=pusher PUSHER_APP_ID=your-app-id PUSHER_APP_KEY=your-app-key PUSHER_APP_SECRET=your-app-secret PUSHER_APP_CLUSTER=your-app-cluster
2. Implement your event broadcasting logic. Laravel provides a simple and intuitive API for broadcasting events. You can use the broadcast
helper function to dispatch events that will be broadcasted to subscribers. For example, to broadcast a new message event to a chat room channel:
use App\Events\NewChatMessage; public function sendMessage(Request $request) { // Validate and store the message $message = $request->input('message'); // Broadcast the event broadcast(new NewChatMessage($message)); return response()->json(['message' => 'Message sent.']); }
3. Subscribe to the channel and listen for the events on the client-side. Laravel Echo provides a convenient way to subscribe to channels and listen for events. You can use Laravel Echo's API to handle real-time data updates in your JavaScript code. For example, to listen for new messages in a chat room:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Handle the new message console.log(event.message); });
With these steps, you can enable real-time data updates in your Laravel application using PubSub. By broadcasting events and subscribing to channels, you can keep the client-side data in sync with the server-side data and provide real-time updates to your users.
Example 1: Broadcasting a Real-time Event with PubSub
To demonstrate how you can use PubSub to broadcast a real-time event from your Laravel application, let's see an example where we broadcast a new message event to a chat room channel:
1. Create a new channel for the chat room in the routes/channels.php
file:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('chat-room', function ($user) { return $user; });
2. Create an event class that will be broadcasted when a new message is sent in the chat room. You can generate the event class using the make:event
Artisan command:
php artisan make:event NewChatMessage
3. In the generated app/Events/NewChatMessage.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class NewChatMessage implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat-room'); } }
4. In your controller or wherever you handle sending messages, broadcast the NewChatMessage
event:
use App\Events\NewChatMessage; public function sendMessage(Request $request) { // Validate and store the message $message = $request->input('message'); // Broadcast the event broadcast(new NewChatMessage($message)); return response()->json(['message' => 'Message sent.']); }
5. In your JavaScript code, listen for the NewChatMessage
event and update the chat room UI with the new message:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Update the chat room UI with the new message console.log(event.message); });
With these steps, you can use PubSub to broadcast a real-time event from your Laravel application and handle it on the client-side.
Related Article: How to Echo or Print an Array in PHP
Example 2: Real-time Data Updates with PubSub
Another use case for PubSub is enabling real-time data updates in your Laravel application. Here's an example of how you can use PubSub to keep client-side data in sync with the server-side data:
1. Create a new channel for the data updates in the routes/channels.php
file:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('data-updates', function ($user) { return $user; });
2. Create an event class that will be broadcasted when there's a data update. You can generate the event class using the make:event
Artisan command:
php artisan make:event DataUpdate
3. In the generated app/Events/DataUpdate.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class DataUpdate implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $data; public function __construct($data) { $this->data = $data; } public function broadcastOn() { return new Channel('data-updates'); } }
4. In your code where you update the data, broadcast the DataUpdate
event:
use App\Events\DataUpdate; public function updateData() { // Update the data $data = ['value' => rand(1, 100)]; // Broadcast the event broadcast(new DataUpdate($data)); return response()->json(['message' => 'Data updated.']); }
5. In your JavaScript code, listen for the DataUpdate
event and update the UI with the new data:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('data-updates') .listen('DataUpdate', (event) => { // Update the UI with the new data console.log(event.data); });
With these steps, you can use PubSub to enable real-time data updates in your Laravel application. By broadcasting events and subscribing to channels, you can keep the client-side data in sync with the server-side data and provide real-time updates to your users.
Event Broadcasting in Laravel for Real-time Features
Event broadcasting is a useful feature in Laravel that allows you to send real-time events to your application's clients. With event broadcasting, you can easily implement real-time features such as chat rooms, notifications, and live updates.
Laravel provides a simple and intuitive API for broadcasting events. You can use the broadcast
helper function to dispatch events that will be broadcasted to subscribers. Laravel supports various broadcasting drivers out of the box, such as Pusher, Redis, and more.
To use event broadcasting in your Laravel application, you'll need to configure a broadcasting driver and implement the necessary event broadcasting logic. Here's how you can do that:
1. Choose a broadcasting driver that suits your needs. Laravel supports various broadcasting drivers out of the box, such as Pusher, Redis, and more. Configure the driver in your .env
file. For example, to use Pusher as the broadcasting driver, add the following configuration options:
BROADCAST_DRIVER=pusher PUSHER_APP_ID=your-app-id PUSHER_APP_KEY=your-app-key PUSHER_APP_SECRET=your-app-secret PUSHER_APP_CLUSTER=your-app-cluster
2. Implement your event broadcasting logic. Laravel provides a simple and intuitive API for broadcasting events. You can use the broadcast
helper function to dispatch events that will be broadcasted to subscribers. For example, to broadcast a new message event to a chat room channel:
use App\Events\NewChatMessage; public function sendMessage(Request $request) { // Validate and store the message $message = $request->input('message'); // Broadcast the event broadcast(new NewChatMessage($message)); return response()->json(['message' => 'Message sent.']); }
3. Subscribe to the channel and listen for the events on the client-side. Laravel Echo provides a convenient way to subscribe to channels and listen for events. You can use Laravel Echo's API to handle real-time updates in your JavaScript code. For example, to listen for new messages in a chat room:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Handle the new message console.log(event.message); });
With these steps, you can use event broadcasting in your Laravel application to send and receive real-time events.
Example 1: Broadcasting a Real-time Event
To demonstrate how you can use event broadcasting to send real-time events from your Laravel application, let's see an example where we broadcast a new message event to a chat room channel:
1. Create a new channel for the chat room in the routes/channels.php
file:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('chat-room', function ($user) { return $user; });
2. Create an event class that will be broadcasted when a new message is sent in the chat room. You can generate the event class using the make:event
Artisan command:
php artisan make:event NewChatMessage
3. In the generated app/Events/NewChatMessage.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class NewChatMessage implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat-room'); } }
4. In your controller or wherever you handle sending messages, broadcast the NewChatMessage
event:
use App\Events\NewChatMessage; public function sendMessage(Request $request) { // Validate and store the message $message = $request->input('message'); // Broadcast the event broadcast(new NewChatMessage($message)); return response()->json(['message' => 'Message sent.']); }
5. In your JavaScript code, listen for the NewChatMessage
event and update the chat room UI with the new message:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Update the chat room UI with the new message console.log(event.message); });
With these steps, you can use event broadcasting to send real-time events from your Laravel application and handle them on the client-side.
Example 2: Real-time Notification Broadcasting
Another use case for event broadcasting is sending real-time notifications to your application's users. Here's an example of how you can use event broadcasting to send real-time notifications in your Laravel application:
1. In your Laravel application, define a notification class that extends the Illuminate\Notifications\Notification
class:
use Illuminate\Notifications\Notification; use Illuminate\Notifications\Messages\BroadcastMessage; class NewMessageNotification extends Notification { protected $message; public function __construct($message) { $this->message = $message; } public function via($notifiable) { return ['broadcast']; } public function toBroadcast($notifiable) { return new BroadcastMessage([ 'message' => $this->message, ]); } }
2. In your code where you want to send the notification, use the Notification
facade to send the notification:
use App\Notifications\NewMessageNotification; use Illuminate\Support\Facades\Notification; Notification::send($user, new NewMessageNotification('You have a new message.'));
3. In your JavaScript code, listen for the notification event and display the notification to the user:
window.Echo.private(`user.${userId}`) .notification((notification) => { // Display the notification to the user console.log(notification.message); });
With these steps, you can use event broadcasting to send real-time notifications to your application's users and handle them on the client-side.
Related Article: Useful PHP Code Snippets for Everyday Tasks
Practical Use Cases for Real-time Notifications in Laravel
Real-time notifications can greatly enhance the user experience of your Laravel applications. By providing instant updates and alerts, you can keep your users informed and engaged. Here are some practical use cases for real-time notifications in Laravel:
1. New message notifications: When a user receives a new message, you can send them a real-time notification to let them know about it. This can be particularly useful in chat applications or messaging systems.
2. Activity feed updates: If your application has an activity feed, you can send real-time notifications whenever there's new activity, such as new posts, comments, or likes. This allows your users to stay up-to-date with the latest content without needing to manually refresh the page.
3. Order status updates: If you have an e-commerce application, you can send real-time notifications to your users when their order status changes. This keeps your users informed about the progress of their orders, reducing the need for manual status checks.
4. System alerts and notifications: Real-time notifications can be used to send system alerts and notifications to your users. For example, you can notify them about scheduled maintenance, service disruptions, or important updates.
5. Real-time collaboration: If your application supports real-time collaboration, you can use notifications to inform users about changes made by other users in real-time. This is particularly useful in applications like document editors, project management tools, and collaborative design tools.
These are just a few examples of how real-time notifications can be used in Laravel applications. The possibilities are endless, and you can tailor the notifications to fit the specific needs of your application and users.
Example 1: Sending Real-time Notifications for New Messages
To demonstrate how you can send real-time notifications for new messages in your Laravel application, let's see an example where we send a notification to a user when they receive a new message:
1. In your Laravel application, define a notification class that extends the Illuminate\Notifications\Notification
class:
use Illuminate\Notifications\Notification; use Illuminate\Notifications\Messages\BroadcastMessage; class NewMessageNotification extends Notification { protected $message; public function __construct($message) { $this->message = $message; } public function via($notifiable) { return ['broadcast']; } public function toBroadcast($notifiable) { return new BroadcastMessage([ 'message' => $this->message, ]); } }
2. In your code where you want to send the notification, use the Notification
facade to send the notification:
use App\Notifications\NewMessageNotification; use Illuminate\Support\Facades\Notification; Notification::send($user, new NewMessageNotification('You have a new message.'));
3. In your JavaScript code, listen for the notification event and display the notification to the user:
window.Echo.private(`user.${userId}`) .notification((notification) => { // Display the notification to the user console.log(notification.message); });
With these steps, you can send real-time notifications to your users when they receive new messages in your Laravel application.
Example 2: Real-time Order Status Updates
Another practical use case for real-time notifications in Laravel is sending order status updates to your users. Here's an example of how you can send real-time notifications for order status updates:
1. In your Laravel application, define a notification class that extends the Illuminate\Notifications\Notification
class:
use Illuminate\Notifications\Notification; use Illuminate\Notifications\Messages\BroadcastMessage; class OrderStatusUpdateNotification extends Notification { protected $order; public function __construct($order) { $this->order = $order; } public function via($notifiable) { return ['broadcast']; } public function toBroadcast($notifiable) { return new BroadcastMessage([ 'order_id' => $this->order->id, 'status' => $this->order->status, ]); } }
2. In your code where you update the order status, send the notification to the user:
use App\Notifications\OrderStatusUpdateNotification; use Illuminate\Support\Facades\Notification; Notification::send($user, new OrderStatusUpdateNotification($order));
3. In your JavaScript code, listen for the notification event and update the UI with the new order status:
window.Echo.private(`user.${userId}`) .notification((notification) => { // Update the order status in the UI console.log(notification.order_id, notification.status); });
With these steps, you can send real-time notifications to your users when their order status changes in your Laravel application.
Utilizing Broadcast Channels for Real-time Communication in Laravel
Broadcast channels are a useful feature in Laravel that allow you to define fine-grained permissions and control over who can listen to your real-time events. By utilizing broadcast channels, you can ensure that only authorized users can receive certain events.
Laravel provides a simple and intuitive API for defining broadcast channels. You can use the Broadcast::channel
method to define the authorization logic for a channel. The closure passed to the Broadcast::channel
method receives the authenticated user as an argument and should return true
if the user is authorized to listen to the channel.
To utilize broadcast channels for real-time communication in your Laravel application, you'll need to define the channels and implement the necessary authorization logic. Here's how you can do that:
1. Define the broadcast channels in the routes/channels.php
file. For example, to define a channel for a chat room:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('chat-room', function ($user) { return $user; });
2. In your event classes, specify the broadcast channel using the broadcastOn
method. For example, to broadcast a new message event to the chat room channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class NewChatMessage implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat-room'); } }
3. In your JavaScript code, listen for the events on the broadcast channels. For example, to listen for new messages in the chat room channel:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Handle the new message console.log(event.message); });
With these steps, you can utilize broadcast channels for real-time communication in your Laravel application. By defining the channels and implementing the authorization logic, you can ensure that only authorized users can receive certain events.
Related Article: How to Use Loops in PHP
Example 1: Defining a Broadcast Channel for a Chat Room
To demonstrate how you can define a broadcast channel for a chat room in your Laravel application, let's see an example:
1. Define the broadcast channel in the routes/channels.php
file:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('chat-room', function ($user) { return $user; });
2. Create an event class that will be broadcasted when a new message is sent in the chat room. You can generate the event class using the make:event
Artisan command:
php artisan make:event NewChatMessage
3. In the generated app/Events/NewChatMessage.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class NewChatMessage implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat-room'); } }
4. In your controller or wherever you handle sending messages, broadcast the NewChatMessage
event:
use App\Events\NewChatMessage; public function sendMessage(Request $request) { // Validate and store the message $message = $request->input('message'); // Broadcast the event broadcast(new NewChatMessage($message)); return response()->json(['message' => 'Message sent.']); }
5. In your JavaScript code, listen for the NewChatMessage
event and update the chat room UI with the new message:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Update the chat room UI with the new message console.log(event.message); });
With these steps, you can define a broadcast channel for a chat room in your Laravel application and handle the new message events on the client-side.
Example 2: Defining a Broadcast Channel for Private Notifications
Another use case for broadcast channels is defining private channels for private notifications. Here's an example of how you can define a private broadcast channel for sending private notifications in your Laravel application:
1. Define the private broadcast channel in the routes/channels.php
file:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('private-notifications.{userId}', function ($user, $userId) { return $user->id === $userId; });
2. Create an event class that will be broadcasted as a private notification. You can generate the event class using the make:event
Artisan command:
php artisan make:event NewPrivateNotification
3. In the generated app/Events/NewPrivateNotification.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class NewPrivateNotification implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new PrivateChannel('private-notifications.' . $this->message->user_id); } }
4. In your code where you want to send the private notification, broadcast the NewPrivateNotification
event:
use App\Events\NewPrivateNotification; public function sendPrivateNotification(Request $request) { // Validate and store the notification $message = $request->input('message'); $userId = $request->input('user_id'); // Broadcast the event broadcast(new NewPrivateNotification($message))->toOthers(); return response()->json(['message' => 'Private notification sent.']); }
5. In your JavaScript code, listen for the private notification event and display the notification to the user:
window.Echo.private(`private-notifications.${userId}`) .listen('NewPrivateNotification', (event) => { // Display the private notification to the user console.log(event.message); });
With these steps, you can define a private broadcast channel for private notifications in your Laravel application and handle the private notification events on the client-side.
Exploring WebSockets Servers and Enabling Real-time Messaging in Laravel
WebSockets servers are responsible for establishing and maintaining the persistent connection between clients and servers in real-time applications. They enable bidirectional communication and facilitate real-time messaging between clients and servers.
Laravel WebSockets is a package that allows you to build real-time features in your Laravel applications using WebSockets. It provides a WebSocket server implementation that works seamlessly with Laravel and enables real-time messaging.
To explore WebSockets servers and enable real-time messaging in your Laravel application, you'll need to install and configure Laravel WebSockets. Here's how you can do that:
1. Install the Laravel WebSockets package using Composer:
composer require beyondcode/laravel-websockets
2. Publish the package's configuration file:
php artisan vendor:publish --provider="BeyondCode\LaravelWebSockets\WebSocketsServiceProvider" --tag="config"
3. Configure your broadcasting driver to use WebSockets. Open the config/broadcasting.php
file and set the default
value to pusher
:
'default' => env('BROADCAST_DRIVER', 'pusher'),
4. Update your .env
file with the necessary WebSocket configuration:
BROADCAST_DRIVER=pusher PUSHER_APP_ID=your-app-id PUSHER_APP_KEY=your-app-key PUSHER_APP_SECRET=your-app-secret PUSHER_APP_CLUSTER=your-app-cluster
5. Run the WebSocket server using Artisan:
php artisan websockets:serve
With the WebSocket server running, you can now enable real-time messaging in your Laravel application using WebSockets.
Example 1: Enabling Real-time Messaging with Laravel WebSockets
To demonstrate how you can enable real-time messaging in your Laravel application using Laravel WebSockets, let's see an example where we implement a real-time chat room feature:
1. Install the Laravel WebSockets package using Composer:
composer require beyondcode/laravel-websockets
2. Publish the package's configuration file:
php artisan vendor:publish --provider="BeyondCode\LaravelWebSockets\WebSocketsServiceProvider" --tag="config"
3. Configure your broadcasting driver to use WebSockets. Open the config/broadcasting.php
file and set the default
value to pusher
:
'default' => env('BROADCAST_DRIVER', 'pusher'),
4. Update your .env
file with the necessary WebSocket configuration:
BROADCAST_DRIVER=pusher PUSHER_APP_ID=your-app-id PUSHER_APP_KEY=your-app-key PUSHER_APP_SECRET=your-app-secret PUSHER_APP_CLUSTER=your-app-cluster
5. Run the WebSocket server using Artisan:
php artisan websockets:serve
With the WebSocket server running, you can now enable real-time messaging in your Laravel application.
Related Article: How To Get The Full URL In PHP
Example 2: Building a Real-time Chat Room with Laravel WebSockets
To demonstrate how you can build a real-time chat room feature in your Laravel application using Laravel WebSockets, let's see an example:
1. Create a new channel for the chat room in the routes/channels.php
file:
use Illuminate\Support\Facades\Broadcast; Broadcast::channel('chat-room', function ($user) { return $user; });
2. Create an event class that will be broadcasted when a new message is sent in the chat room. You can generate the event class using the make:event
Artisan command:
php artisan make:event NewChatMessage
3. In the generated app/Events/NewChatMessage.php
file, define the event properties and the broadcast channel:
use Illuminate\Broadcasting\Channel; use Illuminate\Broadcasting\InteractsWithSockets; use Illuminate\Broadcasting\PresenceChannel; use Illuminate\Broadcasting\PrivateChannel; use Illuminate\Contracts\Broadcasting\ShouldBroadcast; use Illuminate\Foundation\Events\Dispatchable; use Illuminate\Queue\SerializesModels; class NewChatMessage implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public function __construct($message) { $this->message = $message; } public function broadcastOn() { return new Channel('chat-room'); } }
4. In your controller or wherever you handle sending messages, broadcast the NewChatMessage
event:
use App\Events\NewChatMessage; public function sendMessage(Request $request) { // Validate and store the message $message = $request->input('message'); // Broadcast the event broadcast(new NewChatMessage($message)); return response()->json(['message' => 'Message sent.']); }
5. In your JavaScript code, listen for the NewChatMessage
event and update the chat room UI with the new message:
import Echo from 'laravel-echo'; window.Echo = new Echo({ broadcaster: 'pusher', key: process.env.MIX_PUSHER_APP_KEY, cluster: process.env.MIX_PUSHER_APP_CLUSTER, encrypted: true, }); window.Echo.channel('chat-room') .listen('NewChatMessage', (event) => { // Update the chat room UI with the new message console.log(event.message); });
With these steps, you can enable real-time messaging in your Laravel application using Laravel WebSockets and build a real-time chat room feature.
Additional Resources
- Building chat rooms and real-time dashboards with Laravel Echo