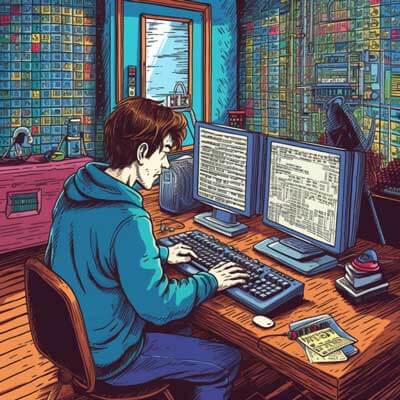
Table of Contents
Real-time features have become an integral part of modern web applications, enabling real-time communication, collaboration, and updates. One of the key technologies that powers real-time features is WebSockets. In this article, we will explore how WebSockets can be harnessed in Rails to build robust and scalable real-time applications.
What is the role of WebSockets in real-time features?
WebSockets provide a persistent connection between the client and the server, allowing bi-directional communication in real-time. Unlike traditional HTTP requests, which are stateless and require the client to initiate every communication, WebSockets enable the server to push updates to the client instantly. This makes WebSockets ideal for applications that require real-time updates, such as chat applications, collaboration tools, and live dashboards.
Related Article: Ruby on Rails with Bootstrap, Elasticsearch and Databases
How does Rails support real-time communication?
Rails, being a useful web application framework, provides an elegant solution for real-time communication through its built-in library called ActionCable. ActionCable is a WebSocket framework that is seamlessly integrated into Rails, allowing developers to build real-time features with ease.
To use ActionCable in Rails, you need to first configure it in your application. This involves setting up a Redis server for handling pub/sub functionality and configuring the ActionCable server to handle WebSocket connections. Once configured, you can define channels in your Rails application, which are responsible for handling real-time communication.
What is the difference between client-side and server-side implementation of real-time features?
Real-time features can be implemented both on the client-side and the server-side.
Client-side implementation involves using JavaScript frameworks or libraries, such as Socket.IO or Pusher, to establish a WebSocket connection with the server and handle real-time updates. The client-side code is responsible for listening to events from the server, handling incoming data, and updating the UI accordingly. This approach gives more control to the client and allows for a more interactive and responsive user experience.
Server-side implementation, on the other hand, involves using server-side technologies like ActionCable in Rails to handle WebSocket connections and manage real-time communication. With server-side implementation, the server takes care of handling WebSocket connections, managing channels, and broadcasting updates to clients. This approach simplifies the client-side code and offloads the real-time communication logic to the server.
What are the advantages of using WebSockets for real-time applications?
Using WebSockets for real-time applications offers several advantages over traditional HTTP requests:
1. Real-time updates: WebSockets allow for instant updates to be pushed from the server to the client, eliminating the need for the client to continuously poll the server for changes. This results in a more efficient and responsive user experience.
2. Bi-directional communication: WebSockets enable bi-directional communication, allowing both the client and the server to send data at any time. This opens up new possibilities for interactive and collaborative features in web applications.
3. Lower latency: Unlike HTTP requests, which incur the overhead of establishing a new connection for every request, WebSockets provide a persistent connection that reduces latency and improves performance.
4. Reduced network traffic: With WebSockets, only the necessary data is sent over the network, resulting in reduced network traffic compared to traditional HTTP requests that often include unnecessary headers and metadata.
5. Scalability: WebSockets are designed to handle a large number of concurrent connections, making them suitable for building scalable real-time applications.
Related Article: Ruby on Rails with Modular Rails, ActiveRecord & Testing
How does event-driven architecture work in real-time web applications?
Event-driven architecture plays a crucial role in real-time web applications. In an event-driven architecture, the application is built around events and event handlers. When an event occurs, such as a new message in a chat application, the server triggers the corresponding event handler, which can then update the necessary clients.
In Rails, ActionCable provides a pub/sub system based on Redis, where channels act as event handlers. Each channel can subscribe to specific events and broadcast updates to the subscribed clients. This event-driven approach allows for decoupled and scalable real-time communication.
What is the role of JavaScript in real-time features?
JavaScript plays a vital role in real-time features, particularly in client-side implementations. With JavaScript, developers can establish WebSocket connections, listen to events from the server, handle incoming data, and update the UI in real-time.
Here's an example of how JavaScript can be used to establish a WebSocket connection and handle real-time updates:
const socket = new WebSocket('ws://localhost:3000/cable'); socket.onopen = function() { // Connection established console.log('WebSocket connection opened'); }; socket.onmessage = function(event) { // Handle incoming data const data = JSON.parse(event.data); console.log('Received data:', data); // Update the UI with the received data }; socket.onclose = function() { // Connection closed console.log('WebSocket connection closed'); };
In this example, we create a new WebSocket object and specify the URL of the WebSocket server. We then define event handlers for the onopen
, onmessage
, and onclose
events to handle the WebSocket connection lifecycle and incoming data.
What is the purpose of Pub/Sub pattern in real-time applications?
The Publish/Subscribe (Pub/Sub) pattern is a messaging pattern that is commonly used in real-time applications. In the context of Rails and ActionCable, the Pub/Sub pattern allows channels to subscribe to specific events and broadcast updates to the subscribed clients.
Here's an example of how the Pub/Sub pattern is used in ActionCable:
class ChatChannel < ApplicationCable::Channel def subscribed # Subscribe to the chat channel stream_from 'chat_channel' end def receive(data) # Handle incoming data ActionCable.server.broadcast('chat_channel', data) end end
In this example, we define a ChatChannel
that extends ApplicationCable::Channel
. In the subscribed
method, we call stream_from('chat_channel')
to subscribe to the 'chat_channel'. This means that any updates broadcasted to the 'chat_channel' will be received by this channel. In the receive
method, we handle incoming data and then use ActionCable.server.broadcast
to broadcast the data to all subscribers of the 'chat_channel'.
How can push notifications be implemented in Rails for real-time updates?
Push notifications can be implemented in Rails using ActionCable and the Pub/Sub pattern. With push notifications, the server can send updates to the client even when the client is not actively interacting with the application.
To implement push notifications in Rails, you can define a channel that handles the delivery of push notifications. When a client subscribes to the channel, it will start receiving push notifications whenever there are updates.
Here's an example of how push notifications can be implemented in Rails:
class NotificationsChannel < ApplicationCable::Channel def subscribed # Subscribe to the notifications channel stream_from "notifications_#{current_user.id}" end def send_notification(data) # Send a push notification to the current user ActionCable.server.broadcast("notifications_#{current_user.id}", data) end end
In this example, we define a NotificationsChannel
that extends ApplicationCable::Channel
. In the subscribed
method, we call stream_from("notifications_#{current_user.id}")
to subscribe to the notifications channel specific to the current user. This ensures that each user only receives their own notifications. In the send_notification
method, we handle sending push notifications to the current user by using ActionCable.server.broadcast
with the appropriate channel.
Related Article: Ruby on Rails Performance Tuning and Optimization
What are some scalability considerations when using ActionCable in Rails?
When building real-time applications with ActionCable in Rails, there are several scalability considerations to keep in mind:
1. Connection management: ActionCable maintains a connection for each WebSocket client, which can consume server resources. It's important to properly manage and scale the number of connections to ensure optimal performance.
2. Channel concurrency: Each channel in ActionCable is capable of handling multiple subscribers simultaneously. However, if a channel becomes a bottleneck due to high traffic or processing requirements, it may be necessary to scale horizontally by adding more instances of the application or using load balancing techniques.
3. Redis scalability: ActionCable relies on Redis for its pub/sub functionality. As the number of WebSocket connections and channels increases, the load on the Redis server may also increase. Scaling the Redis server by adding more nodes or using Redis cluster can help alleviate scalability issues.
4. Database optimization: Real-time applications often require frequent updates to the database. Optimizing database queries, using caching techniques, and scaling the database infrastructure can improve the overall scalability of the application.
How can performance be optimized in ActionCable for real-time chat and collaboration tools?
To optimize performance in ActionCable for real-time chat and collaboration tools, consider the following techniques:
1. Minimize data transfer: Only send the necessary data over the WebSocket connection. Avoid sending large payloads or unnecessary metadata to reduce network traffic and improve performance.
2. Batch updates: Instead of sending individual updates for each event, batch them together to reduce the number of WebSocket messages. This can significantly improve performance, especially when there are frequent updates.
3. Use streaming instead of polling: Instead of continuously polling the server for updates, use streaming techniques to receive updates in real-time. This reduces the number of requests and improves the responsiveness of the application.
4. Optimize database queries: Real-time applications often require frequent database queries. Make sure to optimize database queries by using indexes, caching, and efficient query patterns to minimize the impact on performance.
5. Use caching: Cache frequently accessed data to reduce the load on the server and improve performance. This can be especially useful for chat and collaboration tools where certain data, such as user profiles or chat history, can be cached.
6. Horizontal scaling: Consider scaling the application horizontally by adding more instances or using load balancing techniques. This can distribute the load across multiple servers and improve the overall performance and scalability of the application.
Additional Resources
- Official Rails Guide on Action Cable