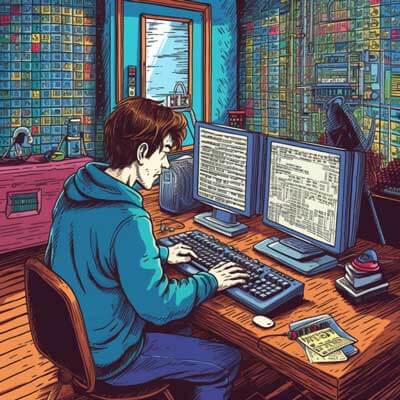
Table of Contents
How can I add asynchronous programming to my FastAPI loop?
FastAPI is built on top of the asynchronous programming concepts in Python. It utilizes the asyncio library, which allows you to write asynchronous code that can run concurrently. By leveraging asynchronous programming, you can improve the performance and responsiveness of your FastAPI application.
To add asynchronous programming to your FastAPI loop, you need to define and use async functions. These functions can be marked with the async
keyword and can contain await
statements, which delegate the execution of certain tasks to the event loop.
Example 1: Defining an async function
from fastapi import FastAPI app = FastAPI() async def async_function(): # Your asynchronous code here await asyncio.sleep(1) return "Async function completed" @app.get("/async") async def async_endpoint(): result = await async_function() return {"message": result}
In the above example, we define an asynchronous function async_function
that uses the await
statement to delegate the execution of asyncio.sleep(1)
to the event loop. We then use this async function in an API endpoint async_endpoint
by using await
to wait for the result of async_function
.
Example 2: Running multiple async functions concurrently
from fastapi import FastAPI import asyncio app = FastAPI() async def async_function1(): await asyncio.sleep(1) return "Async function 1 completed" async def async_function2(): await asyncio.sleep(2) return "Async function 2 completed" @app.get("/async") async def async_endpoint(): results = await asyncio.gather(async_function1(), async_function2()) return {"message1": results[0], "message2": results[1]}
In this example, we define two async functions async_function1
and async_function2
. We use the asyncio.gather
function to run these functions concurrently and wait for their results. The results are then returned in the API endpoint.
Related Article: FastAPI Enterprise Basics: SSO, RBAC, and Auditing
What is an event loop and how does it work in FastAPI?
An event loop is a central component of asynchronous programming in FastAPI. It is responsible for managing and executing asynchronous tasks. The event loop schedules tasks, runs them concurrently, and manages the flow of execution.
In FastAPI, the event loop is provided by the underlying asyncio library. It is created automatically when the FastAPI application starts and runs in the background. FastAPI uses the event loop to handle incoming requests and execute any asynchronous code within the application.
The event loop in FastAPI works by running tasks concurrently and switching between them when they need to wait for I/O operations or other tasks to complete. This allows for efficient utilization of system resources and ensures that the application remains responsive even when handling multiple requests simultaneously.
Example: Running an async function in the event loop
from fastapi import FastAPI import asyncio app = FastAPI() async def async_function(): await asyncio.sleep(1) return "Async function completed" @app.get("/async") async def async_endpoint(): loop = asyncio.get_event_loop() result = await loop.create_task(async_function()) return {"message": result}
In this example, we manually get the event loop using asyncio.get_event_loop()
. We then create a task in the event loop using loop.create_task()
and await its result. This allows us to run the asynchronous function async_function
in the event loop.
How do I create and use async functions in FastAPI?
Creating and using async functions in FastAPI is similar to regular functions, with the addition of the async
keyword. Async functions allow you to write code that can be executed concurrently and provides a way to work with asynchronous operations.
To create an async function in FastAPI, simply prefix the function definition with the async
keyword. Inside the async function, you can use the await
keyword to delegate the execution of certain tasks to the event loop.
Example 1: Creating and using an async function
from fastapi import FastAPI app = FastAPI() async def async_function(): # Your asynchronous code here await asyncio.sleep(1) return "Async function completed" @app.get("/async") async def async_endpoint(): result = await async_function() return {"message": result}
In this example, we define an async function async_function
that uses the await
statement to delegate the execution of asyncio.sleep(1)
to the event loop. We then use this async function in an API endpoint async_endpoint
by using await
to wait for the result of async_function
.
Example 2: Using multiple async functions concurrently
from fastapi import FastAPI import asyncio app = FastAPI() async def async_function1(): await asyncio.sleep(1) return "Async function 1 completed" async def async_function2(): await asyncio.sleep(2) return "Async function 2 completed" @app.get("/async") async def async_endpoint(): results = await asyncio.gather(async_function1(), async_function2()) return {"message1": results[0], "message2": results[1]}
In this example, we define two async functions async_function1
and async_function2
. We use the asyncio.gather
function to run these functions concurrently and wait for their results. The results are then returned in the API endpoint.
What are decorators and how can they be used in FastAPI?
Decorators are a useful feature in Python that allow you to modify the behavior of functions or classes by wrapping them with another function. They provide a way to add additional functionality to existing code without modifying its original implementation.
In FastAPI, decorators are commonly used to define API endpoints and apply additional functionality such as input validation, authentication, and error handling. FastAPI provides several built-in decorators that make it easy to define and customize API endpoints.
Example 1: Defining a basic API endpoint decorator
from fastapi import FastAPI app = FastAPI() def my_decorator(func): def wrapper(*args, **kwargs): # Add additional functionality here return func(*args, **kwargs) return wrapper @app.get("/hello") @my_decorator async def hello_endpoint(): return {"message": "Hello, World!"}
In this example, we define a simple decorator my_decorator
that wraps the hello_endpoint
function. The wrapper
function inside the decorator can add additional functionality before or after the execution of the wrapped function. In this case, it simply calls the wrapped function and returns its result.
Example 2: Using a decorator to add authentication to an API endpoint
from fastapi import FastAPI, Depends from fastapi.security import OAuth2PasswordBearer app = FastAPI() oauth2_scheme = OAuth2PasswordBearer(tokenUrl="token") def authenticate(func): def wrapper(*args, **kwargs): token = oauth2_scheme() # Perform authentication logic here return func(*args, **kwargs) return wrapper @app.get("/protected") @authenticate async def protected_endpoint(): return {"message": "This is a protected endpoint"}
In this example, we define a decorator authenticate
that uses the OAuth2PasswordBearer
class from FastAPI's fastapi.security
module to handle authentication. The decorator retrieves the access token from the request using oauth2_scheme()
and performs the necessary authentication logic before calling the wrapped function.
Related Article: Tutorial: i18n in FastAPI with Pydantic & Handling Encoding
What is middleware and how is it used in FastAPI?
Middleware is a concept in FastAPI that allows you to add additional functionality to your application's request-response cycle. It provides a way to intercept requests and responses, modify them, and apply common functionality such as authentication, logging, error handling, and more.
In FastAPI, middleware is implemented as a chain of functions that are executed sequentially for each request. Each middleware function can modify the request, perform some processing, and optionally modify the response before passing it to the next middleware in the chain.
Example: Creating a simple middleware in FastAPI
from fastapi import FastAPI, Request app = FastAPI() async def simple_middleware(request: Request, call_next): # Do something before the request is processed # For example, you can log the request details print(f"Received request to {request.url.path}") response = await call_next(request) # Do something after the response is processed # For example, you can add headers to the response response.headers["X-My-Header"] = "Hello, World!" return response app.middleware("http")(simple_middleware) @app.get("/hello") async def hello_endpoint(): return {"message": "Hello, World!"}
In this example, we define a simple middleware function simple_middleware
that logs the incoming request URL and adds a custom header to the response. We register this middleware using the app.middleware
method, passing the name of the middleware and the function itself. The middleware is applied to all routes defined in the FastAPI application.
How do I define an API endpoint in FastAPI?
Defining an API endpoint in FastAPI is straightforward and follows a similar pattern to other web frameworks. You need to create an instance of the FastAPI
class, define a route using one of the HTTP methods, and provide a function that will handle the request and return a response.
Example: Defining a basic API endpoint
from fastapi import FastAPI app = FastAPI() @app.get("/hello") async def hello_endpoint(): return {"message": "Hello, World!"}
In this example, we define a basic API endpoint using the app.get
decorator provided by FastAPI. The decorator specifies the HTTP method GET
and the route /hello
. The function hello_endpoint
is called when a GET request is made to the /hello
route. It returns a JSON response with a simple greeting message.
How does FastAPI handle incoming requests?
FastAPI handles incoming requests by matching the requested URL path and HTTP method to the defined API endpoints. When a request is received, FastAPI checks each registered route to find a match. If a match is found, the corresponding function is called to handle the request and generate a response.
FastAPI uses a highly efficient routing system that leverages the capabilities of modern Python features like type annotations and function annotations. This allows FastAPI to perform request validation and data conversion automatically, resulting in faster and more reliable request handling.
Example: Handling a POST request in FastAPI
from fastapi import FastAPI app = FastAPI() @app.post("/users") async def create_user(user: User): # Create user logic here return {"message": "User created successfully"}
In this example, we define a route /users
that handles POST
requests using the app.post
decorator. The function create_user
is called when a POST
request is made to the /users
route. It expects a JSON payload in the request body, which is automatically converted to a User
object based on the type annotation. FastAPI handles the request validation and data conversion automatically, allowing us to focus on the business logic.
What is routing and how is it implemented in FastAPI?
Routing is a fundamental concept in web development that determines how an application responds to different URLs. It allows you to map URLs to specific functions or handlers that are responsible for generating the appropriate response.
In FastAPI, routing is implemented using decorators provided by the FastAPI
class. These decorators define routes for different HTTP methods and associate them with corresponding functions. FastAPI's routing system is highly efficient and provides advanced features like automatic request validation and data conversion.
Example: Defining routes with different HTTP methods in FastAPI
from fastapi import FastAPI app = FastAPI() @app.get("/hello") async def hello_endpoint(): return {"message": "Hello, World!"} @app.post("/users") async def create_user(user: User): # Create user logic here return {"message": "User created successfully"}
In this example, we define two routes using the app.get
and app.post
decorators provided by FastAPI. The hello_endpoint
function is associated with the /hello
route and is called when a GET
request is made to that route. The create_user
function is associated with the /users
route and is called when a POST
request is made to that route. FastAPI automatically handles the request validation and data conversion based on the function annotations.
Related Article: Optimizing FastAPI Applications: Modular Design, Logging, and Testing
What is dependency injection and how is it used in FastAPI?
Dependency injection is a design pattern that allows you to provide dependencies to an object instead of the object creating or managing them itself. It promotes loose coupling between components and improves code reusability, maintainability, and testability.
In FastAPI, dependency injection is used extensively to provide dependencies to API endpoints and other components. FastAPI's dependency injection system is based on Python type hints and function annotations, making it easy to define and use dependencies.
Example: Using dependency injection in FastAPI
from fastapi import FastAPI, Depends app = FastAPI() def get_db(): # Connect to the database here return db @app.get("/users") async def list_users(db = Depends(get_db)): # Use the database connection here return {"message": "List of users"} @app.post("/users") async def create_user(user: User, db = Depends(get_db)): # Use the database connection here return {"message": "User created successfully"}
In this example, we define a function get_db
that returns a database connection. We use the Depends
class provided by FastAPI to declare the dependency on the get_db
function in the list_users
and create_user
functions. FastAPI automatically resolves the dependency and passes the result to the functions when they are called.
Additional Resources
- FastAPI - Official Documentation