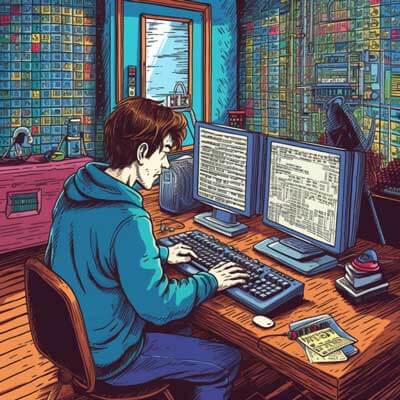
Table of Contents
To add days to a date in JavaScript, you can use the built-in Date
object and its methods. This can be useful in various scenarios, such as calculating future or past dates, scheduling events, or performing date arithmetic. In this answer, we will explore two possible approaches to add days to a date in JavaScript.
Approach 1: Using the setDate Method
One way to add days to a date in JavaScript is by using the setDate
method of the Date
object. This method allows you to set the day of the month for a given date. Here's an example:
// Create a new Date object var date = new Date(); // Add 5 days to the current date date.setDate(date.getDate() + 5); // Output the updated date console.log(date);
In this example, we first create a new Date
object using the Date
constructor, which initializes it with the current date and time. Then, we use the setDate
method to add 5 days to the current date by accessing the day of the month using getDate()
and adding 5 to it. Finally, we log the updated date object to the console.
This approach is straightforward and works well for simple date manipulation. However, it has some limitations. For example, if the resulting date is in a different month or year, the setDate
method will automatically adjust it accordingly. While this behavior might be desirable in some cases, it can lead to unexpected results when adding a large number of days or when dealing with complex date calculations.
Related Article: How to Retrieve Values From Get Parameters in Javascript
Approach 2: Using the getTime and setTime Methods
Another approach to add days to a date in JavaScript is by using the getTime
and setTime
methods of the Date
object. This approach allows for more flexible date manipulation and avoids the limitations mentioned in the previous approach. Here's an example:
// Create a new Date object var date = new Date(); // Get the current timestamp var timestamp = date.getTime(); // Add 5 days in milliseconds var newTimestamp = timestamp + (5 * 24 * 60 * 60 * 1000); // Set the updated timestamp date.setTime(newTimestamp); // Output the updated date console.log(date);
In this example, we first create a new Date
object as before. Then, we use the getTime
method to get the current timestamp of the date object. The timestamp represents the number of milliseconds since January 1, 1970. Next, we add 5 days in milliseconds to the current timestamp by multiplying the number of days by the number of milliseconds in a day (24 hours * 60 minutes * 60 seconds * 1000 milliseconds). After obtaining the updated timestamp, we set it using the setTime
method of the Date
object. Finally, we log the updated date object to the console.
This approach allows you to perform more complex date calculations, such as adding or subtracting any number of days, hours, minutes, or seconds to a date. It also handles different months and years correctly. However, it requires manual conversion between milliseconds and other time units, which can be error-prone and less intuitive than using the setDate
method.
Why Was the Question Asked?
The question "How to add days to date in JavaScript?" is commonly asked by developers who need to perform date manipulation in their JavaScript applications. Dates are a fundamental aspect of many applications, and being able to add or subtract days from a given date is a common requirement. For example, an e-commerce website might need to calculate the estimated delivery date of a package based on the current date and the shipping duration. A task management application might need to calculate the due date of a task based on its start date and duration. By understanding how to add days to a date in JavaScript, developers can implement such functionality effectively.
Potential Reasons for Asking the Question
There can be several potential reasons why someone might ask the question "How to add days to date in JavaScript?". Some of these reasons include:
1. Date Calculations: The person may need to perform date calculations in their JavaScript application, such as adding or subtracting days, weeks, months, or years to a given date.
2. Scheduling and Planning: The person may be working on a scheduling or planning application that requires the ability to calculate future or past dates based on user input or predefined rules.
3. Countdowns and Timers: The person may be building a countdown or timer feature where they need to calculate the end date and time based on the current date and a specified duration.
Related Article: How to Check for String Equality in Javascript
Suggestions and Alternative Ideas
When adding days to a date in JavaScript, it's important to keep in mind the specific requirements of your application and the behavior you expect. Here are some suggestions and alternative ideas to consider:
1. Consider Using a Date Library: JavaScript's built-in Date
object provides basic functionality for date manipulation. However, if you need more advanced features or a simpler API, you might consider using a popular date library like Moment.js or Luxon. These libraries provide a wide range of functions and utilities for working with dates and times.
2. Avoid Mutating the Original Date Object: In both of the approaches mentioned earlier, the original date object is modified to reflect the updated date. While this can be acceptable in some cases, it's generally recommended to avoid mutating objects whenever possible. Instead, you can create a new date object with the updated value and use it in your application.
3. Consider Time Zones: When working with dates and times, it's important to consider time zones. JavaScript's Date
object operates in the local time zone of the user's browser. If your application needs to handle different time zones, you might need to use a library or additional techniques to manage time zone conversions.