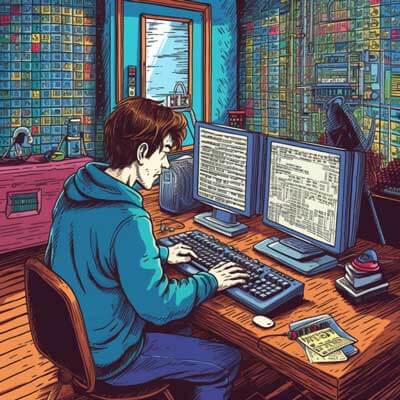
Table of Contents
To append one string to another in Python, you can use the concatenation operator (+) or the join() method. These methods allow you to combine strings together to create a new string that includes both the original strings.
Using the Concatenation Operator (+)
The concatenation operator (+) in Python allows you to concatenate two or more strings together. You can use it to append one string to another by simply adding the two strings using the operator.
Here's an example:
string1 = "Hello" string2 = "World" result = string1 + string2 print(result)
Output:
HelloWorld
In the above example, the concatenation operator (+) is used to append the string "World" to the end of the string "Hello", resulting in the new string "HelloWorld".
Related Article: How To Get Row Count Of Pandas Dataframe
Using the join() Method
The join() method in Python is another way to append one string to another. It takes an iterable (such as a list or a tuple) of strings as its argument and returns a new string that is the concatenation of all the strings in the iterable, with the specified string as the separator.
Here's an example:
string1 = "Hello" string2 = "World" separator = " " result = separator.join([string1, string2]) print(result)
Output:
Hello World
In the above example, the join() method is used to append the string "World" to the end of the string "Hello" with a space separator, resulting in the new string "Hello World".
Best Practices
When appending one string to another in Python, there are a few best practices to keep in mind:
1. Use the concatenation operator (+) when you only need to append a small number of strings together. It is more concise and easier to read.
2. Use the join() method when you need to append a large number of strings together or when you have an iterable of strings that you want to concatenate.
3. If you are appending multiple strings together, consider using f-strings or str.format() to format the strings before concatenating them. This can make your code more readable and maintainable.
4. Avoid using the += operator to append strings together in a loop, especially if you are concatenating a large number of strings. This is because strings in Python are immutable, so each time you use the += operator, a new string object is created, which can be inefficient.
Alternative Ideas
In addition to the concatenation operator (+) and the join() method, there are other ways to append one string to another in Python. Here are a few alternative ideas:
1. Using formatted string literals (f-strings):
string1 = "Hello" string2 = "World" result = f"{string1} {string2}" print(result)
2. Using string interpolation with the str.format() method:
string1 = "Hello" string2 = "World" result = "{} {}".format(string1, string2) print(result)
3. Using the += operator (not recommended for large strings or in a loop):
string1 = "Hello" string2 = "World" string1 += string2 print(string1)