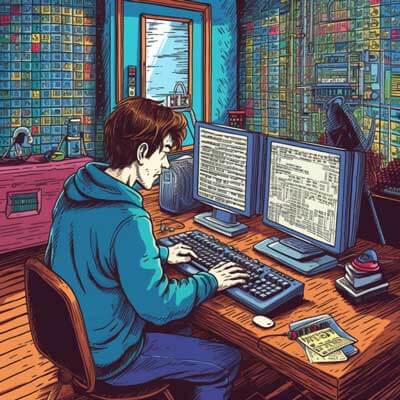
Table of Contents
Overview of Python Dictionaries
Python dictionaries are a useful data structure that allow you to store and retrieve data in a flexible and efficient way. Unlike other data structures in Python, such as lists or tuples, dictionaries do not store elements in a specific order. Instead, they use a key-value pair system, where each element in the dictionary is associated with a unique key.
Dictionaries are defined using curly braces ({}) and key-value pairs are separated by colons (:). Here's an example of a dictionary:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'}
In this example, the keys are 'name', 'age', and 'city', and the corresponding values are 'John', 25, and 'New York'.
Dictionaries are mutable, which means you can modify their contents. This makes them a versatile choice for storing and manipulating data in Python.
Related Article: How To Convert A Tensor To Numpy Array In Tensorflow
Key-Value Pairs in Dictionaries
In dictionaries, each key must be unique. If you try to assign a new value to an existing key, the old value will be overwritten. However, different keys can have the same value. For example:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York', 'country': 'USA'}
In this case, the keys 'city' and 'country' have different names but the same value, 'New York'. This is perfectly valid in dictionaries.
Exploring Mutable Properties of Dictionaries
One of the most useful aspects of dictionaries in Python is their mutability. This means that you can modify the contents of a dictionary after it has been created.
For example, you can add new key-value pairs to a dictionary, update the values of existing keys, or even remove key-value pairs altogether.
Let's explore some of the common dictionary methods that allow you to perform these operations.
Common Python Dictionary Methods
Python provides several built-in methods for working with dictionaries. Here are some of the most commonly used methods:
- keys()
: Returns a list of all the keys in the dictionary.
- values()
: Returns a list of all the values in the dictionary.
- items()
: Returns a list of all the key-value pairs in the dictionary.
- get(key)
: Returns the value associated with the specified key. If the key does not exist, it returns None.
- pop(key)
: Removes the key-value pair associated with the specified key from the dictionary and returns the value.
- clear()
: Removes all the key-value pairs from the dictionary.
These methods provide a convenient way to access and manipulate the contents of a dictionary.
Related Article: How To Convert a Dictionary To JSON In Python
Essential Dictionary Operations
Before we dive into the process of appending to a dictionary, let's take a look at some essential operations that you can perform on dictionaries.
To access the value associated with a specific key, you can use square brackets ([]). For example:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'}print(my_dict['name']) # Output: John
To update the value of an existing key, you can simply assign a new value to it. For example:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'}my_dict['age'] = 30print(my_dict) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
To remove a key-value pair from a dictionary, you can use the del
keyword. For example:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'}del my_dict['age']print(my_dict) # Output: {'name': 'John', 'city': 'New York'}
These basic operations are the building blocks for more advanced dictionary manipulations.
Adding a New Key-Value Pair to a Dictionary
To add a new key-value pair to a dictionary, you can simply assign a value to a new key. If the key already exists, the value will be updated.
Here's an example:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'}my_dict['country'] = 'USA'print(my_dict) # Output: {'name': 'John', 'age': 25, 'city': 'New York', 'country': 'USA'}
In this example, we added a new key-value pair ('country': 'USA') to the existing dictionary.
You can also use the update()
method to add multiple key-value pairs to a dictionary. The update()
method takes another dictionary as an argument and adds its key-value pairs to the original dictionary.
Here's an example:
my_dict = {'name': 'John', 'age': 25, 'city': 'New York'}new_data = {'country': 'USA', 'occupation': 'Engineer'}my_dict.update(new_data)print(my_dict) # Output: {'name': 'John', 'age': 25, 'city': 'New York', 'country': 'USA', 'occupation': 'Engineer'}
In this example, we used the update()
method to add two new key-value pairs ('country': 'USA' and 'occupation': 'Engineer') to the original dictionary.
Difference Between Updating and Appending a Dictionary
It's important to understand the difference between updating and appending a dictionary.
When you update a dictionary, you modify its contents by adding or updating existing key-value pairs. This means that if a key already exists, the value will be updated; otherwise, a new key-value pair will be added.
On the other hand, when you append one dictionary to another, you are merging the contents of both dictionaries into a single dictionary. This means that if a key already exists in the original dictionary, the value from the second dictionary will overwrite the existing value.
Let's see an example to illustrate the difference:
dict1 = {'name': 'John', 'age': 25}dict2 = {'age': 30, 'city': 'New York'}dict1.update(dict2)print(dict1) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, the value of the 'age' key is updated from 25 to 30, and the 'city' key is added to the original dictionary.
Now let's append one dictionary to another:
dict1 = {'name': 'John', 'age': 25}dict2 = {'age': 30, 'city': 'New York'}dict1.update(dict2)print(dict1) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, the value of the 'age' key is updated from 25 to 30, and the 'city' key is added to the original dictionary.
Appending One Dictionary to Another
To append one dictionary to another, you can use the update()
method, as shown in the previous example.
However, if you want to keep the original dictionaries intact and create a new dictionary that contains the merged contents, you can use the following approach:
dict1 = {'name': 'John', 'age': 25}dict2 = {'age': 30, 'city': 'New York'}merged_dict = {<strong>dict1, </strong>dict2}print(merged_dict) # Output: {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, we used the double asterisk (**) operator to merge the contents of dict1
and dict2
into a new dictionary called merged_dict
. The resulting dictionary contains all the key-value pairs from both dictionaries.
This approach allows you to keep the original dictionaries unchanged while creating a new dictionary that combines their contents.
Related Article: How to Use the Python map() Function
Additional Resources
- Python Dictionary - update() Method