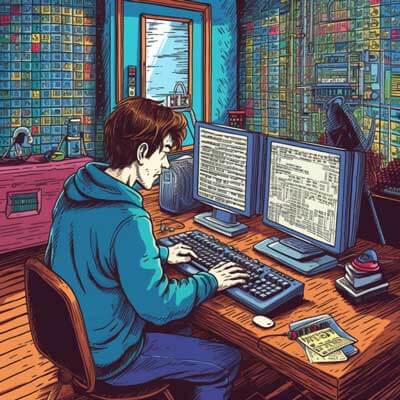
Table of Contents
Introduction to Building Forms
Building forms in React is a fundamental skill for any React developer. Forms are an essential part of most web applications, allowing users to input data and interact with the application. In this chapter, we will explore the basics of building forms in React and understand how to create reusable form components.
To get started, we need to set up a basic React project. Assuming you have Node.js installed, you can create a new React project using Create React App by running the following command in your terminal:
npx create-react-app my-form-app
Once the project is set up, navigate to the project directory and start the development server:
cd my-form-appnpm start
Now that we have our development environment ready, let's proceed to the next chapter to understand the different form components available in React.
Related Article: How to Render a File from a Config in ReactJS
Form Components Overview
React provides a variety of form components that can be used to build forms efficiently. In this chapter, we will explore some of the most commonly used form components and understand their purpose.
1. Input Elements: The most basic form component is the input element, which allows users to enter text, numbers, or make selections. React provides several input components such as input
, textarea
, and select
that can be used to create different types of input fields.
Example of an input element in React:
import React from 'react';function MyForm() { return ( <form> <label htmlFor="name">Name:</label> <input type="text" id="name" name="name" /> </form> );}
2. Checkbox and Radio Buttons: Checkbox and radio buttons are commonly used for multiple-choice options. React provides the input
component with type="checkbox"
and type="radio"
to create these input fields.
Example of a checkbox in React:
import React, { useState } from 'react';function MyForm() { const [isChecked, setIsChecked] = useState(false); const handleCheckboxChange = () => { setIsChecked(!isChecked); }; return ( <form> <label htmlFor="checkbox">Agree to terms:</label> <input type="checkbox" id="checkbox" name="checkbox" checked={isChecked} onChange={handleCheckboxChange} /> </form> );}
3. Dropdown Menus: Dropdown menus, also known as select elements, allow users to choose an option from a list. React provides the select
component along with the option
component to create dropdown menus.
Example of a dropdown menu in React:
import React, { useState } from 'react';function MyForm() { const [selectedOption, setSelectedOption] = useState(''); const handleSelectChange = (event) => { setSelectedOption(event.target.value); }; return ( <form> <label htmlFor="dropdown">Choose an option:</label> <select id="dropdown" name="dropdown" value={selectedOption} onChange={handleSelectChange}> <option value="">Select an option</option> <option value="option1">Option 1</option> <option value="option2">Option 2</option> <option value="option3">Option 3</option> </select> </form> );}
These are just a few examples of the form components available in React. In the next chapter, we will dive deeper into building a basic form and explore different input elements in more detail.
Designing A Basic Form
In this chapter, we will design a basic form using React components. We will create a form with input fields for name, email, and a submit button.
Here's the code snippet for the basic form:
import React, { useState } from 'react';function MyForm() { const [formData, setFormData] = useState({ name: '', email: '', }); const handleInputChange = (event) => { const { name, value } = event.target; setFormData({ ...formData, [name]: value }); }; const handleSubmit = (event) => { event.preventDefault(); // Perform form submission logic here }; return ( <form onSubmit={handleSubmit}> <label htmlFor="name">Name:</label> <input type="text" id="name" name="name" value={formData.name} onChange={handleInputChange} /> <label htmlFor="email">Email:</label> <input type="email" id="email" name="email" value={formData.email} onChange={handleInputChange} /> <button type="submit">Submit</button> </form> );}
In the code snippet above, we use the useState
hook to manage the form data. The handleInputChange
function updates the form data state whenever an input field value changes. The handleSubmit
function is called when the form is submitted, allowing you to perform any necessary form submission logic.
Next, we will explore how to work with input elements in more detail.
Working with Input Elements
Input elements are the most commonly used form components, allowing users to enter text, numbers, or make selections. In this chapter, we will explore different types of input elements in React and understand how to work with them effectively.
Text Input: A text input element is used to capture single-line text input from the user. React provides theinput
component with type="text"
to create a text input field.
Example of a text input element in React:
import React, { useState } from 'react';function MyForm() { const [name, setName] = useState(''); const handleInputChange = (event) => { setName(event.target.value); }; return ( <form> <label htmlFor="name">Name:</label> <input type="text" id="name" name="name" value={name} onChange={handleInputChange} /> </form> );}Email Input: An email input element is used to capture email addresses. React provides the
input
component with type="email"
to create an email input field.
Example of an email input element in React:
import React, { useState } from 'react';function MyForm() { const [email, setEmail] = useState(''); const handleInputChange = (event) => { setEmail(event.target.value); }; return ( <form> <label htmlFor="email">Email:</label> <input type="email" id="email" name="email" value={email} onChange={handleInputChange} /> </form> );}Number Input: A number input element is used to capture numeric input from the user. React provides the
input
component with type="number"
to create a number input field.
Example of a number input element in React:
import React, { useState } from 'react';function MyForm() { const [age, setAge] = useState(0); const handleInputChange = (event) => { setAge(event.target.value); }; return ( <form> <label htmlFor="age">Age:</label> <input type="number" id="age" name="age" value={age} onChange={handleInputChange} /> </form> );}
These are just a few examples of working with input elements in React. In the next chapter, we will explore how to handle form submission and process the form data.