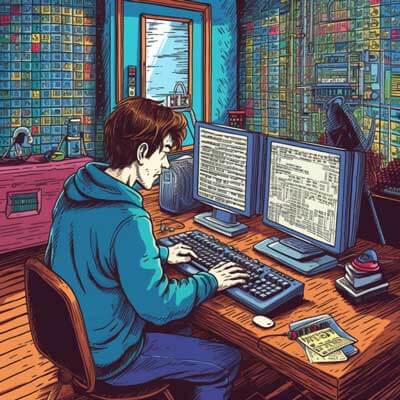
Table of Contents
Overview
Bypassing a router with a proxy allows developers to route requests through a different server during development. This can be useful for testing APIs without needing to deploy to a live server. A proxy acts as an intermediary, forwarding requests from a client to a server and returning the server's response to the client. This setup can help in scenarios where developers want to avoid cross-origin resource sharing (CORS) issues, streamline API interactions, or simulate a production environment.
Related Article: How to Fix Webpack Command Not Found Error
Setting Up devServer for Proxy Configuration
The Webpack development server (devServer
) provides a way to set up a local server for your application. To configure it to use a proxy, you'll need to modify the Webpack configuration file.
Example of a basic Webpack configuration with a devServer proxy:
// webpack.config.js module.exports = { devServer: { proxy: { '/api': { target: 'http://localhost:5000', changeOrigin: true, }, }, }, };
In this code, any request to /api
will be proxied to http://localhost:5000
. The changeOrigin
option is often set to true to ensure the host header of the request matches the target URL.
Configuring Proxy Settings in Development Environment
To configure proxy settings properly, the proxy definition should include paths that your application will request. Developers can define multiple paths and their respective targets.
Example:
// webpack.config.js module.exports = { devServer: { proxy: { '/api': { target: 'http://localhost:5000', changeOrigin: true, }, '/auth': { target: 'http://localhost:4000', changeOrigin: true, }, }, }, };
In this setup, any requests to /api
will go to port 5000 and /auth
will go to port 4000.
Addressing CORS Issues
CORS is a security feature that restricts web pages from making requests to a different domain than the one that served the web page. When a proxy is set up, it can help to bypass CORS restrictions because the request is made from the same origin as the web application.
Using the proxy configuration in Webpack, requests made from the browser to your application are forwarded to the specified target server. This means that the browser sees the requests as coming from the same origin, thus avoiding CORS issues.
For example, if your frontend is running on http://localhost:3000
, and your API is on http://localhost:5000
, setting up the proxy as shown earlier allows the frontend to communicate with the API seamlessly.
Related Article: How to Configure SVGR with Webpack
Modifying HTTP Headers in Proxy Requests
Sometimes, it is necessary to modify the HTTP headers of a request before it is sent to the target server. This can be done using a custom function in the proxy configuration.
Example:
// webpack.config.js const { createProxyMiddleware } = require('http-proxy-middleware'); module.exports = { devServer: { before: function(app) { app.use('/api', createProxyMiddleware({ target: 'http://localhost:5000', changeOrigin: true, onProxyReq: function(proxyReq, req, res) { proxyReq.setHeader('X-Special-Header', 'foobar'); }, })); }, }, };
In this example, an additional header X-Special-Header
is added to the request when proxied to the target server.
Implementing Path Rewriting
Path rewriting is useful when the API structure differs from what the client expects. Developers can modify the request path before it is sent to the target.
Example:
// webpack.config.js module.exports = { devServer: { proxy: { '/old-api': { target: 'http://localhost:5000', pathRewrite: { '^/old-api': '/new-api' }, changeOrigin: true, }, }, }, };
In this instance, requests to /old-api
will be rewritten to /new-api
when sent to the target server at port 5000.
Using API Gateway
An API gateway can simplify routing requests to multiple services. By integrating an API gateway into the development setup, developers can easily manage multiple endpoints.
For instance, if using an API gateway like Kong or AWS API Gateway, it can be configured to handle different services on different paths. When configuring Webpack's devServer
, the proxy can be set to point to the API gateway instead of individual services.
Example:
// webpack.config.js module.exports = { devServer: { proxy: { '/api': { target: 'http://api-gateway.example.com', changeOrigin: true, }, }, }, };
In this setup, the API gateway will route the requests to the appropriate backend services based on the incoming request paths.
Exploring Useful Plugins
Plugins enhance the functionality of the Webpack dev server. The http-proxy-middleware
is a widely used plugin that provides additional features such as logging and error handling.
To install this plugin, run:
npm install http-proxy-middleware --save-dev
Then, import and configure it in your Webpack config:
// webpack.config.js const { createProxyMiddleware } = require('http-proxy-middleware'); module.exports = { devServer: { before: function(app) { app.use('/api', createProxyMiddleware({ target: 'http://localhost:5000', changeOrigin: true, logLevel: 'debug', })); }, }, };
The logLevel
option can help debug issues by providing detailed logs of the proxy activity.
Related Article: How to Configure Electron with Webpack
Handling Network Requests through Proxy
When network requests are made from the client, they can be routed through the proxy. This allows developers to test how the application behaves when interacting with various APIs.
In a React application, for example, a fetch request can be made like so:
fetch('/api/data') .then(response => response.json()) .then(data => console.log(data));
With the proxy set up, this request will be forwarded to the target server, and the response will be returned as if it originated from the same domain.
Improving Local Development
Proxying requests during local development can greatly improve the workflow. Instead of constantly deploying code to test API interactions, developers can work on the frontend and backend simultaneously.
The setup allows for quick iterations and testing without the need for complicated configurations. This leads to faster feedback loops, enabling more effective debugging and feature development.
Creating a Mock Server for Testing
Mock servers can be created to simulate API responses during development. This can be helpful when the backend is not yet ready or when testing edge cases.
Using tools like json-server
, developers can easily set up a mock server:
npm install -g json-server json-server --watch db.json
Then, the proxy can be configured to point to the mock server:
// webpack.config.js module.exports = { devServer: { proxy: { '/api': { target: 'http://localhost:3000', // json-server port changeOrigin: true, }, }, }, };
In this setup, any requests to /api
will be responded to by the mock server running on port 3000.
Managing Cross-Origin Requests with Proxy
Cross-origin requests can be managed effectively by setting up a proxy. By routing requests through the same origin as the frontend, CORS issues are minimized or eliminated.
When a frontend application attempts to fetch resources from a different origin, the browser checks the CORS policy. If the server does not permit the request, it will be blocked. However, with the proxy, requests to the API are seen as coming from the same origin, thus bypassing these restrictions.
The proxy configuration in Webpack ensures that all requests to the defined paths are handled appropriately.
Related Article: How to Use Webpack CopyPlugin for Asset Management
Setting Up Proxy in Development Tools
Development tools often provide options for setting up proxies. For instance, tools like Postman allow users to configure proxies for testing API requests.
In Postman, navigate to the settings and enable the option to use a proxy. This allows requests made in Postman to be routed through the specified proxy settings, ensuring a similar experience as in the browser.
Configuring Multiple Proxies in Development
Multiple proxies can be configured in a single Webpack setup. This is useful when interacting with different APIs or services that require distinct endpoints.
Example:
// webpack.config.js module.exports = { devServer: { proxy: { '/api': { target: 'http://localhost:5000', changeOrigin: true, }, '/auth': { target: 'http://localhost:4000', changeOrigin: true, }, '/uploads': { target: 'http://localhost:3000', changeOrigin: true, }, }, }, };
This configuration allows the application to handle requests to three different services, enabling a flexible development environment.
Identifying Common Proxy Issues
Common issues when working with proxies include:
1. Incorrect target URL: Ensure the target server is running and accessible.
2. CORS errors: Confirm that the proxy is correctly set up to handle CORS.
3. Path not found: Verify that the path matches what the backend service expects.
4. Proxy server not responding: Check the network settings and server status.
Debugging these issues involves reviewing the network requests in the browser's developer tools and checking the console output for errors.
How Path Rewriting Functions in Proxy
Path rewriting modifies the request URL before it is sent to the target server. This is important when the structure of the API requires different endpoints than what the client is using.
The pathRewrite
option in the proxy configuration allows developers to specify rules for altering the request path. For instance, if an API endpoint has changed, developers can easily adjust the proxy configuration without needing to modify the client code.
Example:
// webpack.config.js module.exports = { devServer: { proxy: { '/api/v1': { target: 'http://localhost:5000', pathRewrite: { '^/api/v1': '/api/v2' }, changeOrigin: true, }, }, }, };
This setup rewrites requests from /api/v1
to /api/v2
, ensuring the client can still function without changes.
Related Article: How to Use the Clean Webpack Plugin
Comparing Proxy and Mock Server Use Cases
Proxies and mock servers serve different purposes in development. Proxies are used to route requests to live servers, allowing for real-time interactions with APIs. Mock servers, on the other hand, simulate API responses without needing a backend.
Use cases for proxies include:
- Testing integrations with live services.
- Handling CORS issues during development.
- Simulating a production environment.
Use cases for mock servers include:
- Testing application behavior without a live backend.
- Developing frontend features independently from backend availability.
- Simulating various API responses for edge case testing.
Testing API Routes
Testing API routes can be easily accomplished with a proxy setup. By routing requests through the proxy, developers can ensure that API interactions behave as expected.
Using tools like Postman, requests can be sent to the proxy, and the responses can be validated against expected results. This simplifies testing processes, as developers do not need to set up different environments for testing API calls.
Example of a request in Postman:
- Set the request URL to http://localhost:3000/api/resource
.
- Observe the response from the target server, which is proxied through Webpack.
Handling WebSocket Connections
WebSocket connections allow for real-time communication between the client and server. Proxies can also be configured to handle WebSocket connections, ensuring that real-time data can flow seamlessly.
Example configuration:
// webpack.config.js module.exports = { devServer: { proxy: { '/socket': { target: 'http://localhost:5000', ws: true, // Enable WebSocket proxying changeOrigin: true, }, }, }, };
Setting ws: true
ensures that WebSocket connections are properly proxied to the target server.
Debugging Proxy Requests in Development
Debugging proxy requests can be achieved by using browser developer tools and logging in the server console.
In the browser, the Network tab provides visibility into the requests being made. Developers can inspect headers, payloads, and responses to troubleshoot issues.
On the server side, using logging tools or middleware can reveal how requests are being processed. This can help identify where issues are occurring in the proxy setup.
Example of enabling logging in the proxy:
// webpack.config.js const { createProxyMiddleware } = require('http-proxy-middleware'); module.exports = { devServer: { before: function(app) { app.use('/api', createProxyMiddleware({ target: 'http://localhost:5000', changeOrigin: true, logLevel: 'debug', // Enable detailed logging })); }, }, };
This logging configuration will provide insights into the proxy activity, helping identify any potential misconfigurations or issues.
Related Article: How to Use Webpack Tree Shaking for Smaller Bundles
Additional Resources
- Webpack DevServer