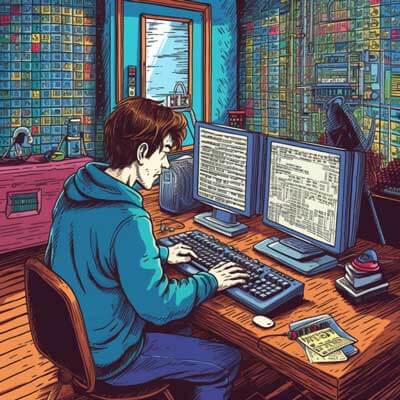
Table of Contents
To calculate the square root of a number in Python, you can make use of the math
module. The math
module provides a function called sqrt()
which can be used to calculate the square root. Here's how you can do it:
import math number = 16 square_root = math.sqrt(number) print(square_root)
In this example, we first import the math
module using the import
statement. Then, we define a variable called number
and assign it a value of 16
. We then use the math.sqrt()
function to calculate the square root of number
and store the result in a variable called square_root
. Finally, we print the value of square_root
using the print()
function.
The output of the above code will be:
4.0
This means that the square root of 16 is 4.
Handling Negative Numbers
The math.sqrt()
function can also handle negative numbers. When you pass a negative number to the function, it will return a complex number representing the square root of that negative number. Here's an example:
import math number = -9 square_root = math.sqrt(number) print(square_root)
The output of the above code will be:
(1.8369701987210297e-16+3j)
In this case, the square root of -9 is a complex number with a very small real part and an imaginary part.
Related Article: How to Use a Foreach Function in Python 3
Alternative Method: Using Exponentiation
Another way to calculate the square root of a number in Python is by using exponentiation. You can raise a number to the power of 0.5
to find its square root. Here's an example:
number = 16 square_root = number ** 0.5 print(square_root)
The output of the above code will be the same as before:
4.0
This method can be useful if you don't want to use the math
module or if you're working in an environment where the math
module is not available.
Best Practices
Related Article: Python Bitwise Operators Tutorial
When calculating square roots in Python, it is important to keep the following best practices in mind:
1. Import the math
module: Before using the sqrt()
function, make sure to import the math
module using the import
statement.
2. Handle exceptions: When dealing with user input or variables that can potentially be negative, consider using exception handling to handle cases where the square root is not defined. For example:
import math number = float(input("Enter a number: ")) try: square_root = math.sqrt(number) print(square_root) except ValueError: print("Invalid input. Cannot calculate square root of a negative number.")
In this example, we use the float()
function to convert the user input to a floating-point number. Then, we use a try-except
block to catch the ValueError
that may occur if the user enters a negative number. If a negative number is entered, an error message is displayed instead of trying to calculate the square root.
3. Use meaningful variable names: When writing code, it is good practice to use variable names that accurately describe the purpose of the variable. For example, instead of using number
, you could use input_number
or value_to_calculate
.
4. Document your code: If you are writing code that will be used by others or reviewed by a team, it is important to add comments or docstrings to explain the purpose and behavior of your code. This can make it easier for others to understand and maintain your code.