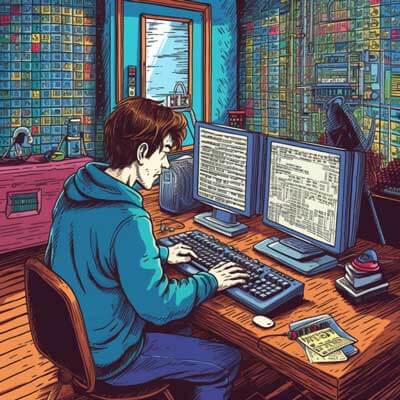
Table of Contents
Flexbox is a useful CSS layout module that provides a flexible and efficient way to arrange and align elements within a container. One of the common use cases is to center elements both horizontally and vertically. In this guide, we will explore different approaches to achieve this using flexbox.
Method 1: Using flexbox properties
To center elements both horizontally and vertically using flexbox, you can make use of the following flexbox properties:
1. Set the parent container to display flex:
.container { display: flex; justify-content: center; align-items: center; }
In the above example, the display: flex
property is applied to the container element. This enables the flex layout for its child elements. By default, flex items are laid out in a row. To center them horizontally, we use the justify-content: center
property. To center them vertically, we use the align-items: center
property.
2. Apply width and height to the flex items:
.item { width: 200px; height: 200px; }
In the above example, we set a fixed width and height for the flex items. Adjust these values based on your requirements.
Here's a complete example:
<div class="container"> <div class="item">Centered Element</div> </div>
.container { display: flex; justify-content: center; align-items: center; } .item { width: 200px; height: 200px; }
Related Article: How to Vertically Center Text with CSS
Method 2: Using margin:auto
Another approach to center elements both horizontally and vertically using flexbox is by leveraging the margin: auto
property. This method works when you have a single element or a limited number of elements to center.
1. Set the parent container to display flex:
.container { display: flex; }
2. Apply auto margin to the flex items:
.item { margin: auto; }
Here's a complete example:
<div class="container"> <div class="item">Centered Element</div> </div>
.container { display: flex; } .item { margin: auto; }
Best Practices and Alternative Ideas
Related Article: How to Vertically Align Text in a Div with CSS
- Use the first method with justify-content: center
and align-items: center
when you have multiple elements to center within a container.
- Use the second method with margin: auto
when you have a single element or a limited number of elements to center.
- Consider using a combination of flexbox and other CSS properties to achieve more complex centering requirements, such as centering elements only horizontally or only vertically.