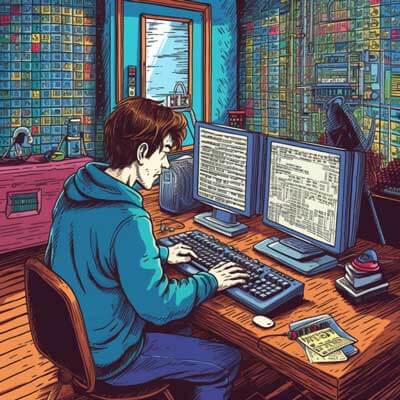
Table of Contents
To check whether a string is empty or not in Python, you can use a variety of approaches. In this answer, we will explore two common methods for checking empty strings in Python.
Method 1: Using the len() function
One simple method to check if a string is empty is to use the len() function. The len() function returns the length of a string. If the length of the string is 0, it means the string is empty.
Here is an example:
string = "" if len(string) == 0: print("The string is empty") else: print("The string is not empty")
In this example, we define a string variable named string
and assign an empty string to it. We then use an if-else statement to check if the length of string
is equal to 0. If it is, we print "The string is empty"; otherwise, we print "The string is not empty".
Related Article: How to Use Pandas to Read Excel Files in Python
Method 2: Using the not operator
Another way to check if a string is empty is by using the not operator. The not operator can be used to negate the truth value of an expression. In the case of strings, an empty string evaluates to False, while a non-empty string evaluates to True.
Here is an example:
string = "" if not string: print("The string is empty") else: print("The string is not empty")
In this example, we again define a string variable named string
and assign an empty string to it. We use an if-else statement with the not operator to check if string
is empty. If it is, we print "The string is empty"; otherwise, we print "The string is not empty".
Best Practices
Related Article: Python Command Line Arguments: How to Use Them
When checking for an empty string in Python, it is important to consider a few best practices:
1. Use the len()
function when you need to perform additional operations on the string's length.
2. Use the not
operator for simple checks where you only need to determine if the string is empty or not.
3. Be mindful of whitespace characters. A string that only contains spaces or tabs is not considered empty by Python's default behavior. To account for whitespace, you can use the strip()
method to remove leading and trailing whitespace before performing the check.
Here is an example that demonstrates the use of strip()
to check for empty strings:
string = " " if not string.strip(): print("The string is empty or contains only whitespace") else: print("The string is not empty")
In this example, the strip()
method is used to remove leading and trailing whitespace from the string before performing the check. If the resulting string is empty, we print "The string is empty or contains only whitespace"; otherwise, we print "The string is not empty".