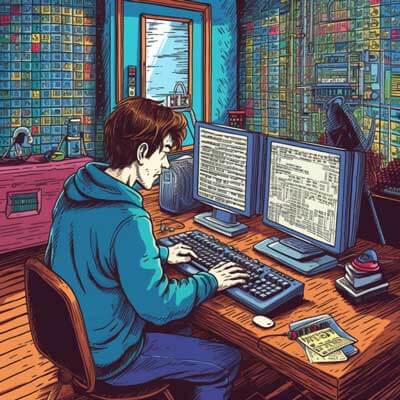
Table of Contents
There are several ways to check for null values in JavaScript. In this answer, we will explore two common approaches: using the strict equality operator (===) and using the typeof operator. We will also discuss best practices and provide examples for each method.
Using the strict equality operator (===)
The strict equality operator (===) compares both the value and the type of the operands. This means that it will only return true if the values being compared are both null.
Here is an example of how to use the strict equality operator to check for null:
let value = null; if (value === null) { console.log("The value is null"); } else { console.log("The value is not null"); }
In this example, the if statement checks if the value is strictly equal to null. If it is, the console will log "The value is null". Otherwise, it will log "The value is not null".
It is important to note that the strict equality operator will not treat undefined as null. If you need to check for both null and undefined values, you can use the loose equality operator (==) instead.
Related Article: How to Get Selected Option From Dropdown in JQuery
Using the typeof operator
Another way to check for null values in JavaScript is by using the typeof operator. The typeof operator returns a string indicating the type of the operand.
Here is an example of how to use the typeof operator to check for null:
let value = null; if (typeof value === "object" && value === null) { console.log("The value is null"); } else { console.log("The value is not null"); }
In this example, the if statement first checks if the typeof value is "object" and then checks if the value is strictly equal to null. If both conditions are true, the console will log "The value is null". Otherwise, it will log "The value is not null".
It is important to note that the typeof operator returns "object" for null values. Therefore, we need to perform an additional check to ensure that the value is actually null.
Best Practices
Related Article: How to Manage State in Next.js
When checking for null values in JavaScript, consider the following best practices:
1. Use the strict equality operator (===) when you only want to check for null values. This ensures that the comparison is both value-based and type-based.
2. Use the typeof operator when you need to check for null values and also want to perform additional type checks.
3. Be cautious when using the loose equality operator (==) to check for null values. It may lead to unexpected results due to JavaScript's type coercion rules.
4. Avoid using the typeof operator alone to check for null values. The typeof operator returns "object" for null values, so an additional null check is necessary.