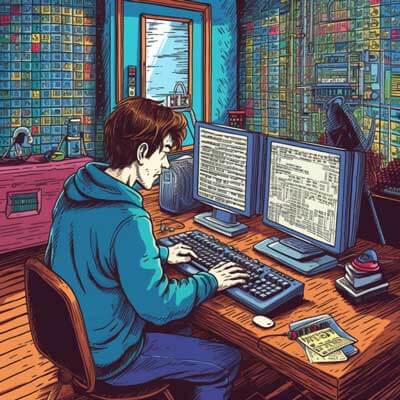
Table of Contents
To check if something is not in a Python list, you can use the not in
operator. This operator allows you to check if a value is not present in a list. It returns True
if the value is not found in the list, and False
otherwise.
Here are two ways to check if something is not in a Python list:
1. Using the not in
operator
You can use the not in
operator to check if a value is not present in a list. The syntax is as follows:
value not in list
Here, value
is the value you want to check for, and list
is the list in which you want to search for the value.
Example:
numbers = [1, 2, 3, 4, 5] # Check if 6 is not in the list if 6 not in numbers: print("6 is not in the list") # Check if 3 is not in the list if 3 not in numbers: print("3 is not in the list")
Output:
6 is not in the list
In the example above, the first if
statement is true because 6 is not present in the numbers
list. The second if
statement is false because 3 is present in the numbers
list.
Related Article: String Interpolation in Python Explained
2. Using the in
operator with the not
keyword
Alternatively, you can use the in
operator with the not
keyword to achieve the same result. The syntax is as follows:
not value in list
Example:
numbers = [1, 2, 3, 4, 5] # Check if 6 is not in the list if not 6 in numbers: print("6 is not in the list") # Check if 3 is not in the list if not 3 in numbers: print("3 is not in the list")
Output:
6 is not in the list
In this example, the output is the same as the previous example. The first if
statement is true because 6 is not present in the numbers
list, and the second if
statement is false because 3 is present in the numbers
list.
These are the two ways to check if something is not in a Python list. You can choose the one that you find more readable or intuitive.
Best Practices
Related Article: How to Define a Function with Optional Arguments in Python
When checking if something is not in a Python list, keep the following best practices in mind:
- Use the not in
operator or the in
operator with the not
keyword, as they provide a concise and readable way to perform the check.
- If you need to check for multiple values, you can use a loop or list comprehension to iterate over the values and perform the check for each value.
- Consider using sets instead of lists if you have a large number of values to check, as sets offer faster membership tests compared to lists.
These best practices can help you write clean and efficient code when checking if something is not in a Python list.