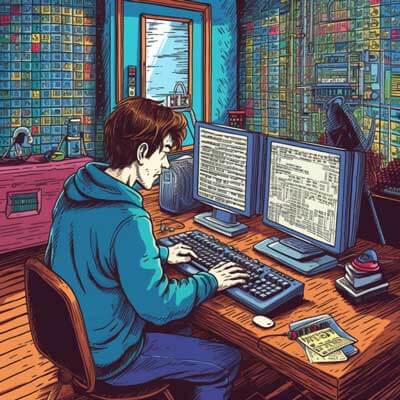
Table of Contents
Overview of Module Bundlers and Task Runners
Module bundlers and task runners are essential tools in modern web development. Module bundlers, such as Webpack, combine various assets and modules into a single file or a few files. This is crucial for optimizing load times and managing dependencies in large applications. On the other hand, task runners, like Gulp, automate repetitive tasks such as minification, compilation, and image optimization. Both tools can improve the development workflow, but they serve different purposes.
Related Article: How to Fix webpack Build Cannot Find Name ‘vi’ Vitest
Key Differences Between Gulp and Webpack
Gulp focuses on task automation, allowing developers to define a series of tasks in a declarative style. It operates on streams, which means it can handle files in memory without needing to write them to disk until the final output.
Webpack, in contrast, is primarily a module bundler. It analyzes the dependency graph of your application and bundles everything together. This bundling includes JavaScript files, CSS, images, and more, producing optimized output for production.
When to Prefer Gulp Over Webpack
Gulp may be the better choice when the project involves a lot of repetitive tasks that require automation, such as file manipulation or running tests. If the focus is on processing files in various ways without needing a complex dependency graph, Gulp's stream-based approach can be advantageous.
Using Gulp and Webpack Together
Combining Gulp and Webpack can be beneficial for leveraging the strengths of both tools. Use Gulp for task automation, such as image optimization or running a local development server, while relying on Webpack for module bundling and managing dependencies.
An example of running Gulp alongside Webpack might include a Gulp task that watches for file changes and triggers Webpack to rebuild the bundle.
// gulpfile.js const gulp = require('gulp'); const webpack = require('webpack-stream'); gulp.task('scripts', function () { return gulp.src('src/index.js') .pipe(webpack(require('./webpack.config.js'))) .pipe(gulp.dest('dist/')); }); gulp.task('watch', function () { gulp.watch('src/**/*.js', gulp.series('scripts')); });
Related Article: How to Optimize CSS Assets with Webpack Plugin
Advantages of Using Webpack
Webpack provides several advantages, including:
1. Dependency Management: Automatically resolves module dependencies and creates an optimized bundle.
2. Code Splitting: Allows splitting the code into several bundles that can be loaded on demand, improving load times.
3. Hot Module Replacement (HMR): Enables live reloading of modules without a full page refresh, enhancing the development experience.
Handling File Dependencies with Webpack
File dependencies are handled through a configuration file, typically webpack.config.js
. Webpack analyzes the entry point and recursively includes all dependent modules.
// webpack.config.js module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: __dirname + '/dist' }, module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader' } } ] } };
Configuration Files in Gulp and Webpack
Gulp uses a gulpfile.js
for configuration, where tasks are defined and can be run from the command line. Webpack requires a webpack.config.js
file, which specifies the entry points, output settings, and loaders for processing different file types.
Managing Tasks with Gulp Compared to Webpack
Task management in Gulp is based on the concept of defining tasks explicitly and running them in a sequence or parallel. It offers a straightforward syntax for chaining tasks.
Webpack, however, does not have a built-in task runner. It focuses on bundling files and requires additional configuration to manage tasks, usually through plugins or loaders.
Related Article: How to Fix Angular Webpack Plugin Initialization Error
Features Exclusive to Webpack
Webpack includes features like:
1. Code Splitting: Split code into smaller chunks that can be loaded on demand.
2. Tree Shaking: Remove unused code from the final bundle to reduce size.
3. Module Federation: Enable multiple Webpack builds to work together.
Asset Management
Gulp handles asset management through plugins that process files as streams. For example, developers can use plugins to optimize images, compile Sass to CSS, or concatenate files.
Webpack manages assets through loaders and plugins that define how different file types should be processed. This includes handling images, CSS files, and even fonts.
Code Splitting Techniques in Webpack
Code splitting is a crucial feature in Webpack. It can be achieved through:
1. Entry Points: Define multiple entry points in the configuration.
2. Dynamic Imports: Use the import()
syntax to load modules on demand.
Example of dynamic imports:
// src/index.js import('./moduleA').then(module => { // Use the loaded module });
Tree Shaking Capabilities of Webpack
Tree shaking is a process where Webpack eliminates unused code from the final bundle. For this to work, code must be written in ES6 module syntax. Webpack analyzes the dependencies and removes parts of the code that are not used.
Example of tree shaking in action:
// src/util.js export const usedFunction = () => {}; export const unusedFunction = () => {}; // src/index.js import { usedFunction } from './util'; usedFunction();
In this case, unusedFunction
will be removed from the final bundle.
Related Article: How to Use webpack -d for Development Mode
Hot Module Replacement in Webpack
Hot Module Replacement (HMR) allows developers to update modules in a running application without a full reload. This leads to a better development experience since the application state is preserved.
To enable HMR, configure it in webpack.config.js
:
// webpack.config.js const webpack = require('webpack'); module.exports = { // Other configurations... plugins: [ new webpack.HotModuleReplacementPlugin() ] };
Minification Processes in Gulp and Webpack
Minification reduces file sizes by removing whitespace, comments, and other unnecessary characters. Gulp handles this through plugins like gulp-uglify
for JavaScript and gulp-clean-css
for CSS.
Example of minifying JavaScript with Gulp:
// gulpfile.js const uglify = require('gulp-uglify'); gulp.task('minify-js', function () { return gulp.src('src/**/*.js') .pipe(uglify()) .pipe(gulp.dest('dist/')); });
Webpack can minify during the build process using the TerserPlugin
for JavaScript and css-minimizer-webpack-plugin
for CSS.
// webpack.config.js const TerserPlugin = require('terser-webpack-plugin'); module.exports = { optimization: { minimize: true, minimizer: [new TerserPlugin()], }, };
Transpilation Support in Gulp and Webpack
Transpilation converts modern JavaScript into a version compatible with older browsers. Gulp accomplishes this using babel
and gulp-babel
.
Example of using Babel with Gulp:
// gulpfile.js const babel = require('gulp-babel'); gulp.task('transpile', function () { return gulp.src('src/**/*.js') .pipe(babel()) .pipe(gulp.dest('dist/')); });
Webpack uses babel-loader
to transpile files during the bundling process.
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: 'babel-loader', }, ], }, };
Common Use Cases
Gulp is ideal for automating tasks such as:
- Image optimization
- SASS/LESS compilation
- Running tests
Webpack excels in scenarios involving:
- Single-page applications (SPAs)
- Complex dependency management
- State management in larger applications
Related Article: How to Set Up Webpack SCSS Loader
Best Practices for Large Projects
For large projects, consider the following best practices:
1. Modular Configuration: Split Gulp and Webpack configurations into smaller, manageable files.
2. Lazy Loading: Use code splitting and dynamic imports to load resources only when needed.
3. Use Environment Variables: Different configurations for development and production environments.
Development Server Options in Gulp and Webpack
Gulp can serve files using the gulp-connect
or browser-sync
plugins. These provide a way to create a simple development server that supports live reloading.
Example of setting up browser-sync
:
// gulpfile.js const browserSync = require('browser-sync').create(); gulp.task('serve', function () { browserSync.init({ server: './dist' }); gulp.watch('src/**/*.js', gulp.series('scripts')).on('change', browserSync.reload); });
Webpack provides a built-in development server, webpack-dev-server
, which supports hot module replacement and serves files from memory.
To configure the Webpack dev server:
// webpack.config.js module.exports = { devServer: { contentBase: './dist', hot: true, }, };