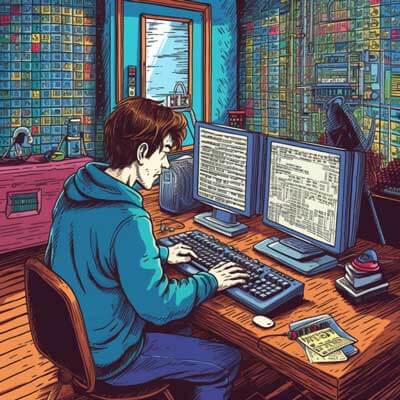
Table of Contents
Choosing between the href
attribute and the onclick
event for callbacks in JavaScript depends on the specific use case and requirements of your application. Both options have their own advantages and considerations, and it's important to understand them in order to make an informed decision.
Using Href for Callbacks
The href
attribute is commonly used to specify the destination of a hyperlink in HTML. However, it can also be utilized to trigger JavaScript functions by using the javascript:
protocol. This approach has some benefits:
1. Accessibility: By using href
for callbacks, you can ensure that users who have JavaScript disabled or are using assistive technologies can still access the functionality provided by the link. This is because the link will lead to a fallback URL that provides an alternative experience.
2. Bookmarking and History: When using the href
attribute, the callback can be bookmarked and shared with others. Additionally, it will be added to the browser's history, allowing users to navigate back to it using the browser's back button.
Here's an example of using href
for a callback:
<a href="myFunction()">Click me</a> function myFunction() { // Code to be executed when the link is clicked }
While using href
for callbacks can be convenient in some cases, there are also some considerations to keep in mind:
1. URL Manipulation: When using href
for callbacks, the URL of the page will change, which may not be desirable in certain scenarios. If you don't want the URL to change, you can prevent the default behavior of the link by returning false
from the callback function or by using the event.preventDefault()
method.
2. Code Separation: Mixing JavaScript code directly in the href
attribute can lead to a lack of code separation and maintainability. It is generally recommended to separate JavaScript code into external files or use event listeners to keep the code organized.
Related Article: How to Fetch a Parameter Value From a Query String in React
Using Onclick for Callbacks
Related Article: How to Create a Countdown Timer with Javascript
The onclick
event is a JavaScript event that occurs when an element is clicked. It allows you to directly specify a JavaScript function to be executed when the event is triggered. Here are some advantages of using onclick
for callbacks:
1. Code Separation: By using onclick
for callbacks, you can keep your HTML markup clean and separate JavaScript code in external files or script tags. This improves code maintainability and readability.
2. Flexibility: onclick
allows you to attach event handlers to any HTML element, not just links. This provides more flexibility in terms of the UI elements you can use to trigger your callbacks.
Here's an example of using onclick
for a callback:
<button>Click me</button> function myFunction() { // Code to be executed when the button is clicked }
However, there are a few considerations when using onclick
for callbacks:
1. Accessibility: Using onclick
for callbacks may not provide an accessible fallback for users who have JavaScript disabled or are using assistive technologies. It's important to ensure that the functionality provided by the callback is also accessible through alternative means.
2. Event Binding: When using onclick
, the event handler is directly bound to the HTML element, which can make it harder to dynamically add or remove event listeners. If you need to attach or detach event listeners dynamically, using event delegation or addEventListener
may be more appropriate.