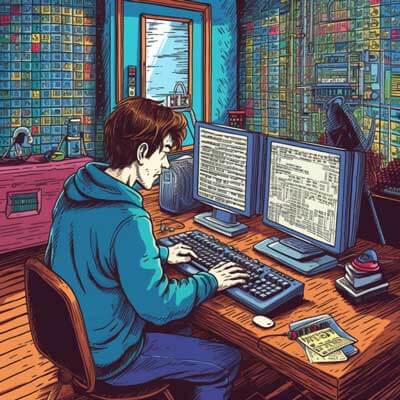
Table of Contents
When working with JSON data in JavaScript, it's important to choose the correct date format to ensure accurate representation and manipulation of dates. In this answer, we will explore different JSON date formats and discuss how to choose the right one for your specific use case.
1. Using ISO 8601 Date Format
The ISO 8601 date format is widely accepted and recommended for representing dates in JSON. It provides a standardized way to represent dates and times in a format that is both human-readable and machine-readable. The ISO 8601 format follows the pattern "YYYY-MM-DDTHH:mm:ss.sssZ", where:
- "YYYY" represents the four-digit year.
- "MM" represents the two-digit month (01-12).
- "DD" represents the two-digit day of the month (01-31).
- "T" is a literal character separating the date and time components.
- "HH" represents the two-digit hour (00-23).
- "mm" represents the two-digit minute (00-59).
- "ss" represents the two-digit second (00-59).
- "sss" represents the milliseconds (000-999).
- "Z" represents the time zone offset in the format "+/-HH:mm".
Here's an example of a date in ISO 8601 format: "2022-01-01T12:00:00.000Z".
When working with JSON dates in JavaScript, you can use the built-in Date
object to parse and format dates in the ISO 8601 format. The Date
object provides various methods for manipulating and extracting different components of a date.
Related Article: How to Install & Configure Nodemon with Nodejs
2. Unix Timestamp Format
Another common approach to representing dates in JSON is by using Unix timestamps. A Unix timestamp represents the number of seconds or milliseconds that have elapsed since the Unix epoch (January 1, 1970, 00:00:00 UTC).
In the Unix timestamp format, dates are represented as numeric values. For example, the Unix timestamp for "2022-01-01T12:00:00.000Z" is 1641043200 (in seconds) or 1641043200000 (in milliseconds).
Using Unix timestamps can simplify date manipulation and calculations, as they can be easily converted to and from Date
objects in JavaScript. You can use the getTime()
method of the Date
object to obtain the Unix timestamp of a specific date, and the setTime()
method to set a Date
object based on a Unix timestamp.
Here's an example of converting a Unix timestamp to a Date
object in JavaScript:
const unixTimestamp = 1641043200; // Unix timestamp in seconds const date = new Date(unixTimestamp * 1000); // Convert to milliseconds console.log(date.toISOString()); // Output: 2022-01-01T12:00:00.000Z
Choosing the Right Date Format
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
When choosing the correct JSON date format in JavaScript, consider the following factors:
1. Compatibility: Ensure that the chosen date format is widely supported by other systems or APIs that consume or provide JSON data. ISO 8601 and Unix timestamps are generally well-supported across different platforms and programming languages.
2. Readability: Choose a date format that is easily understandable by humans, as it can facilitate debugging and troubleshooting. ISO 8601 dates are human-readable and provide clear separation of date and time components.
3. Consistency: If you are working with a team or integrating with other systems, it's important to establish consistency in the date format used across all components. Using a standardized format like ISO 8601 or Unix timestamps can help ensure consistency and avoid confusion.
4. Granularity: Consider the level of precision required for your specific use case. If you only need to represent dates without considering the time component, you can omit the time portion in the chosen date format. On the other hand, if you need to represent dates with millisecond precision, ensure that the chosen format supports it.
5. Time Zone Handling: If your application needs to handle dates in different time zones, consider using the ISO 8601 format with the time zone offset included. This ensures that the date is correctly interpreted in the appropriate time zone.