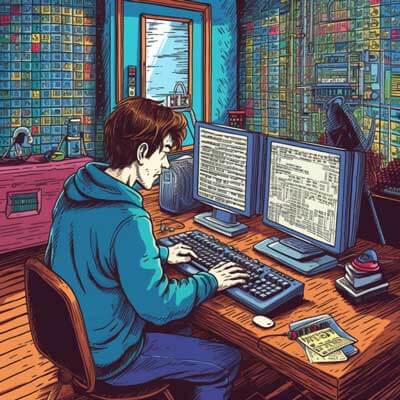
Table of Contents
Comparing dates in JavaScript can be done using various methods and approaches. In this answer, we will explore two common techniques to compare two dates in JavaScript.
Method 1: Using the Comparison Operator
One straightforward way to compare two dates in JavaScript is by using the comparison operator (,
=
). Since JavaScript treats dates as objects, you can use the comparison operator to compare them directly. Here's an example:
const date1 = new Date('2022-01-01'); const date2 = new Date('2022-01-02'); if (date1 date2) { console.log('date1 is after date2'); } else { console.log('date1 and date2 are the same'); }
In this example, we create two Date
objects, date1
and date2
, representing different dates. We then use the comparison operator to check whether date1
is before, after, or the same as date2
.
Related Article: How to Install & Configure Nodemon with Nodejs
Method 2: Using the getTime() Method
Another approach to compare dates in JavaScript is by using the getTime()
method. The getTime()
method returns the numeric value representing the time in milliseconds since January 1, 1970 (Unix Epoch). By comparing the numeric values returned by getTime()
, you can determine the relationship between two dates. Here's an example:
const date1 = new Date('2022-01-01'); const date2 = new Date('2022-01-02'); if (date1.getTime() date2.getTime()) { console.log('date1 is after date2'); } else { console.log('date1 and date2 are the same'); }
In this example, we use the getTime()
method to obtain the numeric values of date1
and date2
, and then compare them using the comparison operators.
Using the getTime()
method is particularly useful when you need to compare dates with more precision, such as when comparing dates with different time components (e.g., hours and minutes).
Considerations and Best Practices
When comparing dates in JavaScript, it's important to keep the following considerations in mind:
1. Timezone: JavaScript dates are based on the user's system timezone. Ensure that you are comparing dates in the same timezone or account for timezone differences when necessary.
2. Date formatting: Make sure the dates are in a format that JavaScript can understand. The ISO 8601 format (YYYY-MM-DD
) is widely supported and recommended.
3. Avoid using the ==
operator: When comparing dates, it is generally recommended to use the comparison operators (,
=
) instead of the equality operator (==
). This is because the equality operator may perform type coercion, leading to unexpected results.
4. Consider using a library: If you need to perform more advanced date operations or handle complex scenarios, consider using a date manipulation library like Moment.js or Luxon. These libraries provide additional functionality and make working with dates more convenient.
Alternative Approach: Using Date Methods
In addition to the approaches mentioned above, JavaScript's Date
object provides several other methods that can be used to compare dates. Some of these methods include getFullYear()
, getMonth()
, getDate()
, getHours()
, getMinutes()
, getSeconds()
, etc. By comparing the values returned by these methods, you can determine the relationship between two dates. However, this approach can be more verbose and may require more code compared to the previous methods.
Here's an example using the getFullYear()
method:
const date1 = new Date('2022-01-01'); const date2 = new Date('2022-01-02'); if (date1.getFullYear() date2.getFullYear()) { console.log('date1 is after date2'); } else { console.log('date1 and date2 are in the same year'); }
In this example, we compare the years of date1
and date2
using the getFullYear()
method. You can extend this approach to compare other date components like months, days, hours, etc., as needed.