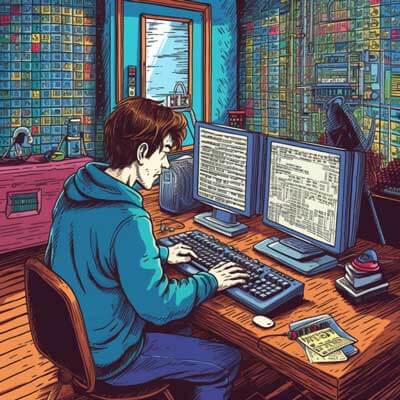
Table of Contents
Vite and Webpack serve as module bundlers for JavaScript applications. Vite focuses on speed and simplicity, while Webpack offers extensive features and configurations. Vite uses native ES modules during development, providing faster hot module replacement and a more responsive development experience. Webpack, on the other hand, compiles all assets into a single output, which can be beneficial for larger projects with complex configurations.
Main Purpose of a Module Bundler
The main purpose of a module bundler is to package various assets like JavaScript files, CSS, images, and other resources into a single bundle or multiple bundles. This process optimizes loading times and improves overall performance by reducing the number of HTTP requests made by the browser. Module bundlers also allow developers to use modern JavaScript features and modules while ensuring compatibility across different browsers.
Related Article: How to Use Extract Text Webpack Plugin
Comparison of Build Tools in Vite and Webpack
Vite employs a build toolchain that uses Rollup for production, which results in optimized bundles. Webpack's build process is highly customizable through loaders and plugins, allowing for granular control over how files are processed. Developers may find Vite easier to set up for smaller projects, while Webpack might be preferred for larger applications requiring advanced configurations.
Configuration Files
Vite uses a simple configuration file named vite.config.js
. This file allows developers to define various settings such as server options, plugins, and build configurations.
Example of a basic vite.config.js
file:
// vite.config.js import { defineConfig } from 'vite'; export default defineConfig({ server: { port: 3000, }, build: { outDir: 'dist', }, });
Webpack requires a webpack.config.js
file, which can be more complex due to the numerous options available. This file allows for detailed customization of the build process, including entry points, output settings, loaders, and plugins.
Example of a basic webpack.config.js
file:
// webpack.config.js const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', }, }, ], }, };
Asset Management Techniques in Vite and Webpack
Vite simplifies asset management through its built-in support for various file types. Developers can import images, CSS, and other assets directly in JavaScript files. Vite handles these imports automatically, making it easier to manage assets without additional configuration.
Webpack relies on loaders to process different asset types. Each loader handles a specific file type, allowing developers to customize how assets are processed. For example, developers can use file-loader
or url-loader
to manage image assets.
Example of using file-loader
in webpack.config.js
:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.(png|jpg|gif)$/, use: { loader: 'file-loader', options: { name: '[path][name].[ext]', outputPath: 'images/', }, }, }, ], }, };
Related Article: How to Bypass a Router with Webpack Proxy
Hot Module Replacement Features
Hot Module Replacement (HMR) allows developers to see changes in real-time without refreshing the page. Vite provides HMR out of the box, taking advantage of native ES modules for instant updates. This results in a more responsive development experience.
Webpack also supports HMR, but it requires additional configuration. Developers must set up the HotModuleReplacementPlugin
and configure the webpack dev server to enable HMR.
Example of enabling HMR in webpack.config.js
:
// webpack.config.js const webpack = require('webpack'); module.exports = { plugins: [ new webpack.HotModuleReplacementPlugin(), ], devServer: { hot: true, }, };
Code Splitting Mechanisms
Code splitting improves loading times by breaking the application into smaller chunks. Vite automatically handles code splitting, leveraging dynamic imports to load modules as needed. This feature simplifies the process for developers, allowing for more efficient loading without extra configuration.
Webpack requires explicit configuration for code splitting. Developers must specify entry points or use dynamic imports to create separate bundles. The optimization.splitChunks
option can also be configured to manage how chunks are created.
Example of configuring code splitting in webpack.config.js
:
// webpack.config.js module.exports = { optimization: { splitChunks: { chunks: 'all', }, }, };
Tree Shaking Benefits
Tree shaking is a technique used to eliminate dead code from the final bundle. Vite benefits from Rollup's tree-shaking capabilities, ensuring that only the code that is actually used gets included in the final build. This results in smaller bundle sizes and improved performance.
Webpack also supports tree shaking, but it relies on ES module syntax and configuration to achieve optimal results. Developers must ensure that the mode
is set to production
to enable tree shaking, as it is only active in that mode.
Example of enabling tree shaking in webpack.config.js
:
// webpack.config.js module.exports = { mode: 'production', };
Performance Optimization Strategies
Vite optimizes performance using techniques such as pre-bundling dependencies and leveraging native ES modules. The development server is fast and responsive, providing a smooth experience during development.
Webpack offers various performance optimization strategies through its configuration options. Developers can enable features such as code splitting, tree shaking, and minification to improve overall performance.
Example of enabling minification in webpack.config.js
:
// webpack.config.js const TerserPlugin = require('terser-webpack-plugin'); module.exports = { optimization: { minimize: true, minimizer: [new TerserPlugin()], }, };
Related Article: How to Configure Electron with Webpack
Plugin Ecosystem
Vite has a growing plugin ecosystem that allows developers to extend its functionality easily. Plugins can be added in the vite.config.js
file and provide additional features like support for TypeScript, JSX, or CSS preprocessors.
Webpack has a more extensive plugin ecosystem due to its longer existence. Developers can find plugins for various tasks, from optimizing builds to enhancing development experiences. Adding plugins requires configuration in the webpack.config.js
file.
Example of adding a plugin to Vite:
// vite.config.js import { defineConfig } from 'vite'; import reactRefresh from '@vitejs/plugin-react-refresh'; export default defineConfig({ plugins: [reactRefresh()], });
Example of adding a plugin to Webpack:
// webpack.config.js const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', }), ], };
Development Server Capabilities
Vite features a fast development server that supports HMR out of the box. It is optimized for speed, allowing developers to see changes in real-time without refreshing the entire page. The server can be configured easily through the vite.config.js
file.
Webpack provides a development server through the webpack-dev-server
package. This server can be configured to support HMR, but it may require additional setup. The development server can serve static files and handle API requests, making it versatile for various development scenarios.
Example of starting a Vite development server:
# Start Vite development server npm run dev
Example of starting a Webpack development server:
# Start Webpack development server npx webpack serve
Advantages of Using Vite Over Webpack
Vite offers several advantages, particularly in development speed and ease of use. It uses native ES modules, resulting in faster hot module replacement and a more responsive development experience. Configuration is simpler, making it easier to get started with smaller projects.
Webpack provides greater flexibility and a more extensive plugin ecosystem, making it suitable for larger applications with complex requirements. It allows for detailed customization of the build process, which can be beneficial for experienced developers.
Setting Up a New Project
Creating a new project with Vite is quick and straightforward. Developers can use the following command to set up a new Vite project:
# Initialize a new Vite project npm create vite@latest my-vite-app --template vanilla
For Webpack, the setup is slightly more involved. Developers can create a new project using npm and then install Webpack and its CLI:
# Initialize a new npm project npm init -y # Install Webpack and Webpack CLI npm install --save-dev webpack webpack-cli
After setting up, developers need to create a webpack.config.js
file and add the necessary scripts in the package.json
.
Related Article: How to Fix Angular Webpack Plugin Initialization Error
Asset Management Comparison
Vite simplifies asset management by allowing direct imports of various file types. Developers can import images, CSS, and other assets seamlessly without additional configuration.
Webpack requires specific loaders to handle different asset types. Developers must set up each loader to process files appropriately, which can add complexity to the configuration.
Example of importing an image in Vite:
// main.js import image from './assets/image.png'; const img = document.createElement('img'); img.src = image; document.body.appendChild(img);
Example of importing an image in Webpack:
// main.js import image from './assets/image.png'; const img = document.createElement('img'); img.src = image; document.body.appendChild(img);
Hot Module Replacement Implementation
Vite implements HMR automatically, allowing developers to see changes in real-time. When a file is modified, Vite only reloads the affected module, making it much faster compared to full page reloads.
Webpack requires specific configurations to enable HMR. Developers must include the HotModuleReplacementPlugin
and configure the dev server to support HMR. This involves more setup compared to Vite.
Example of implementing HMR in Vite:
// main.js if (import.meta.hot) { import.meta.hot.accept((newModule) => { // Handle module updates }); }
Example of implementing HMR in Webpack:
// main.js if (module.hot) { module.hot.accept('./module.js', function() { // Handle module updates }); }
Plugins
Vite allows developers to add plugins easily through the vite.config.js
file. Plugins can enhance the functionality of Vite, providing support for various features like TypeScript or JSX.
Webpack has a larger selection of plugins available due to its maturity. Developers can find plugins for different purposes, including optimization, code splitting, and asset management. Configuring plugins in Webpack typically requires more detailed settings.
Example of adding a TypeScript plugin in Vite:
// vite.config.js import { defineConfig } from 'vite'; import vue from '@vitejs/plugin-vue'; export default defineConfig({ plugins: [vue()], });
Example of adding a TypeScript plugin in Webpack:
// webpack.config.js const TsconfigPathsPlugin = require('tsconfig-paths-webpack-plugin'); module.exports = { resolve: { plugins: [new TsconfigPathsPlugin()], }, };
Code Splitting
Code splitting allows developers to break applications into smaller chunks, improving loading times. Vite handles code splitting automatically with dynamic imports, allowing developers to create separate bundles effortlessly.
Webpack requires explicit configuration for code splitting. Developers can use dynamic imports in their code or configure entry points to create separate bundles.
Example of dynamic import in Vite:
// main.js import('./module.js').then(module => { // Use the module });
Example of dynamic import in Webpack:
// main.js import('./module.js').then(module => { // Use the module });
Related Article: How to Set LibraryTarget in the Webpack Configuration
Optimizing Build Processes
Vite optimizes the build process by using Rollup for production builds. This results in optimized bundles with minimal configuration required from the developer.
Webpack allows for greater customization of the build process. Developers can configure various optimizations, including minification, code splitting, and tree shaking, to enhance performance.
Example of running a build in Vite:
# Build for production npm run build
Example of running a build in Webpack:
# Build for production npx webpack --mode production
Additional Resources
- What is Webpack?