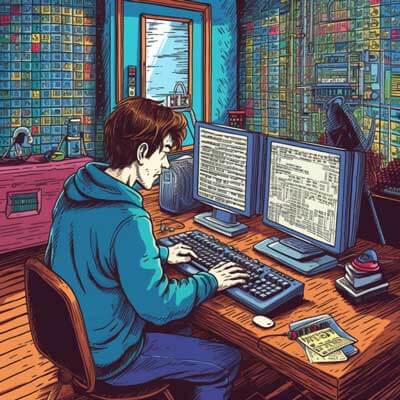
Table of Contents
Concatenating string variables in Bash is a common task that allows you to combine multiple strings into a single string. Bash provides several ways to achieve this. In this answer, we will explore two popular methods: using the concatenation operator and using the printf command.
Method 1: Using the Concatenation Operator
In Bash, you can concatenate string variables using the concatenation operator, which is the plus sign (+). Here's an example:
# Define two string variables first_name="John" last_name="Doe" # Concatenate the variables full_name=$first_name" "$last_name # Print the result echo $full_name
In this example, we define two string variables first_name
and last_name
. We then concatenate them using the concatenation operator and assign the result to the full_name
variable. Finally, we print the full_name
variable, which will output "John Doe".
Using the concatenation operator is simple and straightforward. However, it's important to note that there should be no spaces around the plus sign, as it is used for string concatenation instead of arithmetic addition.
Related Article: Locating and Moving Files in Bash Scripting on Linux
Method 2: Using the printf Command
Another way to concatenate string variables in Bash is by using the printf
command with format specifiers. Here's an example:
# Define two string variables first_name="John" last_name="Doe" # Concatenate the variables using printf full_name=$(printf "%s %s" $first_name $last_name) # Print the result echo $full_name
In this example, we use the printf
command with the format specifier %s
to concatenate the string variables first_name
and last_name
. The %s
specifier is used to indicate a string value. We pass the variables to printf
as arguments, and the resulting concatenated string is assigned to the full_name
variable. Finally, we print the full_name
variable, which will output "John Doe".
The printf
command provides more flexibility for formatting and concatenating strings compared to the concatenation operator. You can also control the order and format of the variables being concatenated by modifying the format specifier.
Alternative Ideas and Best Practices
Related Article: How to Check If a File Does Not Exist in Bash
- If you need to concatenate multiple string variables, you can chain multiple concatenation operations or printf
commands together. For example:
# Chaining concatenation operations full_name=$first_name" "$middle_name" "$last_name # Chaining printf commands full_name=$(printf "%s %s %s" $first_name $middle_name $last_name)
- When concatenating string variables that may contain spaces or special characters, it's recommended to enclose the variables in double quotes to preserve their original formatting. For example:
# Define a string variable with spaces last_name="Smith Jr." # Concatenate with double quotes full_name=$first_name" "$last_name echo $full_name # Output: John Smith Jr. # Concatenate without double quotes full_name=$first_name$last_name echo $full_name # Output: JohnSmith Jr.
- If you need to append a string to an existing variable, you can use the +=
operator. For example:
# Define a string variable greeting="Hello" # Append a string greeting+=" World" echo $greeting # Output: Hello World