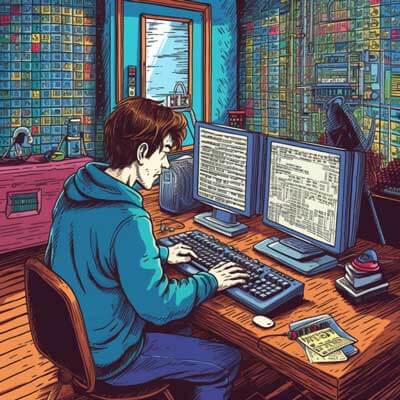
Table of Contents
Overview of Configuring DevServer Proxy
Webpack is a module bundler that allows developers to organize their JavaScript applications and manage dependencies. One of its key features is the Webpack Dev Server, which provides a simple way to serve web applications during development. A vital part of this setup is the ability to configure a proxy, which helps to redirect API requests from the client to a backend server, particularly helpful when dealing with CORS issues. This article will guide you through setting up a DevServer proxy with Webpack using clear examples.
Related Article: How to Fix webpack not recognized as a command error
Setting Up the Development Server
To start using Webpack Dev Server, you need to install it first. You can do this using npm. Run the following command in your project directory:
npm install webpack-dev-server --save-dev
Next, you need to configure the Dev Server in your Webpack configuration file, typically named webpack.config.js
. Here is an example configuration:
// webpack.config.jsconst path = require('path');module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000, },};
This setup defines the entry point for your application and specifies where the bundled files will be served from. You can run the Dev Server with the command:
npx webpack serve
Enabling Hot Module Replacement
Hot Module Replacement (HMR) allows modules to be updated in the browser without a full refresh. This feature enhances the development experience by retaining application state. To enable HMR, modify the devServer
section in your webpack.config.js
:
devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000, hot: true, // Enable Hot Module Replacement},
Ensure that your JavaScript entry point supports HMR:
// src/index.jsif (module.hot) { module.hot.accept();}
This code snippet checks if the module is hot-replaceable and accepts updates.
Configuring Proxy Settings
To configure the proxy, add a proxy
property in the devServer
configuration. This allows you to route API requests to a different server. For example, if your frontend runs on localhost:9000
and your API is on localhost:3000
, you can set up the proxy as follows:
devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000, hot: true, proxy: { '/api': { target: 'http://localhost:3000', secure: false, }, },},
With this configuration, any request starting with /api
will be forwarded to http://localhost:3000/api
.
Related Article: How to Use Webpack CopyPlugin for Asset Management
Addressing CORS Issues
CORS (Cross-Origin Resource Sharing) issues occur when your frontend tries to access resources from a different origin. By using a proxy in the Dev Server configuration, you can bypass these restrictions during development. The proxy acts as a middleman, allowing the browser to communicate with the backend without facing CORS restrictions.
In the example provided, requests to /api
are proxied to http://localhost:3000
, effectively eliminating CORS errors for those requests.
API Proxying Techniques
When setting up a proxy, you can customize the behavior based on your needs. Here are some techniques:
1. Path Rewriting: If your backend API has a different endpoint structure, you can rewrite the path. For example:
proxy: { '/api': { target: 'http://localhost:3000', pathRewrite: {'^/api' : ''}, secure: false, },},
This configuration removes /api
from the request path when forwarding to the backend.
2. Multiple Proxies: You can set up multiple proxies for different API endpoints. For example:
proxy: { '/api': { target: 'http://localhost:3000', secure: false, }, '/auth': { target: 'http://localhost:4000', secure: false, },},
This setup allows requests to /api
to be forwarded to one server while /auth
requests go to another.
Using Middleware Features
Webpack Dev Server supports middleware, which can be used to handle requests before they reach the proxy. This is useful for logging, authentication, or modifying requests.
To add middleware, you can use the before
function in the devServer
configuration:
devServer: { before: function(app, server) { app.get('/api/some-endpoint', function(req, res) { res.json({ message: 'This is a custom response' }); }); },},
In this example, when a request is made to /api/some-endpoint
, the server responds with a custom JSON response instead of going to the backend.
Serving Static Files
Serving static files is essential for development. You can specify which directory contains your static files using the contentBase
option. Typically, this is the dist
directory where your bundled files reside.
For example:
devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000,},
With this configuration, any static files in the dist
directory will be served at the root URL.
Related Article: How to generate source maps for ESM in Webpack
Creating a Comprehensive Configuration
Creating a comprehensive Webpack Dev Server configuration involves combining the various features discussed. Here is an example of a more complete webpack.config.js
:
const path = require('path');module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000, hot: true, proxy: { '/api': { target: 'http://localhost:3000', pathRewrite: {'^/api': ''}, secure: false, }, }, before: function(app, server) { app.get('/api/some-endpoint', function(req, res) { res.json({ message: 'This is a custom response' }); }); }, },};
This configuration includes HMR, static file serving, API proxying, and custom middleware.
Operating in Development Mode
Running Webpack Dev Server is typically done in development mode. This mode is optimized for development, providing features like hot reloading, enhanced error messages, and detailed logging.
You can start the server using:
npx webpack serve --mode development
This command ensures that the server runs in development mode, allowing you to take full advantage of the development features provided by Webpack.
Activating Inline Mode
Inline mode allows you to include the client script in the bundle, enabling live reloading without the need for a full page refresh. To activate inline mode, modify the devServer
configuration as follows:
devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000, hot: true, inline: true, // Enable inline mode},
With inline mode enabled, the client script is injected into the bundle, ensuring that updates are applied instantly.
Implementing File Watching
Webpack Dev Server automatically watches for file changes in your project. When changes are detected, the server will trigger a reload. You can customize the watch behavior by modifying the watchOptions
property:
devServer: { watchOptions: { poll: true, // Use polling instead of inotify aggregateTimeout: 300, // Delay the rebuild after the first change },},
The poll
option checks for changes at specified intervals, while aggregateTimeout
sets a delay to wait before rebuilding.
Related Article: How to Fix Webpack Command Not Found Error
Establishing a Proxy in DevServer
Setting up a proxy in DevServer is crucial for routing API requests. The proxy feature allows you to avoid CORS issues and simplifies backend integration. By defining the proxy
property, you can specify how requests should be handled.
For example, to proxy requests to a specific path:
proxy: { '/api': { target: 'http://localhost:3000', changeOrigin: true, },},
The changeOrigin
option modifies the origin of the host header to the target URL.
Benefits of Using a Development Server
Using a development server like Webpack Dev Server offers several benefits:
1. Live Reloading: Changes are automatically reflected in the browser without needing a full refresh.
2. HMR: Hot Module Replacement allows for seamless updates of modules.
3. Proxying: Simplifies API interactions and bypasses CORS issues.
4. Middleware Support: Enables custom request handling.
5. Static File Serving: Easily serve static assets during development.
These features enhance productivity and streamline the development process.
Handling CORS with DevServer
CORS issues often arise when the frontend and backend are served from different origins. Webpack Dev Server's proxy feature effectively resolves these issues by routing requests through the same origin. This eliminates the need for complex CORS configurations on the backend during development.
Hot Module Replacement Explained
Hot Module Replacement (HMR) allows for updates to modules in real-time without refreshing the entire page. This is particularly useful for maintaining application state, as it avoids losing unsaved changes.
When HMR is enabled, Webpack keeps track of the state of each module. When a change is detected, only that module is reloaded. To implement HMR, make sure to include the hot
option in the configuration and use module.hot.accept()
in your entry file.
Related Article: How to Set Webpack Target to Node.js
Configuring Static File Serving
For a seamless development experience, serving static files is essential. The contentBase
option in the devServer
configuration specifies the directory from which to serve static files.
For instance, if your static files are in the dist
folder, you would configure it as follows:
devServer: { contentBase: path.join(__dirname, 'dist'),},
This ensures that static files are served from the specified directory, making them accessible during development.
Necessary Settings for Middleware
When using middleware features in Webpack Dev Server, certain settings are crucial for proper functionality. The before
function allows you to define custom routes and responses.
Here’s a practical example of how to implement middleware:
devServer: { before: function(app, server) { app.get('/api/custom-endpoint', function(req, res) { res.json({ message: 'Custom response from middleware' }); }); },},
This example sets up a custom endpoint that responds with a JSON object, demonstrating how middleware can intercept requests.
Using Multiple Proxies
In complex applications, you may need to interact with multiple backend services. Webpack Dev Server allows you to set up multiple proxies easily. You can define different targets for different paths as shown below:
proxy: { '/api': { target: 'http://localhost:3000', secure: false, }, '/auth': { target: 'http://localhost:4000', secure: false, },},
This configuration directs requests to /api
and /auth
to their respective servers, facilitating seamless communication.
Troubleshooting Proxy Issues
When working with proxies, issues may arise that prevent proper routing. Common problems include:
1. Incorrect Target URL: Ensure that the target URL in the proxy configuration is correct.
2. CORS Errors: If you still encounter CORS issues, double-check that your proxy is set up correctly.
3. Network Issues: Verify that the backend server is running and accessible.
4. Browser Caching: Clear the browser cache, as old responses might interfere with new requests.
Debugging these issues often involves inspecting network requests in the browser's developer tools to ensure they are reaching the intended target.
Related Article: How to Configure Webpack for Expo Projects
Differences Between Development and Production Servers
Development servers like Webpack Dev Server are designed for local development, focusing on features that enhance the development experience. In contrast, production servers are optimized for performance and security.
Key differences include:
1. Hot Reloading: Development servers support HMR, while production servers do not.
2. Error Handling: Development servers provide detailed error messages, while production servers typically show generic messages for security.
3. Static File Handling: Production servers use optimized and minified assets, while development servers serve unminified files for easier debugging.
4. Performance Optimization: Production servers implement various optimizations to improve loading times and resource usage.
Understanding these differences helps in configuring your application for the right environment.
Improving Performance with DevServer
Optimizing performance while using Webpack Dev Server can significantly enhance the development experience. Here are some tips:
1. Enable Compression: Use the compress
option to enable gzip compression, reducing the size of files served.
devServer: { compress: true,},
2. Optimize Asset Size: Use tools like TerserWebpackPlugin
to minimize JavaScript file sizes.
3. Implement Caching: Use the cache
option in the Dev Server configuration to cache modules.
4. Limit Watch Options: If your project has many files, consider limiting the watch options to specific directories.
Incorporating these strategies can lead to a smoother and more responsive development environment.
Additional Resources
- Setting Up a Proxy in Webpack Dev Server