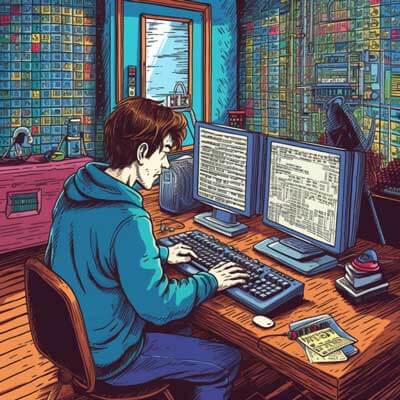
Table of Contents
Overview of Configuring Electron with Bundling
Configuring Electron with Webpack combines the benefits of both technologies. Electron allows developers to build desktop applications using web technologies, while Webpack serves as a module bundler that optimizes the application for performance. The primary goal is to package JavaScript, CSS, images, and other assets into a single bundle that can be easily managed and served. This approach helps to streamline development, improve load times, and ensure that the application runs smoothly across all platforms.
Related Article: How to Set Up Webpack SCSS Loader
Setting Up Entry Points in Electron
Entry points define where Webpack starts when it bundles the application. For Electron, there are typically two main entry points: one for the main process and one for the renderer process.
Create a
webpack.config.js
file in the root directory of your project. This file will define how Webpack processes your application.
// webpack.config.js const path = require('path'); module.exports = { entry: { main: './src/main.js', // Main process renderer: './src/renderer.js' // Renderer process }, // Other configurations... };
The
main.js
file runs the Electron application, while renderer.js
is responsible for the UI. This separation ensures that both processes are independently managed and bundled.
Configuring Output Settings
Output settings determine where Webpack will place the bundled files after processing. This is crucial for Electron applications, as the main and renderer processes need to be correctly referenced in the final build.
Add the output configuration to the
webpack.config.js
file:
// webpack.config.js output: { filename: '[name].bundle.js', // Uses entry point names path: path.resolve(__dirname, 'dist'), // Output directory publicPath: './', // Ensures paths are relative },
Webpack will now create
main.bundle.js
and renderer.bundle.js
in the dist
folder after bundling the application.
Managing Environment Variables
Environment variables allow configuration settings to change depending on the environment (development, production, etc.). This is helpful for API keys, database URLs, and other sensitive information.
Use the
dotenv
package to manage environment variables. Install it with:
npm install dotenv --save
Create a
.env
file in your project root:
NODE_ENV=development API_URL=https://api.example.com
Load these variables in your
webpack.config.js
:
// webpack.config.js require('dotenv').config(); module.exports = { // Other configurations... plugins: [ new webpack.DefinePlugin({ 'process.env.NODE_ENV': JSON.stringify(process.env.NODE_ENV), 'process.env.API_URL': JSON.stringify(process.env.API_URL) }) ] };
This setup enables you to access
process.env.NODE_ENV
and process.env.API_URL
in your application.
Related Article: How to Compare Vite and Webpack for Your Project
Implementing Code Splitting
Code splitting allows you to divide your code into separate bundles that can be loaded on demand. This reduces the initial loading time of your application, improving performance.
In Webpack, you can implement code splitting using dynamic imports. For example, in your
renderer.js
, you might have:
// renderer.js const loadModule = async () => { const module = await import('./someModule.js'); module.init(); }; loadModule();
Webpack will automatically create a new bundle for
someModule.js
and load it when needed.
Using Tree Shaking to Reduce Electron App Size
Tree shaking is a technique in Webpack that eliminates dead code from your bundles. This means any code that is not used in your application will not be included in the final output, reducing the size of the application.
To enable tree shaking, ensure that your Webpack configuration has the following:
// webpack.config.js module.exports = { mode: 'production', // Enable production mode // Other configurations... };
Additionally, ensure that all modules are written in ES6 format. For example:
// myModule.js export const usedFunction = () => { /* some code */ }; export const unusedFunction = () => { /* some code */ };
Only
usedFunction
will be included in the final bundle if it is imported and used in your application.
Enabling Hot Module Replacement
Hot Module Replacement (HMR) allows you to update your application in real-time during development without needing a full reload. This provides a better development experience.
To enable HMR in Electron with Webpack, use the
webpack-dev-server
and webpack-hot-middleware
. First, install the required packages:
npm install webpack-dev-server webpack-hot-middleware --save-dev
Modify your
webpack.config.js
:
const webpack = require('webpack'); module.exports = { // Other configurations... plugins: [ new webpack.HotModuleReplacementPlugin(), ], };
In your
main.js
, you can set up HMR like this:
if (module.hot) { module.hot.accept(); }
This allows the application to reflect changes instantly in the renderer process.
Using a Development Server
A development server provides a local environment for testing your application. It serves your app and automatically reloads when changes are made.
To set this up with Webpack, modify your
webpack.config.js
to include a dev server configuration:
devServer: { contentBase: path.join(__dirname, 'dist'), hot: true, // Enable HMR port: 3000, // Port for the dev server },
You can then run the development server using:
npx webpack serve
This will start the server and serve your application at
http://localhost:3000
.
Related Article: How to Fix Angular Webpack Plugin Initialization Error
Recommended Loaders for Electron Projects
Loaders in Webpack transform files into modules as they are added to your application. For Electron projects, several loaders are commonly used:
1. babel-loader: Transpiles modern JavaScript to a version compatible with older browsers.
Install it with:
npm install babel-loader @babel/core @babel/preset-env --save-dev
Configure it in
webpack.config.js
:
module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env'] } } } ] }
2. css-loader: Allows you to import CSS files directly into your JavaScript.
Install it with:
npm install css-loader style-loader --save-dev
Configure it in
webpack.config.js
:
module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'] } ] }
3. file-loader: Helps to manage image and font files by copying them to the output directory.
Install it with:
npm install file-loader --save-dev
Configure it:
module: { rules: [ { test: /\.(png|jpg|gif|svg|eot|ttf|woff|woff2)$/, use: { loader: 'file-loader', options: { name: '[path][name].[ext]', outputPath: 'assets/', } } } ] }
These loaders help to manage different types of assets within your Electron application.
Essential Plugins
Plugins extend the functionality of Webpack and are crucial in optimizing Electron applications. Here are some essential plugins:
1. HtmlWebpackPlugin: Simplifies the creation of HTML files that include your bundles.
Install it with:
npm install html-webpack-plugin --save-dev
Configure it:
const HtmlWebpackPlugin = require('html-webpack-plugin'); plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', // Template for your HTML file filename: 'index.html', // Output file name }), ],
2. CleanWebpackPlugin: Cleans the output directory before each build.
Install it with:
npm install clean-webpack-plugin --save-dev
Configure it:
const { CleanWebpackPlugin } = require('clean-webpack-plugin'); plugins: [ new CleanWebpackPlugin(), ],
3. MiniCssExtractPlugin: Extracts CSS into separate files, which is useful for production.
Install it with:
npm install mini-css-extract-plugin --save-dev
Configure it:
const MiniCssExtractPlugin = require('mini-css-extract-plugin'); plugins: [ new MiniCssExtractPlugin({ filename: '[name].css', chunkFilename: '[id].css', }), ],
These plugins help streamline the build process and improve application performance.
Handling Static Assets
Handling static assets like images, fonts, and other media files is essential in any Electron application. Loaders play a key role in this process.
For instance, to manage images and fonts, you can leverage the
file-loader
as mentioned previously. This loader enables you to import assets directly into your JavaScript files.
In your
renderer.js
, you might have:
import logo from './assets/logo.png'; const img = document.createElement('img'); img.src = logo; document.body.appendChild(img);
Webpack processes the image and places it in the output directory, allowing you to reference it easily in your code.
Optimizing Size with Asset Management Techniques
Optimizing the size of your Electron application is crucial for performance and user experience. Techniques such as image optimization, compression, and minification can significantly reduce the size of your final bundle.
1. Image Optimization: Use tools like
image-webpack-loader
to optimize images during the build process. Install it with:
npm install image-webpack-loader --save-dev
Configure it in
webpack.config.js
:
module: { rules: [ { test: /\.(png|jpg|gif)$/, use: [ { loader: 'url-loader', options: { limit: 8192, // In bytes }, }, { loader: 'image-webpack-loader', options: { mozjpeg: { progressive: true, quality: 65, }, // Other optimization options... }, }, ], }, ], }
2. Compression: Use the
compression-webpack-plugin
to compress your JavaScript bundles.
Install it with:
npm install compression-webpack-plugin --save-dev
Configure it:
const CompressionWebpackPlugin = require('compression-webpack-plugin'); plugins: [ new CompressionWebpackPlugin({ algorithm: 'gzip', test: /\.js$|\.css$|\.html$/, threshold: 10240, minRatio: 0.8, }), ],
These techniques help reduce the overall application size, leading to faster loading times and better performance.
Related Article: How To Define A Plugin In Webpack
Module Federation
Module Federation allows multiple Webpack builds to share code, enabling greater flexibility and reusability. This is particularly useful in large applications or micro-frontend architectures.
To set up Module Federation, you will need to configure your
webpack.config.js
as follows:
const ModuleFederationPlugin = require('webpack/lib/container/ModuleFederationPlugin'); plugins: [ new ModuleFederationPlugin({ name: 'app1', filename: 'remoteEntry.js', remotes: { app2: 'app2@http://localhost:3001/remoteEntry.js', }, exposes: { './Component': './src/Component', }, shared: { react: { singleton: true, eager: true }, 'react-dom': { singleton: true, eager: true }, }, }), ],
In this example,
app1
can consume components from app2
, enhancing modularity and maintaining a clean architecture.
Best Practices for Configuring Electron with Bundling
Following best practices ensures a smooth development experience and a high-quality application. Here are some best practices:
1. Keep Processes Separate: Maintain distinct entry points for the main and renderer processes to keep concerns separated.
2. Use Production Mode: Always set Webpack to production mode for builds to enable optimizations like minification and tree shaking.
3. Version Control: Use version control for your configuration files to track changes and revert if necessary.
4. Regular Updates: Keep Webpack and its plugins up to date to benefit from the latest features and security patches.
5. Testing: Implement automated testing to ensure that changes do not break existing functionality.
Configuring Production Builds for Electron Applications
Configuring production builds is essential for deploying a stable version of your Electron application. Use the following configurations in your
webpack.config.js
:
const TerserPlugin = require('terser-webpack-plugin'); module.exports = { mode: 'production', optimization: { minimize: true, minimizer: [new TerserPlugin()], }, output: { path: path.resolve(__dirname, 'build'), filename: '[name].[contenthash].js', }, };
In production builds, ensure that the application is minified and optimized for performance. Use the
TerserPlugin
to minimize JavaScript files, and utilize content hashes in filenames for better caching strategies.
Following these guidelines will help create a robust Electron application that is optimized for performance and scalability.
Additional Resources
- Setting Up Webpack with Electron