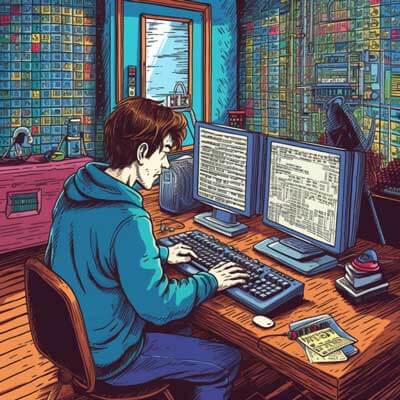
Table of Contents
Configuring SVGR with Webpack allows developers to transform SVG images into React components seamlessly. This integration streamlines the process of using SVGs in React applications, making them more manageable and reusable. The configuration generally involves adding a rule in the Webpack configuration file to handle SVG files with SVGR.
What is SVGR?
SVGR is a tool that transforms SVG files into React components. The main purpose is to allow developers to import SVGs directly into their React applications as components, which can then be styled and manipulated using JavaScript and CSS. Instead of using <img>
tags for SVGs, SVGR enables a more React-friendly approach, making it easier to handle SVGs as part of the component architecture.
Related Article: How to Configure Webpack for Expo Projects
Converting SVG to React Components with SVGR
To convert SVG files into React components, follow these steps:
1. Install SVGR and Webpack dependencies:
npm install @svgr/webpack --save-dev
2. Update your Webpack configuration to include SVGR. Open your webpack.config.js
and add the following rule:
module.exports = { module: { rules: [ { test: /\.svg$/, use: [ '@svgr/webpack', 'url-loader', // or 'file-loader' depending on your need ], }, ], }, };
3. Import the SVG in your React component:
import MyIcon from './my-icon.svg'; const App = () => ( <div> <MyIcon /> </div> ); export default App;
This setup allows you to use SVG files as React components.
Advantages of Using SVGR in Projects
SVGR offers several advantages for React projects:
- Component-based approach: SVGs can be treated as React components, allowing for better reusability and maintainability.
- Props handling: You can pass props to SVG components, enabling dynamic styling and behavior.
- Tree-shaking: Only the SVG components used in the application are included in the final bundle, helping reduce file size.
- Built-in optimization: SVGR optimizes the SVG files during the conversion process, ensuring better performance.
Optimizing SVG Files Using SVGR
Optimizing SVG files is crucial for performance. SVGR performs some optimizations by default, but further optimization can be achieved by configuring SVGR options. For example, you can enable the svgo
feature:
1. Create an SVGR configuration file, .svgrrc.js
:
module.exports = { svgo: { plugins: [ { removeViewBox: false, }, ], }, };
2. This file can contain various SVGO options to customize the optimization process based on your needs.
Related Article: How to Use Webpack in Your Projects
SVGR Compatibility with TypeScript
SVGR is compatible with TypeScript projects. To use SVG files as components in a TypeScript environment, you need to declare the module for SVG files. Create a TypeScript declaration file, svg.d.ts
, in your project:
declare module '*.svg' { import React from 'react'; const ReactComponent: React.FC<React.SVGProps<SVGSVGElement>>; export default ReactComponent; }
This declaration ensures TypeScript recognizes SVG imports as React components, allowing for seamless integration.
Common Issues Encountered with SVGR and Webpack
Some common issues when using SVGR with Webpack include:
- Incorrect Webpack configuration: If the rule for SVGs is not set correctly, SVG files may not be processed by SVGR.
- TypeScript errors: If TypeScript is not configured to recognize SVG files, it may throw errors on import.
- Missing SVGO plugins: If certain optimizations do not work, ensure the required SVGO plugins are installed and configured correctly.
Customizing SVGR Configuration for Specific Needs
Customization of SVGR is possible through various options. These options can be set in the .svgrrc.js
file or directly in the Webpack configuration. For example, if you want to modify the default behavior for styling, you can add options like:
module.exports = { replaceAttrValues: { '#000': 'currentColor', // Change black color to currentColor }, };
This allows for greater control over how SVGs are rendered and styled.
Using SVGR with Other Bundlers
SVGR is not limited to Webpack. It can also be configured with other bundlers like Rollup or Parcel. For example, with Rollup, you would install the @svgr/rollup
:
npm install @svgr/rollup --save-dev
Then configure it in your rollup.config.js
:
import svgr from '@svgr/rollup'; export default { plugins: [ svgr(), ], };
This allows for similar functionality in handling SVGs as React components.
Related Article: How to Use Webpack Tree Shaking for Smaller Bundles
Alternatives
While SVGR is a popular choice, there are alternatives for managing SVGs in React applications:
- React SVG: A library that allows SVGs to be imported as components without additional configuration.
- SVG Sprite: A technique for combining multiple SVGs into a single file to reduce HTTP requests.
- Direct SVG Imports: Importing SVGs as strings and using dangerouslySetInnerHTML
.
Each of these alternatives has its use cases, depending on the project requirements.
Integrating SVGR with Babel
Integrating SVGR with Babel can enhance the functionality of your SVG components. To do this, you can use the @svgr/babel-plugin
:
1. Install the Babel plugin:
npm install @svgr/babel-plugin --save-dev
2. Add it to your Babel configuration:
{ "plugins": [ "@svgr/babel-plugin" ] }
This setup allows you to use SVGR with Babel transformations, giving you access to additional features like JSX syntax optimization.
Using File Loader for SVG Assets in SVGR
When managing SVG assets, using the file-loader
or url-loader
alongside SVGR can be beneficial. This approach allows you to handle SVG files that are not directly converted to components. To set this up, ensure your Webpack configuration includes both loaders:
module.exports = { module: { rules: [ { test: /\.svg$/, oneOf: [ { resourceQuery: /react/, // This loader will be applied for SVGs imported as React components use: '@svgr/webpack', }, { use: 'file-loader', // This loader will handle other SVG imports }, ], }, ], }, };
This distinction allows for flexibility in how SVG files are used in the application.
Asset Management Strategies
Managing SVG assets effectively involves organizing them in a way that makes them easy to import and use across your React components. Here are some strategies:
- Folder Structure: Organize SVG files in a dedicated folder, such as src/assets/icons/
, to keep the project tidy.
- Component Indexing: Create an index file that exports all SVG components from a central location:
export { default as MyIcon } from './my-icon.svg'; export { default as AnotherIcon } from './another-icon.svg';
- Naming Conventions: Use consistent naming conventions for SVG files to simplify imports and usage in components.
Related Article: How to Configure DevServer Proxy in Webpack
JavaScript Bundling Techniques
Bundling techniques with SVGR focus on ensuring SVG components are included in the final bundle efficiently. Using tree-shaking, only the components that are imported in the code will be bundled, reducing overall file size. To achieve this, ensure that your Webpack configuration is optimized and that you are importing SVG components selectively.
Implementing CSS in JS with SVGR Components
Using CSS-in-JS libraries like Styled Components or Emotion with SVGR components is a common practice in modern React applications. You can style SVG components directly using these libraries. For example, with Styled Components:
import styled from 'styled-components'; import MyIcon from './my-icon.svg'; const StyledIcon = styled(MyIcon)` width: 50px; height: 50px; fill: red; `; const App = () => ( <div> <StyledIcon /> </div> ); export default App;
This approach gives you the flexibility to style SVGs as you would with any other React component.
Building Component Libraries
When building component libraries, SVGR can be an integral part of managing SVG assets. By converting SVGs into components, you can create a library of reusable icons and graphics. Follow these steps:
1. Organize SVG files in a designated folder.
2. Create a dedicated components folder in your library for SVG components.
3. Use an index file to export all SVG components for easy imports.
Additional Resources
- SVGR Documentation