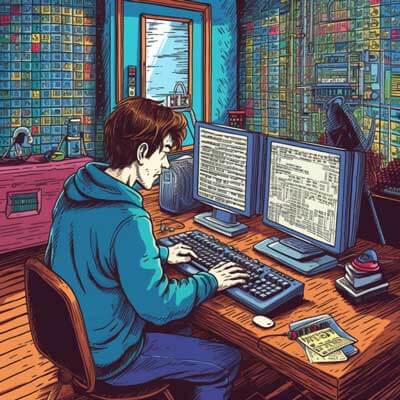
Table of Contents
Overview of Configuration for Expo Projects
Expo is a framework and platform for universal React applications. It provides developers with a set of tools for building mobile applications easily. Although Expo abstracts away many complexities, there are times when custom configurations can enhance your project. Webpack is a module bundler that can help in managing your JavaScript applications, including those built with Expo. Configuring Webpack for Expo projects enables better control over the build process, allowing for custom optimizations and enhancements.
Related Article: How to Use Webpack Manifest Plugin
Setting Up a Basic Configuration
Start by initializing a new Expo project. Use the command:
expo init my-expo-app
Navigate into your project folder:
cd my-expo-app
Next, install Webpack and necessary dependencies:
npm install --save-dev webpack webpack-cli webpack-dev-server
Create a webpack.config.js
file in your project root. A basic configuration might look like this:
// webpack.config.js const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, mode: 'development', };
This configuration specifies the entry point and output options. The mode
property determines the environment in which Webpack runs.
Entry Point Configuration Details
The entry point is where Webpack starts building the dependency graph for your application. In the configuration above, the entry point is set to ./src/index.js
. This file typically contains the root React component of your application.
If your application has multiple entry points, you can specify them as follows:
// webpack.config.js module.exports = { entry: { app: './src/index.js', admin: './src/admin.js', }, // other configurations... };
This allows you to compile separate bundles for different parts of your application.
Output Configuration Explained
The output property defines where the bundled files will be stored and how they will be named. In the example given, the output filename is bundle.js
, and it will be placed in the dist
folder, which is created in the root of your project.
You can further customize the output settings:
// webpack.config.js output: { filename: '[name].bundle.js', path: path.resolve(__dirname, 'dist'), publicPath: '/dist/', },
Using [name]
allows you to create bundles with dynamic names based on your entry points.
Related Article: How to Compare Vite and Webpack for Your Project
Working with Loaders
Loaders are transformations that are applied to the source files of your application. They allow you to preprocess files as you import or bundle them. For example, to handle JavaScript files, you need to use Babel. Install the necessary loader and presets:
npm install --save-dev babel-loader @babel/core @babel/preset-env @babel/preset-react
Next, configure the loader in your Webpack config:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.jsx?$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env', '@babel/preset-react'], }, }, }, ], }, };
This setup allows Webpack to process .js
and .jsx
files using Babel, which transpiles modern JavaScript and React code into a version that can run in most browsers.
Essential Plugins for Your Setup
Plugins extend Webpack's capabilities. Some essential plugins for Expo projects include:
1. HtmlWebpackPlugin: Generates an HTML file to serve your bundled application.
2. MiniCssExtractPlugin: Extracts CSS into separate files.
Install these plugins:
npm install --save-dev html-webpack-plugin mini-css-extract-plugin
Add them to your Webpack config:
const HtmlWebpackPlugin = require('html-webpack-plugin'); const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', }), new MiniCssExtractPlugin({ filename: '[name].css', }), ], };
This configuration generates an index.html
file in the output directory, referencing your bundled JavaScript and CSS files.
Managing Assets
Handling static assets like images and fonts is crucial in any project. Use file-loader or url-loader to manage these assets. Install file-loader:
npm install --save-dev file-loader
Add a rule for images in your Webpack configuration:
// webpack.config.js module.exports = { module: { rules: [ // other rules... { test: /\.(png|jpe?g|gif|svg)$/, loader: 'file-loader', options: { name: '[path][name].[ext]', }, }, ], }, };
This configuration will copy image files to the output directory, maintaining their original paths.
Development Mode Features and Setup
In development mode, faster builds and live reloading are essential. Use Webpack Dev Server for this purpose. Install it if you haven’t already:
npm install --save-dev webpack-dev-server
Add the following to your Webpack config:
devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000, hot: true, },
Run the server using:
npx webpack serve
This command starts a local development server on port 9000, enabling hot reloading.
Related Article: How to Choose Between Gulp and Webpack
Production Mode Optimization Techniques
For production builds, performance optimizations are necessary. Set the mode to 'production':
mode: 'production',
Webpack automatically optimizes the build by minimizing the output. You can also add specific optimizations:
optimization: { splitChunks: { chunks: 'all', }, minimize: true, },
This configuration splits the code into smaller chunks, which can be loaded as needed.
Implementing Hot Module Replacement
Hot Module Replacement (HMR) allows modules to be updated in the browser without a full reload. To enable HMR, ensure the devServer
is configured correctly, as shown earlier. Additionally, modify your entry configuration:
entry: [ 'webpack-hot-middleware/client', './src/index.js', ],
This setup allows Webpack to replace modules in the browser during development, improving the development experience.
Code Splitting Techniques for Better Performance
Code splitting allows loading parts of your application on-demand. This can be achieved using dynamic imports. Modify your import statements:
const SomeComponent = React.lazy(() => import('./SomeComponent'));
Wrap your component in a Suspense
component:
<Suspense fallback={<div>Loading...</div>}> <SomeComponent /> </Suspense>
This configuration ensures that SomeComponent
is only loaded when needed, reducing the initial bundle size.
Tree Shaking
Tree shaking is a technique for removing unused code from your final bundle. To enable tree shaking, ensure your project uses ES6 module syntax. Webpack can automatically eliminate dead code during the production build:
mode: 'production',
If you are using libraries like Lodash, import only the specific functions you need:
import debounce from 'lodash/debounce';
This practice can significantly reduce the size of your bundles.
Related Article: How to Use the Webpack CLI Option -d
Best Practices for Structuring Your Configuration File
Organizing your Webpack configuration enhances readability and maintainability. Here are some best practices:
1. Use multiple configuration files for different environments (e.g., webpack.dev.js
, webpack.prod.js
).
2. Separate common configurations into a shared file.
3. Use descriptive names for entry points and output files.
4. Group related module
rules together.
5. Comment your code to explain complex configurations.
Example of splitting configurations:
// webpack.common.js const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, module: { rules: [ // Loaders... ], }, }; // webpack.dev.js const common = require('./webpack.common.js'); module.exports = { ...common, mode: 'development', devServer: { // Dev server options... }, }; // webpack.prod.js const common = require('./webpack.common.js'); module.exports = { ...common, mode: 'production', optimization: { // Optimization options... }, };
This structure keeps the configuration organized and makes it easier to manage different environments.
Additional Resources
- Basic Webpack Configuration Guide