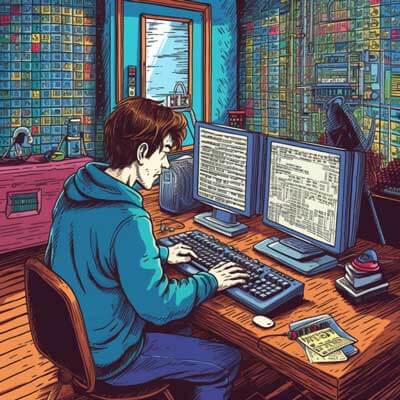
Table of Contents
Converting a string to a boolean value in JavaScript can be done using various methods. Here are two possible solutions:
Method 1: Using the Boolean() Function
The simplest way to convert a string to a boolean value in JavaScript is by using the built-in Boolean() function. This function takes a value as an argument and returns its boolean equivalent.
Example:
var str = "true"; var boolValue = Boolean(str); console.log(boolValue); // Output: true
In the example above, the string "true" is converted to the boolean value true using the Boolean() function. The resulting boolean value is then stored in the boolValue variable and printed to the console.
Related Article: Is Next.js a Frontend or a Backend Framework?
Method 2: Using Comparison Operators
Another way to convert a string to a boolean value is by using comparison operators such as == or ===. When comparing a string with a boolean value using these operators, JavaScript automatically performs type coercion and converts the string to a boolean.
Example:
var str = "false"; var boolValue = (str === "true"); console.log(boolValue); // Output: false
In the example above, the string "false" is compared with the string "true" using the === operator. Since the two values are not identical, the comparison returns false, which is stored in the boolValue variable and printed to the console.
Best Practices
When converting a string to a boolean in JavaScript, it is important to keep the following best practices in mind:
1. Use the Boolean() function when you want to explicitly convert a value to a boolean. This approach is more readable and avoids any confusion.
2. Be cautious when using comparison operators to convert strings to booleans. Make sure to use the appropriate operator (== or ===) based on your requirements. Remember that the === operator performs strict equality comparison, while the == operator performs loose equality comparison with type coercion.
3. Always test your code with different inputs to ensure that the conversion is working as expected. This helps in identifying any edge cases or unexpected behavior.
Alternative Ideas
Apart from the methods mentioned above, you can also convert a string to a boolean using other techniques such as:
- Using a ternary operator to conditionally assign a boolean value based on the string's content.
- Using regular expressions to match specific patterns in the string and determine the boolean value accordingly.
However, the methods described in this answer are the most straightforward and commonly used approaches for converting a string to a boolean in JavaScript.
Overall, converting a string to a boolean in JavaScript is a simple task that can be accomplished using built-in functions or comparison operators. By following best practices and choosing the appropriate method based on your requirements, you can ensure accurate boolean conversions in your JavaScript code.