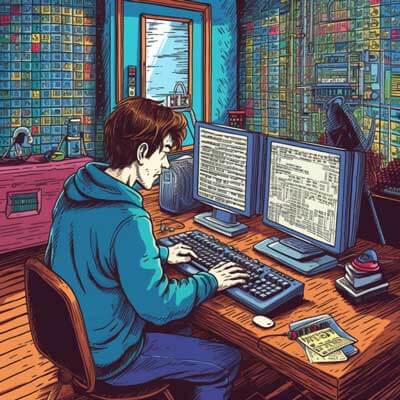
Table of Contents
In Python, you can convert a string to a boolean value using the built-in bool()
function. The bool()
function takes an argument and returns True
or False
based on the truthiness of the argument. Here are a few ways to convert a string to a boolean in Python:
Using the bool() function
You can use the bool()
function to convert a string to a boolean value. The bool()
function returns True
for non-empty strings and False
for empty strings. Here's an example:
string = "True" boolean = bool(string) print(boolean) # Output: True
In this example, the string "True"
is converted to the boolean value True
using the bool()
function.
Related Article: How to Implement Data Science and Data Engineering Projects with Python
Using a dictionary
Another way to convert a string to a boolean value is by using a dictionary. You can define a dictionary that maps specific strings to their corresponding boolean values. Here's an example:
string = "yes" boolean_dict = {"true": True, "yes": True, "false": False, "no": False} boolean = boolean_dict.get(string.lower()) print(boolean) # Output: True
In this example, the string "yes"
is converted to the boolean value True
using a dictionary lookup.
Best Practices
Related Article: Python Programming for Kids
When converting a string to a boolean in Python, it's important to consider the following best practices:
- Handle case-insensitivity: Since strings in Python are case-sensitive, it's a good practice to convert the string to lowercase before performing the conversion. This ensures that variations in case don't affect the conversion outcome.
- Handle invalid values: If the input string does not match any expected values, it's important to handle this case and provide a default value or raise an exception.
- Use explicit conversions: While Python's bool()
function can automatically convert certain string values to boolean, it's generally a good practice to use explicit conversions using custom logic or dictionaries. This allows for better control over the conversion process and makes the code more readable.