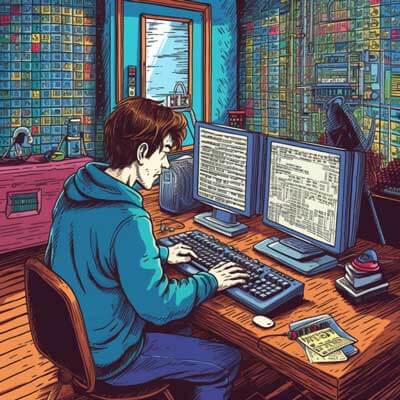
Table of Contents
Converting a string to a dictionary is a common operation in Python when working with data. Fortunately, Python provides several ways to accomplish this task. In this article, we will explore various methods to convert a string to a dictionary in Python.
Method 1: Using the eval() function
One way to convert a string to a dictionary is by using the eval() function. The eval() function evaluates an expression and returns the result. In this case, we can pass the string representation of a dictionary to the eval() function, and it will return the corresponding dictionary object.
Here's an example:
string_dict = '{"name": "John", "age": 30, "city": "New York"}' dictionary = eval(string_dict) print(dictionary)
Output:
{'name': 'John', 'age': 30, 'city': 'New York'}
It is important to note that using the eval() function can be risky if you are working with untrusted or user-generated strings. The eval() function can execute arbitrary code, so ensure that you only use it with trusted sources.
Related Article: How To Use Ternary Operator In Python
Method 2: Using the json module
Another way to convert a string to a dictionary is by using the json module. The json module provides functions for encoding and decoding JSON data. We can use the json.loads() function to parse a JSON-formatted string into a dictionary.
Here's an example:
import json string_dict = '{"name": "John", "age": 30, "city": "New York"}' dictionary = json.loads(string_dict) print(dictionary)
Output:
{'name': 'John', 'age': 30, 'city': 'New York'}
The json.loads() function can handle more complex JSON strings, including nested dictionaries and arrays. It is a safer alternative to using the eval() function because it only parses JSON data and does not execute arbitrary code.
Method 3: Using the ast module
The ast module provides a safe way to evaluate and parse Python expressions. We can use the ast.literal_eval() function to convert a string to a dictionary. The ast.literal_eval() function only evaluates a subset of Python expressions, making it safer than the eval() function.
Here's an example:
import ast string_dict = '{"name": "John", "age": 30, "city": "New York"}' dictionary = ast.literal_eval(string_dict) print(dictionary)
Output:
{'name': 'John', 'age': 30, 'city': 'New York'}
The ast.literal_eval() function can handle simple data types like strings, numbers, booleans, lists, and dictionaries. However, it cannot evaluate more complex expressions or code.
Method 4: Manually parsing the string
If you have a specific string format that does not match valid JSON or Python syntax, you can manually parse the string to convert it to a dictionary. This method requires you to understand the structure of the string and extract the key-value pairs.
Here's an example:
string_dict = "name=John,age=30,city=New York" dictionary = {} for pair in string_dict.split(","): key, value = pair.split("=") dictionary[key] = value print(dictionary)
Output:
{'name': 'John', 'age': '30', 'city': 'New York'}
This method works well if you have control over the string format and it is consistent. However, it can be error-prone and may require additional error handling if the string format is not guaranteed.