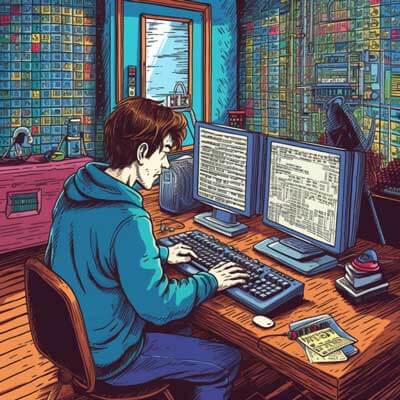
Table of Contents
To convert a Unix timestamp to time in Javascript, you can use the built-in Date
object and its methods. Here are two possible ways to achieve this:
Using the toLocaleString()
method
The toLocaleString()
method of the Date
object returns a string representation of the date and time based on the host system's current locale settings. By passing the Unix timestamp multiplied by 1000 (to convert it to milliseconds) as an argument to the Date
constructor, you can create a Date
object representing that timestamp. You can then use the toLocaleString()
method to obtain the formatted date and time string.
const unixTimestamp = 1622771526; const date = new Date(unixTimestamp * 1000); const timeString = date.toLocaleString(); console.log(timeString);
This will output the date and time string in the format determined by the host system's locale settings, such as "6/4/2021, 1:52:06 PM" in the US.
Related Article: Integrating React Components with Vanilla JavaScript
Using the toLocaleTimeString()
method
If you only need the time portion of the Unix timestamp, you can use the toLocaleTimeString()
method instead. This method returns a string representing the time portion of the Date
object based on the host system's current locale settings.
const unixTimestamp = 1622771526; const date = new Date(unixTimestamp * 1000); const timeString = date.toLocaleTimeString(); console.log(timeString);
This will output the time string in the format determined by the host system's locale settings, such as "1:52:06 PM" in the US.
Alternative Approach: Using the Intl.DateTimeFormat
object
Another alternative to convert a Unix timestamp to time in Javascript is by using the Intl.DateTimeFormat
object. This object provides a way to format dates and times based on the specified locale.
const unixTimestamp = 1622771526; const date = new Date(unixTimestamp * 1000); const timeFormatter = new Intl.DateTimeFormat(undefined, { timeStyle: 'medium' }); const timeString = timeFormatter.format(date); console.log(timeString);
The Intl.DateTimeFormat
object is initialized with the desired locale (in this case, undefined
to use the default locale) and an options object specifying the desired time style ('medium'
in this example). The format()
method is then used to obtain the formatted time string.
This approach gives you more control over the formatting options, allowing you to customize the output based on your specific requirements.
Best Practices
When converting a Unix timestamp to time in Javascript, keep the following best practices in mind:
1. Ensure that the Unix timestamp is in seconds and not milliseconds. If you have a Unix timestamp in milliseconds, divide it by 1000 to convert it to seconds before performing the conversion.
2. If you need to perform multiple conversions or formatting operations, consider creating a helper function to encapsulate the logic. This can improve code readability and reusability.
3. Be aware of the limitations of date and time formatting in different locales. Different locales may have different conventions for date and time representation, including the order of components, separators, and formatting styles. Test your code with different locales to ensure it works as expected.
4. When dealing with time zones, be mindful of the fact that Unix timestamps represent a point in time relative to Coordinated Universal Time (UTC). If you need to display the time in a specific time zone, you may need to adjust the date object accordingly.