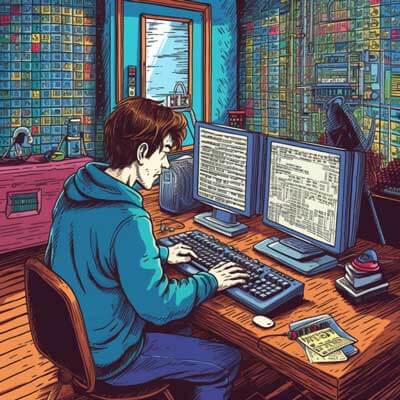
Table of Contents
Converting an object to a string in JavaScript can be done in several ways. In this guide, we will explore different methods to accomplish this task.
Method 1: Using the JSON.stringify() method
One of the easiest and most common ways to convert an object to a string is by using the JSON.stringify() method. This method takes an object as input and returns a string representation of the object.
Here's an example:
const obj = { name: 'John', age: 25, hobbies: ['reading', 'coding', 'gaming'] }; const objAsString = JSON.stringify(obj); console.log(objAsString); // Output: {"name":"John","age":25,"hobbies":["reading","coding","gaming"]}
In the above example, we have an object obj
that contains a name, age, and hobbies. We use the JSON.stringify() method to convert the object to a string and store it in the objAsString
variable. Finally, we log the objAsString
variable to the console, which displays the string representation of the object.
Related Article: Integrating HTMX with Javascript Frameworks
Method 2: Using the toString() method
Another way to convert an object to a string is by using the toString() method. This method is available on many built-in JavaScript objects, including Date, Array, and Function.
Here's an example:
const date = new Date(); const dateAsString = date.toString(); console.log(dateAsString); // Output: Tue Nov 23 2021 13:45:29 GMT+0000 (Coordinated Universal Time)
In the above example, we create a new Date object date
and then call the toString() method on it. The toString() method returns a string representation of the date object, including the date, time, and timezone information.
It's important to note that the toString() method may not always provide a meaningful string representation for custom objects or objects that don't have a built-in toString() method. In such cases, the method may return a default string representation that is not very useful.
Method 3: Implementing a custom toString() method
If you want more control over the string representation of an object, you can implement a custom toString() method for your object. This allows you to define how the object should be converted to a string.
Here's an example:
class Person { constructor(name, age) { this.name = name; this.age = age; } toString() { return `Person: ${this.name}, Age: ${this.age}`; } } const person = new Person('John', 25); const personAsString = person.toString(); console.log(personAsString); // Output: Person: John, Age: 25
In the above example, we define a Person class with a constructor that takes a name and age. We also implement a custom toString() method for the Person class that returns a formatted string representation of the object.
Method 4: Using the String() constructor
The String() constructor in JavaScript can also be used to convert an object to a string. This constructor takes any value as input and returns a string representation of that value.
Here's an example:
const num = 42; const numAsString = String(num); console.log(numAsString); // Output: "42"
In the above example, we have a number variable num
that holds the value 42. By using the String() constructor, we convert the number to a string and store it in the numAsString
variable. Finally, we log the numAsString
variable to the console, which displays the string representation of the number.
It's important to note that using the String() constructor may not always produce the desired string representation for complex objects. In such cases, it's recommended to use the JSON.stringify() method or implement a custom toString() method, as mentioned in the previous methods.