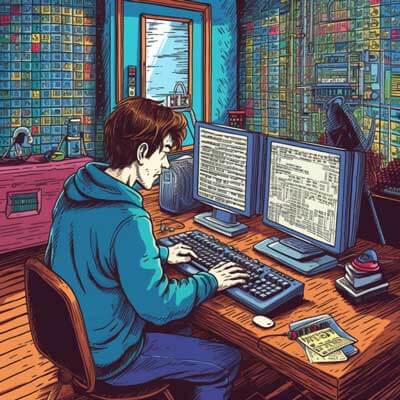
Table of Contents
To convert a date to a timestamp in JavaScript, you can use the getTime()
method of the Date
object or the valueOf()
method. These methods return the number of milliseconds since January 1, 1970, 00:00:00 UTC. To convert this value to a timestamp, you can divide it by 1000 to get the number of seconds.
Here are two possible ways to convert a date to a timestamp in JavaScript:
Using the getTime() method
You can use the getTime()
method of the Date
object to get the timestamp. Here's an example:
const date = new Date(); const timestamp = date.getTime() / 1000; console.log(timestamp);
In this example, we create a new Date
object using the current date and time. We then use the getTime()
method to get the timestamp in milliseconds. Finally, we divide the timestamp by 1000 to get the number of seconds.
Related Article: How To Generate Random String Characters In Javascript
Using the valueOf() method
Alternatively, you can use the valueOf()
method of the Date
object to get the timestamp. Here's an example:
const date = new Date(); const timestamp = date.valueOf() / 1000; console.log(timestamp);
In this example, we create a new Date
object using the current date and time. We then use the valueOf()
method to get the timestamp in milliseconds. Finally, we divide the timestamp by 1000 to get the number of seconds.
It's important to note that both the getTime()
and valueOf()
methods return the timestamp in milliseconds. If you need the timestamp in seconds, you need to divide the value by 1000.
Best Practices
Related Article: How to Remove a Specific Item from an Array in JavaScript
When converting a date to a timestamp in JavaScript, here are some best practices to keep in mind:
- Make sure you have a valid Date
object before converting it to a timestamp. If you're working with user input or data from an API, validate the input to ensure it represents a valid date.
- Consider using a library like Moment.js or date-fns for more advanced date and time manipulation. These libraries provide additional functionality and make working with dates in JavaScript easier.
- If you need to work with timezones, be aware that JavaScript's built-in Date
object uses the local timezone of the user's device. Consider using a library like Moment.js with timezone support or the toLocaleString()
method to handle timezones correctly.
- Be mindful of the units you're working with. JavaScript's Date
object returns timestamps in milliseconds, so you may need to convert them to seconds or another unit if required.
Overall, converting a date to a timestamp in JavaScript is a straightforward process using the getTime()
or valueOf()
methods of the Date
object. By following best practices and considering the requirements of your application, you can ensure accurate and reliable timestamp conversions.