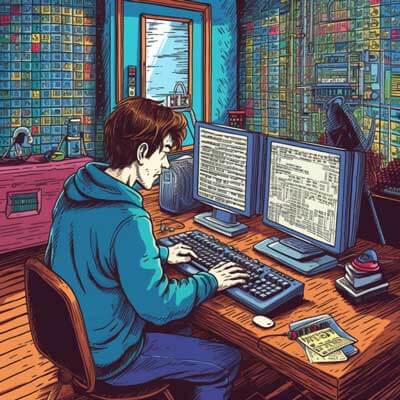
Table of Contents
There are several ways to convert a Python datetime object to a string representation of a date. In this answer, we will explore two common methods: using the strftime() method and the str() function.
Using the strftime() Method
The strftime() method is a useful tool in Python's datetime module that allows you to format datetime objects into string representations based on a specified format string. Here's how you can use it to convert a datetime object to a string of date:
import datetime # Create a datetime object now = datetime.datetime.now() # Format the datetime object to a string of date formatted_date = now.strftime("%Y-%m-%d") # Print the formatted date print(formatted_date)
Output:
2022-06-28
In the code snippet above, we import the datetime module and create a datetime object called now
, which represents the current date and time. We then use the strftime()
method on the now
object, passing in the format string "%Y-%m-%d"
, which specifies the desired format for the date. The %Y
represents the four-digit year, %m
represents the month with leading zeros, and %d
represents the day of the month with leading zeros. The strftime()
method returns a formatted string of the date, which we assign to the variable formatted_date
and print it out.
It's important to note that the strftime()
method supports a wide range of format codes, allowing you to customize the output according to your needs. Here are some common format codes for date formatting:
- %Y
: Four-digit year
- %y
: Two-digit year
- %m
: Month with leading zeros
- %b
: Abbreviated month name
- %B
: Full month name
- %d
: Day of the month with leading zeros
- %A
: Full weekday name
- %a
: Abbreviated weekday name
For a complete list of format codes, you can refer to the official Python documentation: strftime() and strptime() Behavior
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
Using the str() Function
Related Article: How to Access Python Data Structures with Square Brackets
Another simple way to convert a Python datetime object to a string of date is by using the str() function. The str() function converts the datetime object to its default string representation, which includes the date and time information. To extract only the date portion, we can manipulate the string using string slicing or the split() method. Here's an example:
import datetime # Create a datetime object now = datetime.datetime.now() # Convert the datetime object to a string date_string = str(now) # Extract only the date portion formatted_date = date_string[:10] # Print the formatted date print(formatted_date)
Output:
2022-06-28
In the code snippet above, we create a datetime object called now
representing the current date and time. We then convert the datetime object to a string using the str() function and assign it to the variable date_string
. Since the default string representation of a datetime object includes both the date and time information, we use string slicing to extract only the first 10 characters, which represent the date portion. The resulting string is assigned to the variable formatted_date
and printed out.
Although using the str() function is a straightforward approach, it may not be as flexible as using the strftime() method when it comes to customizing the date format. If you require more control over the date format, it is recommended to use the strftime() method instead.