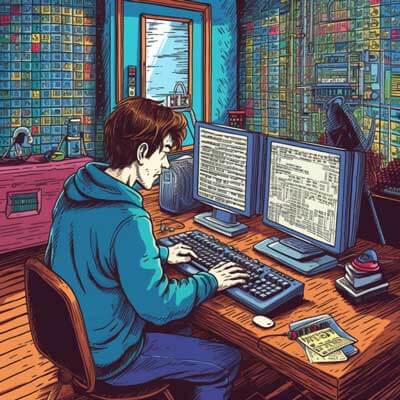
Table of Contents
To convert a string to a date in Java, you can use the SimpleDateFormat class from the java.text package. SimpleDateFormat allows you to define a pattern that matches the format of the string you want to convert. Here are two possible approaches:
Approach 1: Using SimpleDateFormat
1. Create an instance of SimpleDateFormat and specify the desired date format pattern. The pattern consists of various letters and symbols that represent different parts of the date and time. For example, "dd-MM-yyyy" represents a date format with the day, month, and year separated by hyphens.
2. Use the format() method of SimpleDateFormat to parse the string and convert it to a Date object. The format() method takes the string as input and returns a formatted date.
3. Handle any exceptions that may be thrown by the SimpleDateFormat class. The parse() method can throw a ParseException if the string does not match the specified format.
Example:
import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; public class StringToDateExample { public static void main(String[] args) { String dateString = "01-01-2022"; String pattern = "dd-MM-yyyy"; SimpleDateFormat dateFormat = new SimpleDateFormat(pattern); try { Date date = dateFormat.parse(dateString); System.out.println(date); } catch (ParseException e) { e.printStackTrace(); } } }
Output:
Sat Jan 01 00:00:00 GMT 2022
Related Article: How to Implement Recursion in Java
Approach 2: Using DateTimeFormatter (Java 8 and above)
Starting from Java 8, you can also use the DateTimeFormatter class from the java.time.format package to convert a string to a date. DateTimeFormatter is part of the new date and time API introduced in Java 8.
1. Create an instance of DateTimeFormatter and specify the desired date format pattern using the predefined patterns or a custom pattern. For example, "dd-MM-yyyy" represents a date format with the day, month, and year separated by hyphens.
2. Use the parse() method of DateTimeFormatter to parse the string and convert it to a LocalDate, LocalDateTime, or ZonedDateTime object, depending on your needs.
3. Handle any exceptions that may be thrown by the parse() method. It can throw a DateTimeParseException if the string does not match the specified format.
Example:
import java.time.LocalDate; import java.time.format.DateTimeFormatter; import java.time.format.DateTimeParseException; public class StringToDateExample { public static void main(String[] args) { String dateString = "01-01-2022"; String pattern = "dd-MM-yyyy"; DateTimeFormatter formatter = DateTimeFormatter.ofPattern(pattern); try { LocalDate date = LocalDate.parse(dateString, formatter); System.out.println(date); } catch (DateTimeParseException e) { e.printStackTrace(); } } }
Output:
2022-01-01
Best Practices
Related Article: Java OOP Tutorial
- When specifying the date format pattern, make sure it matches the format of the string you want to convert. Otherwise, the conversion may fail, and you may get unexpected results.
- Be aware of the case sensitivity of the characters in the format pattern. For example, "MM" represents the month in uppercase, while "mm" represents the minutes in lowercase.
- Consider using try-with-resources when working with SimpleDateFormat or DateTimeFormatter to automatically close the resources after use. For example:
try (SimpleDateFormat dateFormat = new SimpleDateFormat(pattern)) { // Use the dateFormat instance here } catch (ParseException e) { e.printStackTrace(); }
- If you need to perform date calculations or manipulate the date further, consider using the java.time.LocalDate, java.time.LocalDateTime, or java.time.ZonedDateTime classes from the new date and time API introduced in Java 8. These classes provide more flexibility and improved performance compared to the older java.util.Date and java.util.Calendar classes.
Overall, converting a string to a date in Java involves creating an instance of SimpleDateFormat or DateTimeFormatter, specifying the desired format pattern, and using the parse() method to convert the string to a Date or LocalDate object. Remember to handle any exceptions that may be thrown during the conversion process.
For more information, you can refer to the official Java documentation on SimpleDateFormat (https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/text/SimpleDateFormat.html) and DateTimeFormatter (https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/time/format/DateTimeFormatter.html).