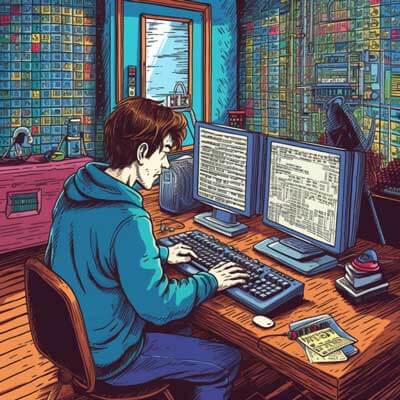
Table of Contents
Creating a two-dimensional array in JavaScript allows you to store and manipulate data in a structured manner. In this guide, we will explore different ways to create a two-dimensional array and cover some best practices.
Method 1: Using Array Literal Syntax
One of the simplest ways to create a two-dimensional array in JavaScript is by using the array literal syntax. This method involves defining an array and initializing it with nested arrays.
Here's an example of creating a two-dimensional array using the array literal syntax:
const twoDimArray = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ];
In the above example, we have created a two-dimensional array with three nested arrays, each containing three elements.
Related Article: How to Check If a String is a Valid Number in JavaScript
Method 2: Using a Loop to Initialize the Array
Another approach to creating a two-dimensional array is by using a loop to initialize the array elements. This method is useful when you want to dynamically create the array based on certain conditions or data.
Here's an example of creating a two-dimensional array using a loop:
const rows = 3; const cols = 3; const twoDimArray = []; for (let i = 0; i < rows; i++) { const row = []; for (let j = 0; j < cols; j++) { row.push(i * cols + j + 1); } twoDimArray.push(row); }
In the above example, we use two nested loops to iterate over the rows and columns of the array. We create a new array for each row and push it into the main array.
Accessing Elements in a Two-Dimensional Array
To access elements in a two-dimensional array, you need to specify both the row and column indices. Here's how you can access and modify elements in a two-dimensional array:
const twoDimArray = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; // Accessing an element const element = twoDimArray[rowIndex][colIndex]; // Modifying an element twoDimArray[rowIndex][colIndex] = newValue;
Best Practices and Tips
- When creating a two-dimensional array, ensure that all rows have the same number of columns. Inconsistent column lengths can lead to unexpected behavior.
- Consider using meaningful variable names for better code readability. For example, using numRows
and numCols
instead of rows
and cols
can make your code more understandable.
- If you need to perform operations or manipulate data in a two-dimensional array, consider using built-in array methods like map
, reduce
, or forEach
for cleaner and more concise code.
- Be cautious when modifying elements in a two-dimensional array. Make sure to handle edge cases and check for out-of-bounds indices to avoid errors.