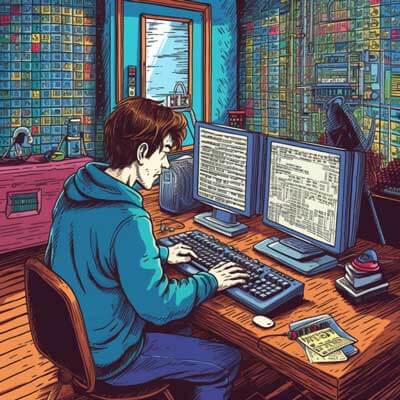
Table of Contents
Creating multiline comments in Python is a simple and useful technique that allows you to add explanatory or descriptive text to your code. While Python does not have a specific syntax for multiline comments like some other programming languages, there are a few ways to achieve the same effect.
Using Triple Quotes
One way to create multiline comments in Python is by using triple quotes. Triple quotes, either single or double, can be used to enclose multiple lines of text. These lines are treated as string literals and are ignored by the Python interpreter.
Here's an example of using triple quotes to create multiline comments:
""" This is a multiline comment that spans multiple lines. It can be used to provide explanations or documentation for your code. """
The triple quotes can be placed either at the beginning or end of the code block. It's important to note that the triple-quoted string is a valid Python object, so it will consume memory even though it is not used in the program. Therefore, it is generally recommended to use this approach for documentation purposes rather than commenting out code.
Related Article: How To Read JSON From a File In Python
Using the Hash Character
Another way to achieve multiline comments in Python is by using the hash character (#) at the beginning of each line. While the hash character is typically used to start single-line comments, it can also be used to comment out multiple lines by adding the character at the beginning of each line.
Here's an example of using the hash character to create multiline comments:
# This is a multiline comment # that spans multiple lines. # It can be used to provide # explanations or documentation # for your code.
Using the hash character method is a common practice for temporarily disabling or commenting out blocks of code during development or debugging. However, it's important to remove or uncomment the lines when the code is ready for production to ensure that the commented-out code does not affect the functionality or performance of the program.
Best Practices for Multiline Comments
Related Article: How to Sort a Pandas Dataframe by One Column in Python
When creating multiline comments in Python, it's important to follow some best practices to ensure readability and maintainability of your code:
1. Use descriptive and concise comments: Make sure your comments provide useful information without being overly verbose. Keep them concise and to the point.
2. Comment the "why," not the "what": Focus on explaining the reasons behind your code decisions rather than describing what the code does. This helps other developers understand your thought process and intentions.
3. Use proper formatting and indentation: Maintain consistent formatting and indentation in your multiline comments to improve readability. Align the comment text with the surrounding code to make it visually clear that it is a comment.
4. Update comments when code changes: Whenever you modify the code, remember to update the corresponding comments to reflect the changes accurately. Outdated comments can be misleading and cause confusion.
5. Avoid excessive commenting: While comments are important for clarity, avoid overusing them. Write code that is self-explanatory whenever possible. Only use comments when necessary to avoid cluttering your code with unnecessary text.
6. Consider using docstrings for documentation: For longer, more detailed explanations or documentation, consider using docstrings. Docstrings are string literals enclosed in triple quotes that are used to document functions, classes, and modules in Python. They serve as both comments and documentation that can be accessed using Python's built-in help()
function or through tools like Sphinx for generating documentation.