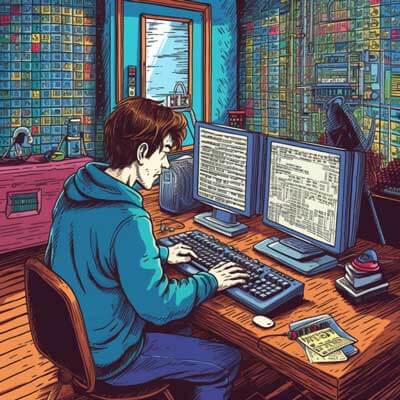
Table of Contents
When working with Python and the Pandas library, you may encounter a ValueError when trying to create a DataFrame from variables. This error typically occurs when you are using all scalar values and not passing an index. In this article, we will explore the cause of this error and discuss possible solutions.
Understanding the ValueError
The ValueError "If using all scalar values, you must pass an index" is raised when trying to create a DataFrame using all scalar values without specifying an index. A scalar value is a single value, such as an integer, float, or string, as opposed to a collection of values like a list or an array.
By default, when creating a DataFrame from scalar values, Pandas expects an index to be provided. The index provides a unique label for each row in the DataFrame, allowing for easy access and manipulation of the data.
Related Article: How to Use Python Multiprocessing
Possible Solutions
To resolve the ValueError, you have a few options:
1. Specify an Index
One way to address the ValueError is to explicitly specify an index when creating the DataFrame. You can do this by passing a list of index labels as an argument to the index
parameter of the DataFrame constructor.
Here's an example:
import pandas as pd # Create variables name = 'John' age = 30 city = 'New York' # Create DataFrame with specified index df = pd.DataFrame({'Name': name, 'Age': age, 'City': city}, index=[0]) print(df)
Output:
Name Age City 0 John 30 New York
In the above example, we create a DataFrame with the variables name
, age
, and city
. We pass these variables as a dictionary to the DataFrame constructor, and also specify the index as [0]
. This ensures that each variable corresponds to a single row in the DataFrame.
2. Use a Collection of Values
Another solution is to use a collection of values, such as lists or arrays, instead of scalar values. This way, Pandas automatically generates a default index for the DataFrame.
Here's an example:
import pandas as pd # Create variables as lists names = ['John', 'Alice', 'Bob'] ages = [30, 25, 35] cities = ['New York', 'Paris', 'London'] # Create DataFrame using lists df = pd.DataFrame({'Name': names, 'Age': ages, 'City': cities}) print(df)
Output:
Name Age City 0 John 30 New York 1 Alice 25 Paris 2 Bob 35 London
In the above example, we define the variables names
, ages
, and cities
as lists. We then pass these lists as a dictionary to the DataFrame constructor. Because we are using collections of values, Pandas automatically generates a default index for the DataFrame.
Related Article: How to Reverse a String in Python
Potential Reasons for the Error
The ValueError "If using all scalar values, you must pass an index" is typically encountered when creating a DataFrame with all scalar values and not specifying an index. There are a few potential reasons why this error may occur:
1. Forgetting to specify an index: If you are creating a DataFrame with scalar values, it is important to remember to specify an index. Without an index, Pandas cannot uniquely identify each row in the DataFrame.
2. Using scalar values instead of collections: If you are trying to create a DataFrame using scalar values, consider using collections like lists or arrays instead. This allows Pandas to automatically generate a default index for the DataFrame.
Best Practices
When creating a DataFrame from variables, it is good practice to consider the following:
1. Specify an index: If you are using all scalar values, make sure to specify an index when creating the DataFrame. This ensures that each row in the DataFrame is uniquely identified.
2. Use collections of values: Whenever possible, use collections like lists or arrays instead of scalar values. This allows Pandas to generate a default index for the DataFrame.
3. Check variable types: Make sure that the variables you are using to create the DataFrame are of the appropriate type. For example, if you are creating a DataFrame with numeric values, ensure that the variables are of type int or float.
4. Handle missing values: If your variables contain missing values, consider handling them appropriately. Pandas provides functions like fillna()
and dropna()
to handle missing values in a DataFrame.