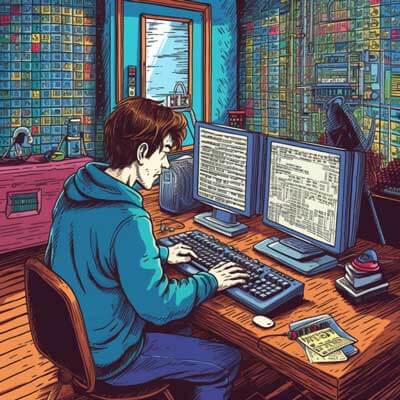
Table of Contents
Overview
Plugins extend the functionality of Webpack, allowing developers to customize the build process. They enable various features that enhance the efficiency of module bundling. Defining a plugin accurately is essential for optimizing the workflow and improving the performance of web applications.
Related Article: How to Use Webpack Manifest Plugin
What is a Plugin in Module Bundling
A plugin in module bundling serves as a tool that modifies the output of the build process. It operates on the compilation lifecycle and can handle various tasks such as optimizing assets, managing environment variables, and injecting scripts into HTML files. Plugins interact with the build process at different stages, offering developers a way to influence how resources are processed.
Differences Between Loaders and Plugins
Loaders and plugins serve different purposes. Loaders transform files before they are bundled. For instance, a loader can convert TypeScript files into JavaScript. In contrast, plugins tap into the build process and can affect the output in broader ways. They can manipulate the entire build, perform optimizations, and handle tasks that aren't specific to a single file type.
How To Create a Custom Plugin
Creating a custom plugin involves defining a class that implements the apply
method. This method allows the plugin to hook into the Webpack build process. A simple example of a custom plugin might look like this:
// my-plugin.js class MyPlugin { apply(compiler) { compiler.hooks.emit.tapAsync('MyPlugin', (compilation, callback) => { console.log('This will be logged before assets are emitted.'); callback(); }); } } module.exports = MyPlugin;
This plugin logs a message before the assets are emitted. It uses the emit
hook to interact with the compilation process.
Related Article: How To Exclude Test Files In Webpack With Esbuild
Configuring a Plugin in Your Setup
To configure a plugin, add it to the plugins
array in the Webpack configuration file. Here’s how to include the custom plugin created earlier:
// webpack.config.js const MyPlugin = require('./my-plugin'); module.exports = { // other configurations... plugins: [ new MyPlugin(), ], };
This configuration allows Webpack to utilize the custom plugin during the build process.
Benefits of Using Plugins in Your Configuration
Plugins bring numerous advantages to a Webpack configuration. They can optimize performance, automate tasks, and enhance the development experience. By using plugins, developers can reduce build times, manage assets more effectively, and streamline the overall workflow.
Popular Plugins and Their Functions
Several popular plugins are commonly used in Webpack setups. Some of these include:
- HtmlWebpackPlugin: Simplifies the creation of HTML files by automatically injecting bundled scripts.
const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ title: 'My App', }), ], };
- MiniCssExtractPlugin: Extracts CSS into separate files, improving load times.
const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { plugins: [ new MiniCssExtractPlugin(), ], };
- TerserPlugin: Minifies JavaScript files for production.
const TerserPlugin = require('terser-webpack-plugin'); module.exports = { optimization: { minimize: true, minimizer: [new TerserPlugin()], }, };
Enhancing Functionality with Multiple Plugins
Combining multiple plugins can significantly enhance the functionality of a Webpack configuration. For example, using HtmlWebpackPlugin
with MiniCssExtractPlugin
allows for optimized HTML files that reference external CSS. This combination can lead to faster load times and a better user experience.
const HtmlWebpackPlugin = require('html-webpack-plugin'); const MiniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ title: 'My App', }), new MiniCssExtractPlugin({ filename: '[name].css', }), ], };
Related Article: How to Set LibraryTarget in the Webpack Configuration
Lifecycle of a Plugin
The lifecycle of a plugin in Webpack consists of several hooks that allow it to interact with the build process. These hooks include compile
, emit
, and done
, among others. Each hook provides specific opportunities to execute code at different stages of the build.
For example, the done
hook can be used for final actions after the build completes:
class MyPlugin { apply(compiler) { compiler.hooks.done.tap('MyPlugin', (stats) => { console.log('Build is done!'); }); } }
Troubleshooting Common Plugin Issues
Common issues with plugins include misconfiguration or compatibility problems. If a plugin isn’t functioning as expected, check the following:
- Ensure the plugin is correctly installed and listed in the Webpack configuration.
- Verify that you are using compatible versions of Webpack and the plugin.
- Check the Webpack documentation for any required options or configurations specific to the plugin.
Using console logs or debugging tools can help identify where a plugin may be failing in the build process.
Asset Management with Plugins
Managing assets effectively is crucial for optimized web applications. Plugins such as CopyWebpackPlugin
allow for the easy copying of files into the output directory. This can be particularly useful for static assets like images or fonts.
const CopyWebpackPlugin = require('copy-webpack-plugin'); module.exports = { plugins: [ new CopyWebpackPlugin({ patterns: [ { from: 'src/assets', to: 'assets' }, ], }), ], };
This configuration copies files from the src/assets
directory to the assets
directory in the output.
Code Splitting and Plugins
Code splitting is a technique used to optimize loading times by breaking up code into smaller bundles. The SplitChunksPlugin
allows for automatic code splitting based on module usage. This can lead to improved performance and faster loading.
module.exports = { optimization: { splitChunks: { chunks: 'all', }, }, };
This configuration ensures that shared dependencies are split into separate chunks.
Related Article: How to Use webpack -d for Development Mode
Hot Module Replacement
Hot Module Replacement (HMR) allows developers to update code in a running application without refreshing the entire page. The webpack-dev-server
enables HMR by default. This results in a smoother development experience and faster feedback on changes.
const webpack = require('webpack'); module.exports = { devServer: { hot: true, }, plugins: [ new webpack.HotModuleReplacementPlugin(), ], };
This configuration activates HMR and injects updates into the running application.
Entry Points
Defining entry points in a Webpack configuration specifies where the bundling process starts. Plugins can interact with these entry points to modify how modules are processed. For instance, a plugin might analyze the entry points to determine shared dependencies.
module.exports = { entry: { app: './src/index.js', vendor: './src/vendor.js', }, };
This configuration defines two entry points for the application.
Output Configuration
The output configuration determines how and where the bundled files are emitted. Plugins can modify the output filename or path based on specific conditions. This allows for greater control over the final structure of the output files.
module.exports = { output: { filename: '[name].[contenthash].js', path: __dirname + '/dist', }, };
This configuration uses content hashing to ensure cache busting.
Tree Shaking and Plugin Integration
Tree shaking is a technique used to eliminate dead code from the final bundle. The TerserPlugin
can facilitate tree shaking by removing unused exports during the minification process. This results in smaller bundle sizes and improved load times.
module.exports = { optimization: { usedExports: true, minimize: true, minimizer: [new TerserPlugin()], }, };
This configuration enables tree shaking during the build process.
Related Article: How to Use the Clean Webpack Plugin
Development Server
The development server provides a local environment for testing changes in real-time. Plugins can interact with the development server to enhance the development experience. For example, logging plugin messages or displaying build status can be helpful during development.
module.exports = { devServer: { onListening: function(server) { console.log('Webpack Dev Server is running!'); }, }, };
This configuration logs a message when the development server is active.
Additional Resources
- Understanding Webpack Plugins