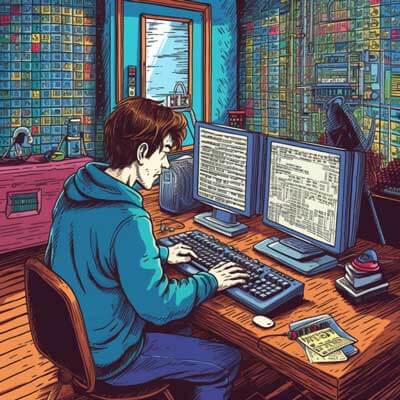
Table of Contents
Identifying the programming language used in a codebase can be essential for various reasons, such as understanding how to contribute to the project or knowing which tools and libraries to use. In many cases, this detection is based on file extensions, specific syntax patterns, or even the presence of certain keywords. For npm projects, the primary language is JavaScript, but many projects can include multiple languages, especially when they involve tooling or libraries that may be written in other languages.
package.json Structure
The package.json file serves as the heart of any npm project. This JSON file contains metadata relevant to the project, including its name, version, description, main entry point, scripts, and dependencies. The structure is hierarchical and consists of key-value pairs. Here’s a basic example of what a package.json file looks like:
{ "name": "my-project", "version": "1.0.0", "description": "A simple project", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "dependencies": { "express": "^4.17.1" } }
Each key in this JSON file provides important information about the project, which can also aid in detecting the primary programming language used.
Related Article: How to Use npm Tiny Invariant in Your Project
Key Files for Language Detection
Apart from the package.json file, there are other key files that can indicate which languages are present in a project. For instance:
- .js: Indicates JavaScript files.
- .ts: Indicates TypeScript files.
- .jsx: Indicates React JavaScript files.
- .json: Often signifies configurations that might be read by scripts in various languages.
- .py: Indicates Python files, if a project includes Python scripts.
- .rb: Indicates Ruby files, useful in some web projects.
Each of these file types serves as a signal for the language in use, and the presence of these files can help in determining the language context of the project.
How npm Detects Programming Language
When npm is executed, it primarily looks for the package.json file to gather project metadata. While npm itself is centered on JavaScript, it can detect other languages through the file types mentioned earlier. For example, if a project contains TypeScript files, npm can infer that TypeScript is also part of the environment, especially when using TypeScript-specific packages.
The detection process is not just limited to npm commands but extends to various Node.js modules that may have language-specific implementations. The presence of these modules in the dependencies section of the package.json file further indicates the programming languages utilized in the project.
Language-Specific Dependencies
Dependencies listed in the package.json file often indicate the specific programming language in use. For example, if you see dependencies like "typescript" or "ts-node", it implies that TypeScript is being used in the project. Similarly, if you find "babel" or "react", it suggests that the project uses JavaScript frameworks or platforms.
Here’s how dependencies might look in a package.json file:
"dependencies": { "express": "^4.17.1", "typescript": "^4.1.2", "react": "^17.0.1" }
This list shows that the project employs both JavaScript and TypeScript, along with React, which is a JavaScript library.
Related Article: How to Fix Deno NPM Module Is Not a Function Error
Configuring Scripts Automatically
npm scripts can automate tasks and are specified in the package.json file under the "scripts" key. The scripts can be language-specific; for example, a script to compile TypeScript code can be added as follows:
"scripts": { "build": "tsc", "start": "node index.js" }
In this case, the "build" script runs the TypeScript compiler, while the "start" script runs the main JavaScript file. These scripts help streamline the development process and make it easier to manage tasks associated with different languages.
Common Programming Languages Supported
npm primarily supports JavaScript and its variants, such as TypeScript and JSX. However, it is not limited to these languages. Other languages that may be included in npm projects are:
- Python: When using Node.js to execute Python scripts.
- Ruby: Often used alongside JavaScript in web applications.
- Java: Sometimes integrated for backend services.
The presence of any of these languages can be identified through file types and dependencies, as previously discussed.
Checking Package Language in npm
To determine the primary language used in an npm package, you can use the following command:
npm view <package-name> scripts
This command retrieves the scripts defined in the package, allowing you to see which languages might be in use based on the commands listed. Additionally, you can check the README file of the package, as it often contains information about the languages and technologies employed.
Multiple Languages in a Single Project
It is common for a project to incorporate multiple programming languages. For instance, a web application may use JavaScript for the frontend, Python for the backend, and TypeScript for type safety. The presence of different file types and dependencies in package.json can indicate this diversity.
For example, a project may have a structure like:
/my-project ├── src/ │ ├── index.js │ ├── app.py │ └── utils.ts ├── package.json └── README.md
In this structure, JavaScript, Python, and TypeScript are all being utilized, showcasing how npm can manage projects with multiple languages.
Related Article: How to List Global npm Packages
Setting Programming Language in package.json
While npm does not require you to explicitly set a programming language in the package.json file, you can indicate the primary language through the structure and dependencies. For example, if you are using TypeScript, you can include the "typescript" dependency and add related scripts:
"dependencies": { "typescript": "^4.1.2" }, "scripts": { "compile": "tsc", "start": "node dist/index.js" }
This setup clarifies that TypeScript is a significant part of the project, even if the package.json does not explicitly state the language used.
Commands for Language Detection
Using commands to detect the programming language in a project can streamline the process. Here are useful commands that help identify languages and their versions:
To check the version of the dependencies and their languages:
npm ls --depth=0
This command lists all installed packages at the top level, showing you which ones are being used and hinting at the languages involved.
To check for specific language-related packages:
npm list --prod | grep 'python\|typescript\|ruby'
This command filters the installed packages to see if any relevant languages are present in the project.
Using npm for Non-JavaScript Languages
npm is primarily designed for JavaScript projects, but it can also be used to manage non-JavaScript languages by installing compatible packages. For instance, if you are working with a Python project, you might need to manage packages that allow JavaScript to interface with Python scripts.
An example is using a package like child_process in Node.js to run Python code:
const { spawn } = require('child_process'); const pythonProcess = spawn('python', ['script.py']); pythonProcess.stdout.on('data', (data) => { console.log(`Output: ${data}`); });
This code snippet demonstrates how to run a Python script from Node.js, integrating both languages within the same project.