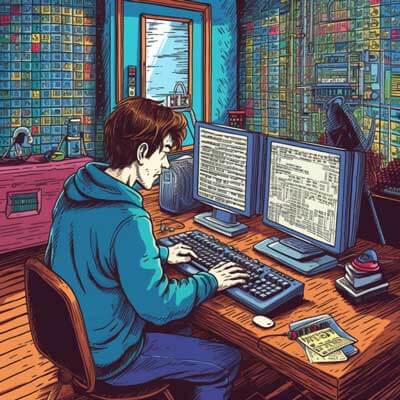
Table of Contents
To determine if a checkbox is checked in jQuery, you can use the prop()
method or the is()
method. Both methods provide a simple and efficient way to check the state of a checkbox.
Using the prop() Method
The prop()
method in jQuery allows you to get the value of a property for the first element in the set of matched elements or set one or more properties for every matched element. To determine if a checkbox is checked, you can use the prop()
method in combination with the :checked
selector.
Here's an example:
if ($('#myCheckbox').prop('checked')) { // Checkbox is checked } else { // Checkbox is not checked }
In the example above, #myCheckbox
is the ID of the checkbox element. The prop('checked')
method returns true
if the checkbox is checked, and false
otherwise.
Related Article: How To Fix 'Maximum Call Stack Size Exceeded' Error
Using the is() Method
The is()
method in jQuery allows you to check the current matched set of elements against a selector, element, or jQuery object and return true
if at least one of these elements matches the given arguments. To determine if a checkbox is checked, you can use the is()
method in combination with the :checked
selector.
Here's an example:
if ($('#myCheckbox').is(':checked')) { // Checkbox is checked } else { // Checkbox is not checked }
In the example above, #myCheckbox
is the ID of the checkbox element. The is(':checked')
method returns true
if the checkbox is checked, and false
otherwise.
Best Practices
When working with checkboxes in jQuery, it's important to follow some best practices:
1. Use proper selectors: Make sure to use unique and specific selectors to target the checkbox element. Using an ID selector (#myCheckbox
) is recommended for better performance.
2. Cache the checkbox element: If you need to check the state of a checkbox multiple times, it's a good practice to cache the checkbox element in a variable to avoid querying the DOM repeatedly.
3. Use "change" event: Instead of checking the state of the checkbox manually, consider attaching a "change" event handler to the checkbox. This allows you to perform actions whenever the checkbox state changes.
Here's an example that demonstrates these best practices:
$(document).ready(function() { var $myCheckbox = $('#myCheckbox'); $myCheckbox.on('change', function() { if ($myCheckbox.is(':checked')) { // Checkbox is checked } else { // Checkbox is not checked } }); });
In the example above, the checkbox element with the ID myCheckbox
is cached in the $myCheckbox
variable. The "change" event handler is attached to the checkbox, and whenever the checkbox state changes, the appropriate action is performed.
Alternative Ideas
While the prop()
method and the is()
method are the most common ways to determine if a checkbox is checked in jQuery, there are alternative approaches that you can consider:
1. Using the attr()
method: Instead of the prop()
method, you can use the attr()
method to get the value of the checked
attribute. However, note that the attr()
method retrieves the value as it was initially set, so it may not reflect the current state of the checkbox if it has been programmatically modified.
2. Using vanilla JavaScript: If you prefer to avoid jQuery, you can use vanilla JavaScript to determine if a checkbox is checked. You can use the checked
property of the checkbox element itself, like document.getElementById('myCheckbox').checked
.
Ultimately, the choice of approach depends on your specific requirements and the overall context of your project.