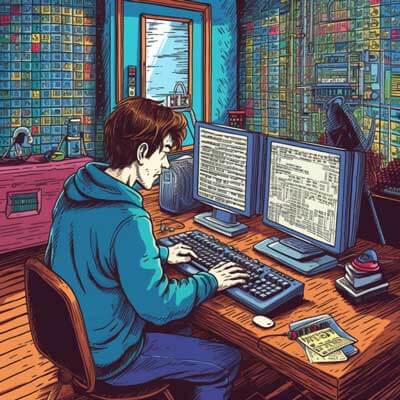
Table of Contents
JavaScript is a versatile programming language that is commonly used for web development. With the introduction of ECMAScript modules (ESM), developers now have the option to use the .mjs file extension to denote JavaScript modules. In this answer, we will explore the differences between .js and .mjs files in JavaScript.
1. File Extension
The most obvious difference between .js and .mjs files is the file extension itself. The .js extension is the traditional extension used for JavaScript files, while .mjs is used specifically for ECMAScript modules.
Related Article: How To Fix Javascript: $ Is Not Defined
2. Module Support
JavaScript modules provide a way to encapsulate and organize code into reusable components. They allow for better code organization and help to avoid naming conflicts. .mjs files are treated as ECMAScript modules by default, which means they have built-in support for module features such as import and export statements.
On the other hand, .js files are not treated as modules by default. In order to use module features in .js files, you need to explicitly enable module support by adding the "type" attribute with the value of "module" to the script tag in your HTML file, like this:
3. Script Loading
Another difference between .js and .mjs files is how they are loaded by the browser. When a .js file is loaded, it is executed immediately. This can lead to potential issues with dependencies and the order in which scripts are loaded.
On the contrary, .mjs files are loaded as ECMAScript modules, which means they are subject to the module loading algorithm. This algorithm ensures that dependencies are resolved correctly and modules are executed in the appropriate order. This makes it easier to handle complex dependency trees and avoid issues related to script loading.
4. Top-Level Variables
In JavaScript, variables declared at the top level of a script file are added to the global scope. This can lead to naming conflicts and other issues, especially when multiple scripts are included on the same page.
In .mjs files, top-level variables are not added to the global scope by default. Instead, each .mjs file has its own module scope. This helps to encapsulate variables and avoid naming conflicts between modules.
If you want to explicitly export a variable from an .mjs file and make it accessible to other modules, you can use the "export" keyword, like this:
// script.mjs export const myVariable = 'Hello, world!';
Then, in another .mjs file, you can import and use the exported variable:
// main.mjs import { myVariable } from './script.mjs'; console.log(myVariable); // Output: Hello, world!
Related Article: How To Check If a Key Exists In a Javascript Object
5. Usage in Node.js
While the .mjs file extension was initially introduced for browser-based JavaScript, it is also supported in Node.js starting from version 12. In Node.js, you can use the .mjs extension to take advantage of ECMAScript modules.
To use .mjs files in Node.js, you need to use the "--experimental-modules" flag when running your script, like this:
node --experimental-modules script.mjs
Alternatively, you can add the "type" attribute with the value of "module" to the package.json file of your Node.js project:
{ "type": "module", "scripts": { "start": "node script.mjs" } }
This allows you to run the script using the "npm start" command.