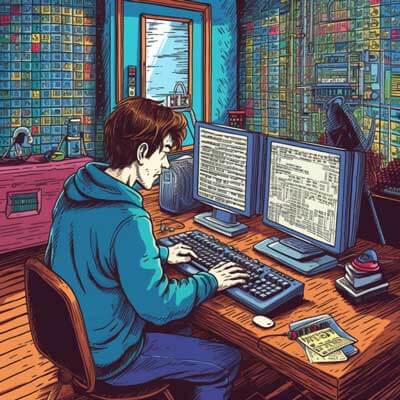
Table of Contents
To echo or print an array in PHP, you can use the print_r()
or var_dump()
functions. These functions allow you to display the contents of an array in a human-readable format. Here are the steps to echo or print an array in PHP:
Using the print_r() function
The print_r()
function is a built-in PHP function that prints the information about a variable in a more readable format. It is commonly used for debugging purposes and to display the contents of an array. Here's how you can use the print_r()
function to echo an array:
$array = array('apple', 'banana', 'orange'); print_r($array);
Output:
Array ( [0] => apple [1] => banana [2] => orange )
In the above example, the print_r()
function is used to display the contents of the $array
variable. The output shows each element of the array along with its corresponding index.
Related Article: How to Use the Now Function in PHP
Using the var_dump() function
The var_dump()
function is another built-in PHP function that is used to display information about a variable, including its type and value. It is particularly useful for debugging and understanding the structure of complex variables like arrays. Here's how you can use the var_dump()
function to echo an array:
$array = array('apple', 'banana', 'orange'); var_dump($array);
Output:
array(3) { [0]=> string(5) "apple" [1]=> string(6) "banana" [2]=> string(6) "orange" }
In the above example, the var_dump()
function is used to display information about the $array
variable. The output includes the type of the variable, the number of elements in the array, and the value of each element.
Best Practices
Related Article: How to Delete An Element From An Array In PHP
- When using print_r()
or var_dump()
, it's a good practice to wrap the output in <pre></pre>
tags. This preserves the formatting and makes the output more readable. For example:
$array = array('apple', 'banana', 'orange'); echo '<pre>'; print_r($array); echo '</pre>';
- If you want to capture the output of print_r()
or var_dump()
in a string variable instead of directly echoing it, you can use the ob_start()
and ob_get_clean()
functions. Here's an example:
$array = array('apple', 'banana', 'orange'); ob_start(); print_r($array); $output = ob_get_clean(); echo $output;
In the above example, the ob_start()
function starts output buffering, capturing all subsequent output. The print_r()
function is then called to print the array, and the output is stored in the buffer. Finally, the ob_get_clean()
function retrieves the buffer content and assigns it to the $output
variable, which can be echoed or used as needed.