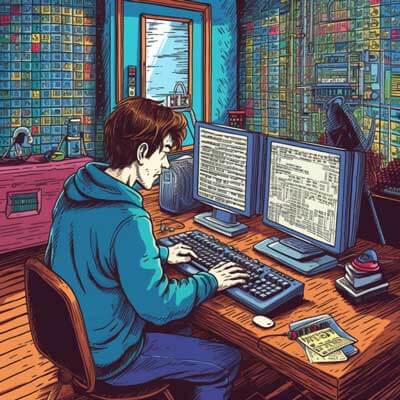
Table of Contents
To encode a string to Base64 in JavaScript, you can use the built-in btoa()
function. This function takes a string as input and returns the Base64-encoded version of the string.
Here's how you can use the btoa()
function to encode a string to Base64 in JavaScript:
const stringToEncode = "Hello, World!"; const encodedString = btoa(stringToEncode); console.log(encodedString);
This will output the Base64-encoded version of the string "Hello, World!".
Explanation
The btoa()
function stands for "binary to ASCII" and is a built-in JavaScript function that converts a binary string to a Base64-encoded ASCII string. It takes a string as input and returns the Base64-encoded version of the string.
In the example above, we first declare a variable stringToEncode
and assign it the value "Hello, World!". We then use the btoa()
function to encode the string and assign the result to a variable encodedString
. Finally, we log the encoded string to the console using console.log()
.
Related Article: AngularJS: Check if a Field Contains a MatFormFieldControl
Alternative Method: Using the Buffer
Object
Another way to encode a string to Base64 in JavaScript is by using the Buffer
object. This method is especially useful when working with Node.js, as it is a built-in module in Node.js.
Here's how you can use the Buffer
object to encode a string to Base64 in JavaScript:
const stringToEncode = "Hello, World!"; const encodedString = Buffer.from(stringToEncode).toString("base64"); console.log(encodedString);
This will also output the Base64-encoded version of the string "Hello, World!".
Explanation
The Buffer
object in Node.js provides a way to work with binary data. To encode a string to Base64 using the Buffer
object, we first create a Buffer
object from the string using Buffer.from(stringToEncode)
. We then call the toString()
method on the Buffer
object and pass "base64" as the encoding parameter to specify that we want to encode the string to Base64. The result is assigned to the variable encodedString
, and we log it to the console using console.log()
.
Best Practices
When encoding a string to Base64 in JavaScript, keep the following best practices in mind:
1. Always handle errors: The btoa()
function and Buffer.from().toString("base64")
method may throw errors if the input string contains characters that cannot be represented in Base64. Make sure to handle these errors gracefully using try-catch blocks or error handling mechanisms.
2. Consider compatibility: The btoa()
function is available in most modern browsers, but it may not be supported in older browsers or in certain environments. If you need to support older browsers or non-browser environments, using the Buffer
object method is a more reliable option.
3. Be mindful of character encoding: Base64 encoding is a binary-to-text encoding scheme, which means it can handle any binary data. However, when encoding a string, make sure the string is in the correct character encoding. The btoa()
function and Buffer.from().toString("base64")
method assume the string is in UTF-8 encoding by default.
4. Consider decoding requirements: If you need to decode the Base64-encoded string back to its original form, make sure to use the appropriate decoding method (atob()
or Buffer.from().toString("utf-8")
). Keep in mind that decoding Base64 can result in binary data, not just strings.