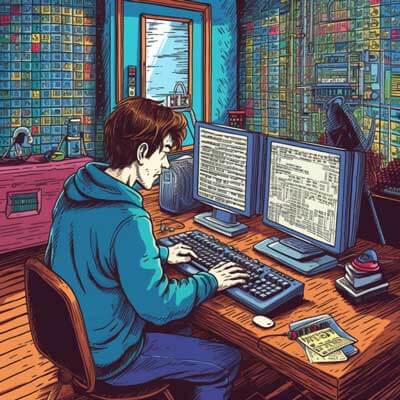
Table of Contents
Overview of the Termination Process
When writing Python programs, it's important to ensure that they terminate correctly. Properly ending a Python program involves cleaning up resources, closing open files, and releasing system resources. Failing to do so can lead to memory leaks, file corruption, and other issues. In this article, we will explore different ways to end Python programs correctly and gracefully.
Related Article: How to Use Static Methods in Python
Code Snippet for Exiting Python
To exit a Python program, you can use the exit()
function. Here's a simple code snippet that demonstrates how to exit a Python program:
import sys def main(): # Your code here sys.exit(0) if __name__ == "__main__": main()
In this example, the sys.exit(0)
statement is used to exit the program with a status code of 0. The status code indicates the reason for termination, with 0 typically indicating successful termination.
Using 'sys.exit()' to Terminate a Program
The sys.exit()
function is a widely used method to terminate Python programs. It allows you to specify an exit status code, which can be used to indicate the reason for termination. By convention, a status code of 0 indicates successful termination, while any non-zero value indicates an error or abnormal termination.
Here's an example that shows how to use sys.exit()
to terminate a Python program:
import sys def main(): # Your code here if some_condition: sys.exit(1) else: sys.exit(0) if __name__ == "__main__": main()
In this example, the program checks for a certain condition, and if it evaluates to True
, the program exits with a status code of 1. Otherwise, it exits with a status code of 0.
Terminating a Running Python Program from the Command Line
Sometimes, you may need to terminate a running Python program from the command line. This can be useful when a program is stuck in an infinite loop or taking longer to execute than expected.
To terminate a running Python program from the command line, you can use the Ctrl + C
keyboard shortcut. This sends an interrupt signal to the program, causing it to terminate gracefully. By default, the program will receive the KeyboardInterrupt
exception, allowing it to perform any necessary cleanup before exiting.
Related Article: How To Concatenate Lists In Python
Gracefully Stopping Python Script Execution
Gracefully stopping a Python script execution involves ensuring that all resources are properly cleaned up before the script exits. This includes closing open files, releasing system resources, and terminating any running processes or threads.
To gracefully stop Python script execution, you can use the try-except-finally
block to catch any exceptions and perform cleanup operations in the finally
block. Here's an example:
import time def main(): try: # Your code here time.sleep(10) # Simulating a long-running operation except Exception as e: # Handle any exceptions print("An error occurred:", str(e)) finally: # Perform cleanup operations print("Cleaning up resources...") if __name__ == "__main__": main()
In this example, the script sleeps for 10 seconds to simulate a long-running operation. If any exception occurs, it is caught in the except
block and handled accordingly. The finally
block is then executed to perform cleanup operations, such as closing files or releasing resources.
Closing the Python Shell Safely
When working with the Python shell, it's important to properly exit the shell to ensure that all resources are cleaned up and any pending operations are completed.
To safely close the Python shell, you can use the exit()
function or the Ctrl + D
keyboard shortcut. Both methods will terminate the shell and return you to the command prompt.
It's worth noting that if you have unsaved changes in the shell, you will be prompted to confirm whether you want to save the history before exiting. This allows you to save any important commands or outputs from your session.
Different Ways to End Python Programs
There are several ways to end Python programs, each with its own benefits and use cases. Some common methods include:
1. Using sys.exit()
to exit the program with a specific status code.
2. Raising an exception to terminate the program.
3. Using the os._exit()
function to terminate the program immediately without performing any cleanup operations.
4. Killing the program process using system commands or task managers.
The choice of which method to use depends on the specific requirements of your program and the desired behavior upon termination.
Handling Termination Signals
In addition to manually terminating a Python program, it's also important to handle termination signals that can be sent by the operating system or user. These signals, such as SIGINT
(sent by pressing Ctrl + C
) or SIGTERM
(sent by the operating system during shutdown), allow the program to gracefully handle termination requests.
To handle termination signals in Python, you can use the signal
module. Here's an example that demonstrates how to handle SIGINT
:
import signal import sys def signal_handler(sig, frame): print("Received SIGINT, terminating...") # Perform cleanup operations here sys.exit(0) def main(): signal.signal(signal.SIGINT, signal_handler) # Your code here if __name__ == "__main__": main()
In this example, the signal.signal()
function is used to register a signal handler function for SIGINT
. When a SIGINT
signal is received, the signal_handler()
function is called, allowing you to perform any necessary cleanup operations before terminating the program.