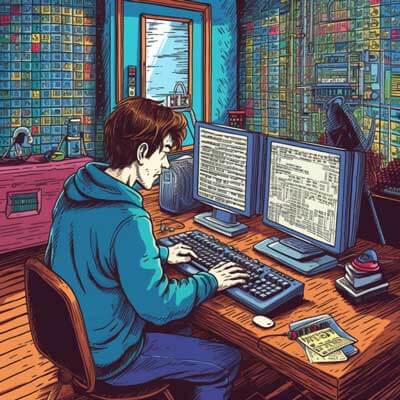
Table of Contents
Overview
Excluding test files from a Webpack build is essential to ensure that only the necessary files are included in the production bundle. Test files are typically not needed in production, and including them can lead to larger bundle sizes, longer build times, and potential exposure of test logic. This guide covers various methods to exclude test files, ensuring a cleaner and more optimized build process.
Related Article: How To Exclude Test Files In Webpack With Esbuild
Setting Up the Configuration File
Establish a basic Webpack configuration file named webpack.config.js
. This file serves as the heart of the Webpack setup, where all configurations for module resolution, output settings, and plugins will be defined.
Example of a simple configuration file:
// webpack.config.js const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, module: { rules: [], }, plugins: [], };
This initial setup specifies the entry point and output settings, creating a foundation for further configurations.
Using Exclude Patterns in Configuration
In Webpack, exclude patterns can be defined in the module rules. By using regular expressions or glob patterns, you can specify which files to ignore during the build process. The exclude
property is particularly useful when configuring loaders.
Example of excluding test files:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /__tests__/, // Exclude all files in __tests__ directory use: 'babel-loader', }, ], }, };
This setup ensures that any JavaScript files located in the __tests__
directory are not processed by Babel.
Configuring Babel-Loader for Test Files
When using Babel for transpiling JavaScript files, it's common to configure the babel-loader
to exclude test files. This can be done by applying the exclude
property within the loader configuration.
Example configuration:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /(\.test\.js$|__tests__)/, // Exclude test files use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env'], }, }, }, ], }, };
The above example excludes files that end with .test.js
or are located in any __tests__
directory, ensuring they are not included in the build process.
Related Article: How to Optimize CSS Assets with Webpack Plugin
Implementing Webpack Plugins for Exclusions
Webpack plugins can enhance the build process and help manage file exclusions more effectively. The IgnorePlugin
is one such plugin that can be configured to ignore specified files or modules.
Example of using the IgnorePlugin
:
// webpack.config.js const webpack = require('webpack'); module.exports = { plugins: [ new webpack.IgnorePlugin({ resourceRegExp: /^\.\/__tests__\/.*$/, // Ignore all files in __tests__ directory }), ], };
This configuration will ensure that Webpack ignores files located in the __tests__
directory during the build process, thus excluding them from the final bundle.
Defining Entry Points Without Test Files
When setting entry points in Webpack, it's crucial to ensure that they do not include test files. This can be managed by specifying paths that only lead to source files and excluding any directories or patterns associated with tests.
Example of defining entry points:
// webpack.config.js module.exports = { entry: { app: './src/index.js', // Only include source files }, };
This entry point only includes index.js
from the src
folder, excluding any potential test files.
Output Settings to Exclude Test Files
The output settings in Webpack dictate where the bundled files will be placed. While this does not directly exclude test files, ensuring your output path does not lead to test directories is crucial for maintaining a clean structure.
Example of output settings:
// webpack.config.js module.exports = { output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), // Output goes to 'dist' folder, no test files here }, };
This configuration directs the output files into the dist
folder, which should not contain any test files.
Creating Module Rules for Test Exclusion
Defining module rules for handling different file types is an essential part of Webpack configuration. Adding rules specifically to exclude test files helps streamline the build process.
Example of creating module rules:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /\.test\.js$/, // Exclude files that end with .test.js use: 'babel-loader', }, { test: /\.css$/, use: ['style-loader', 'css-loader'], // Include CSS files }, ], }, };
This setup processes JavaScript and CSS files while excluding any file that ends with .test.js
.
Related Article: How to Set Up Webpack SCSS Loader
Managing Source Maps
Source maps are beneficial for debugging, but when test files are excluded from the build, it's important to ensure that source maps reflect this exclusion. This prevents unnecessary clutter in debugging tools.
Example of configuring source maps:
// webpack.config.js module.exports = { devtool: 'source-map', // Enable source maps module: { rules: [ { test: /\.js$/, exclude: /\.test\.js$/, // Exclude test files from source maps use: 'babel-loader', }, ], }, };
With this configuration, Webpack generates source maps for the included files only, ensuring a clean debugging experience.
Using File-Loader for Asset Management
When managing assets like images or fonts, the file-loader
can be used to include these files in the build. To exclude specific test assets, you can configure the loader accordingly.
Example of using file-loader
:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.(png|jpg|gif|svg)$/, exclude: /__tests__/, // Exclude test assets use: 'file-loader', }, ], }, };
This configuration ensures that only non-test assets are processed by file-loader
, keeping the final bundle clean.
Optimizing Build Performance by Excluding Tests
Excluding test files can significantly improve build performance. By reducing the number of files processed, Webpack can complete the build process faster. This is especially important in larger projects with many test cases.
Example of performance optimization:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /__tests__|\.test\.js$/, // Exclude all tests use: 'babel-loader', }, ], }, optimization: { minimize: true, // Minimize the output for better performance }, };
This configuration not only excludes test files but also enables minimization, further enhancing build performance.
Switching to Production Mode with Exclusions
When preparing for production, it's common to exclude test files from the build. Webpack can be configured to switch to production mode, which optimizes the output bundle and automatically excludes unnecessary files.
Example of switching to production mode:
// webpack.config.js const mode = process.env.NODE_ENV || 'development'; module.exports = { mode, module: { rules: [ { test: /\.js$/, exclude: /__tests__|\.test\.js$/, // Exclude test files use: 'babel-loader', }, ], }, };
Related Article: How to Use Webpack Tree Shaking for Smaller Bundles
Excluding Specific Files from the Build Process
In some cases, specific files may need to be excluded from the build. This can be done using specific patterns in the loader configuration, allowing for granular control over what gets bundled.
Example of excluding specific files:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /exclude-this-file\.js$/, // Exclude a specific file use: 'babel-loader', }, ], }, };
This configuration excludes only exclude-this-file.js
while allowing all other JavaScript files to be processed.
Ignoring Test Files in the Build Process
Ignoring test files can be done using various methods in Webpack. The IgnorePlugin
or specific loader configurations are effective ways to ensure that test files do not interfere with the build output.
Example of using the IgnorePlugin
:
// webpack.config.js const webpack = require('webpack'); module.exports = { plugins: [ new webpack.IgnorePlugin(/\.test\.js$/), // Ignore all test files ], };
With this setup, Webpack will ignore any file ending with .test.js
, ensuring that they are not included in the final build.
Using Glob Patterns for File Exclusion
Glob patterns can be used for more advanced file exclusion techniques. This allows for specifying file paths and types dynamically, making it easier to manage larger projects with multiple test files.
Example of using glob patterns:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /.*\.test\.js$/, // Exclude all test files using glob pattern use: 'babel-loader', }, ], }, };
This method simplifies the exclusion of various test files while ensuring that only relevant files are included in the build.
Configuring to Skip Test Directories
When organizing a project, test files are often placed in specific directories. Webpack can be configured to skip entire directories, ensuring that all files within those directories are excluded from the build.
Example of skipping test directories:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /tests|__tests__/, // Exclude entire test directories use: 'babel-loader', }, ], }, };
With this setup, any files located in tests
or __tests__
directories will not be processed, keeping the build clean.
Related Article: How to Configure DevServer Proxy in Webpack
Selecting Loaders to Exclude Test Files
Different loaders can be applied to various file types within a Webpack configuration. By carefully choosing loaders and applying the exclude
property, you can control which files are processed.
Example of selecting loaders:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /__tests__/, // Exclude all test files use: 'babel-loader', }, { test: /\.css$/, use: ['style-loader', 'css-loader'], // Include CSS files }, ], }, };
This setup allows for JavaScript files to be excluded from Babel processing while ensuring that CSS files are handled appropriately.
Conditional Exclusions in Configuration
Conditional exclusions can be based on environment variables or other conditions. This allows for a flexible configuration that can adapt based on whether you are in development or production mode.
Example of conditional exclusions:
// webpack.config.js const isProduction = process.env.NODE_ENV === 'production'; module.exports = { module: { rules: [ { test: /\.js$/, exclude: isProduction ? /__tests__/ : /$/, // Exclude tests only in production use: 'babel-loader', }, ], }, };
In this configuration, test files are excluded only when the build is in production mode.
Modifying Configurations to Ignore Test Files
Modifying existing Webpack configurations to ignore test files can be done by adding exclude
patterns to relevant rules or using plugins to manage exclusions.
Example modification:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /__tests__/, // Add exclusion for test files use: 'babel-loader', }, { test: /\.css$/, use: ['style-loader', 'css-loader'], }, ], }, };
This modification ensures that all JavaScript files in the __tests__
directory are excluded from the build.
Verifying Exclusion of Test Files in the Bundle
After configuring Webpack, it's important to verify that test files are excluded from the final bundle. This can be done by inspecting the output directory or checking the contents of the bundle file.
Example verification process:
1. Run the Webpack build:
npx webpack --config webpack.config.js
2. Inspect the output files in the dist
directory to ensure no test files are present.
Using tools like webpack-bundle-analyzer
can also provide insights into the contents of the bundle, confirming the exclusion of unwanted files.
Related Article: How to Configure Electron with Webpack
Settings Needed for Ignoring Test Files
To effectively ignore test files in a Webpack build, the following settings are typically required:
1. Loader Configurations: Ensure that each loader that processes files has the correct exclude
patterns.
2. Plugins: Utilize plugins like IgnorePlugin
for broader exclusions.
3. Entry Points: Define entry points carefully to avoid including test files.
4. Output Settings: Verify that output paths do not include test directories.
Additional Resources
- Excluding Files from Webpack Build