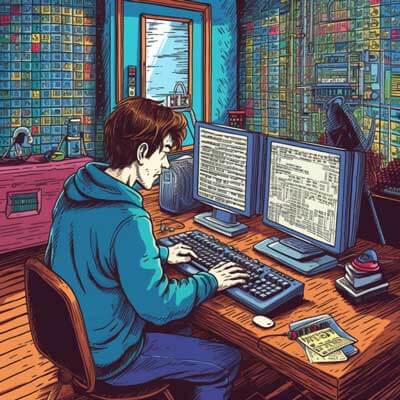
Table of Contents
Overview of Excluding Test Files with Esbuild
Excluding test files in a Webpack project that uses Esbuild as a loader can optimize the build process. Test files, usually found in directories like __tests__
or named with .spec.js
or .test.js
, are not necessary for production. By excluding them, the build time can be reduced, and the final bundle size can be minimized. This is especially useful when you want to streamline your application for deployment.
Related Article: How To Fix Unhandled Exception Cannot Find Module Webpack
Setting Up Esbuild with Your Project
To begin using Esbuild in your Webpack project, you need to install the necessary packages. Run the following command:
npm install --save-dev esbuild esbuild-loader
This command installs Esbuild and the loader that integrates Esbuild with Webpack. Next, configure Webpack to use Esbuild. Open your webpack.config.js
file and add the loader:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, loader: 'esbuild-loader', options: { loader: 'js', target: 'esnext', // Specify the JavaScript version }, }, ], }, };
This configuration tells Webpack to use Esbuild for JavaScript files. Adjust the target
option as needed for your project.
Configuring Exclude Patterns in Configuration Files
To exclude test files, modify the Webpack configuration to specify patterns for exclusion. You can use the exclude
option in the module rules. For example:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /(__tests__|\.spec\.js|\.test\.js)$/, // Exclude test files loader: 'esbuild-loader', options: { loader: 'js', target: 'esnext', }, }, ], }, };
This configuration will now ignore any files in the __tests__
folder and any files ending in .spec.js
or .test.js
.
Using Plugins & File Exclusion
Plugins can enhance Webpack's functionality, including file exclusion. The webpack-ignore-plugin
is a handy tool to ignore specific files or directories during the build process. Install the plugin:
npm install --save-dev webpack-ignore-plugin
Then, update your Webpack configuration to use the plugin:
// webpack.config.js const WebpackIgnorePlugin = require('webpack-ignore-plugin'); module.exports = { plugins: [ new WebpackIgnorePlugin({ resourceRegExp: /(__tests__|\.spec\.js|\.test\.js)$/, // Exclude test files }), ], };
This setup will ensure that the specified test files are ignored during the build.
Related Article: How to Use the Compression Plugin in Webpack
Managing Transpilation with Babel and Esbuild
In cases where Babel is also used in the project, it is essential to configure the transpilation process properly. You can set up Babel to transform only the necessary files while allowing Esbuild to handle the rest. First, ensure Babel is installed:
npm install --save-dev @babel/core @babel/preset-env babel-loader
Then, configure Babel in a .babelrc
file:
{ "presets": ["@babel/preset-env"] }
Next, update the Webpack configuration to use Babel for specific files and Esbuild for others:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /(__tests__|\.spec\.js|\.test\.js)$/, use: 'babel-loader', }, { test: /\.js$/, include: /src/, loader: 'esbuild-loader', options: { loader: 'js', target: 'esnext', }, }, ], }, };
This configuration ensures that Esbuild and Babel are used appropriately, maintaining compatibility with modern JavaScript while excluding test files.
Implementing Tree Shaking for Unused Code
Tree shaking is a technique to eliminate dead code from your production bundle. With Webpack and Esbuild, this can be achieved through the mode
setting and proper imports. Set the mode to production
in the Webpack configuration:
// webpack.config.js module.exports = { mode: 'production', };
Ensure that the code is using ES6 module syntax, as tree shaking relies on static analysis of the imports and exports to identify unused code. For example:
// src/index.js import { usedFunction } from './util'; import { unusedFunction } from './util'; // This will be removed usedFunction();
In this example, unusedFunction
will be eliminated during the tree shaking process if it is not used elsewhere.
Optimizing Source Maps
Source maps are essential for debugging, as they map your minified code back to the original source. To optimize source maps in your Webpack configuration, set the devtool
option:
// webpack.config.js module.exports = { devtool: 'source-map', };
This setting generates source maps that provide the best debugging experience in production builds. For development, you might want to use cheap-module-source-map
, which offers faster builds:
// webpack.config.js module.exports = { devtool: 'cheap-module-source-map', };
Adjusting the source map option allows you to balance between build speed and debugging capabilities.
Handling Development Mode Exclusions
In development mode, excluding test files can still be valuable. However, you might want to include them temporarily for debugging. You can set up Webpack to conditionally exclude files based on the environment:
// webpack.config.js const isDevelopment = process.env.NODE_ENV === 'development'; module.exports = { module: { rules: [ { test: /\.js$/, exclude: isDevelopment ? /(__tests__|\.spec\.js|\.test\.js)$/ : [], loader: 'esbuild-loader', options: { loader: 'js', target: 'esnext', }, }, ], }, };
This configuration allows test files to be included when running in development mode, enabling easier debugging.
Related Article: How to Use the Copy Webpack Plugin
Production Mode Settings
For production builds, the exclusion of test files is crucial. You can enforce these exclusions by structuring your Webpack configuration to differentiate between development and production:
// webpack.config.js const isProduction = process.env.NODE_ENV === 'production'; module.exports = { module: { rules: [ { test: /\.js$/, exclude: isProduction ? /(__tests__|\.spec\.js|\.test\.js)$/ : [], loader: 'esbuild-loader', options: { loader: 'js', target: 'esnext', }, }, ], }, };
Code Splitting Techniques
Code splitting allows you to break your application into smaller chunks, which can be loaded on demand. This can significantly improve load times. Webpack provides built-in support for code splitting using dynamic imports:
// src/index.js import('./module') .then(module => { module.default(); }) .catch(err => { console.error('Error loading the module', err); });
When using dynamic imports, Webpack will create separate bundles for the imported modules. This ensures that only the necessary code is loaded, improving performance and user experience.
Excluding Directories in Your Configuration
Excluding entire directories can simplify your Webpack configuration. You can easily exclude directories by specifying patterns in the exclude
option:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.js$/, exclude: /node_modules|__tests__/, // Exclude these directories loader: 'esbuild-loader', options: { loader: 'js', target: 'esnext', }, }, ], }, };
This pattern excludes all files in the node_modules
and __tests__
directories from being processed by Esbuild.
Excluding Specific File Types with Patterns
Exclusion patterns can be customized to target specific file types. For instance, if you want to exclude all .scss
files, you can add a pattern to the exclude
option:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.(js|jsx)$/, exclude: /\.scss$/, // Exclude SCSS files loader: 'esbuild-loader', options: { loader: 'jsx', target: 'esnext', }, }, ], }, };
This configuration ensures that any .scss
files are ignored during the build process.
Related Article: How to Bypass a Router with Webpack Proxy
Conditional Exclusion of Test Files
Conditional exclusion allows for flexibility in how test files are handled. By checking environment variables or specific conditions, you can adjust your Webpack configuration accordingly:
// webpack.config.js const shouldExcludeTests = process.env.EXCLUDE_TESTS === 'true'; module.exports = { module: { rules: [ { test: /\.js$/, exclude: shouldExcludeTests ? /(__tests__|\.spec\.js|\.test\.js)$/ : [], loader: 'esbuild-loader', options: { loader: 'js', target: 'esnext', }, }, ], }, };
In this example, setting the EXCLUDE_TESTS
environment variable to true
will exclude the test files. This approach provides control over how test files are included or excluded.
Comparing Esbuild and Other Build Tools
When comparing Esbuild to other build tools like Babel and Webpack, Esbuild stands out for its speed and simplicity. While Babel focuses on transpilation, Esbuild combines both transpilation and bundling into one tool. This results in faster build times due to its native Go implementation.
Webpack, on the other hand, offers comprehensive features for asset management and is highly configurable. However, the configuration can be more complex compared to Esbuild. Choosing between these tools often depends on project requirements and team familiarity.
Improving Build Performance with Esbuild
To enhance build performance with Esbuild, consider the following strategies:
1. Parallel Builds: Esbuild supports parallel processing, allowing multiple files to be built at the same time. This can drastically reduce build times.
2. Incremental Builds: Use Esbuild’s incremental build features to only rebuild modified files, speeding up the development process.
3. Minification: Enable minification in production builds to reduce bundle sizes.
Adjust the Webpack configuration to leverage these features, ensuring an optimized build process.
Managing Test Files in Your Project Workflow
Maintaining a clear workflow for managing test files is crucial. Organize test files in dedicated directories, such as __tests__
, and maintain consistent naming conventions. When running tests, separate test runs from the main build process, ensuring that test files are only included as needed.
Using tools like Jest or Mocha for testing can help in managing test files effectively. Integrate these tools within your project setup, ensuring that test files are executed in isolation from the production build.
Related Article: How to Configure DevServer Proxy in Webpack
Additional Resources
- Excluding Test Files in Webpack