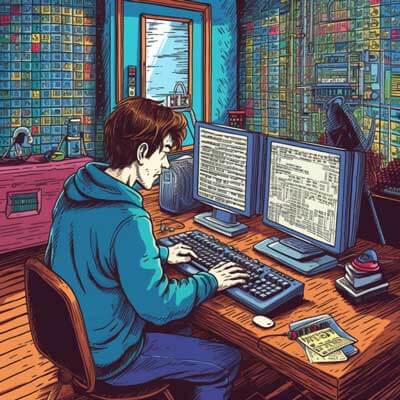
Table of Contents
To extract a parameter value from a query string in React, you can use the built-in JavaScript methods for manipulating URLs and query strings. Here are two possible approaches:
1. Using the URLSearchParams API
The URLSearchParams interface provides methods for working with the query string of a URL. You can create a new instance of URLSearchParams by passing the query string as a parameter. Then, you can use the get() method to retrieve the value of a specific parameter.
Here's an example of how you can extract a parameter value from a query string using the URLSearchParams API:
const queryString = window.location.search; const urlParams = new URLSearchParams(queryString); const paramValue = urlParams.get('paramName'); console.log(paramValue);
In this example, window.location.search
retrieves the query string from the current URL. Then, we create a new instance of URLSearchParams
using the query string. Finally, we use the get()
method to retrieve the value of the specified parameter ("paramName" in this case). The value is stored in the paramValue
variable and logged to the console.
Related Article: How to Fetch Post JSON Data in Javascript
2. Using a Custom Function
If you prefer a more manual approach, you can write a custom function to extract the parameter value from a query string. This approach involves splitting the query string into individual key-value pairs and then searching for the desired parameter.
Here's an example of a custom function to extract a parameter value from a query string:
function getParamFromQueryString(queryString, paramName) { const params = queryString.slice(1).split('&'); for (let i = 0; i < params.length; i++) { const [key, value] = params[i].split('='); if (key === paramName) { return decodeURIComponent(value); } } return null; } const queryString = window.location.search; const paramValue = getParamFromQueryString(queryString, 'paramName'); console.log(paramValue);
In this example, the getParamFromQueryString
function takes two parameters: the query string and the parameter name. It splits the query string into individual key-value pairs and iterates over them. If the key matches the specified parameter name, the corresponding value is returned after decoding it using decodeURIComponent()
. If no matching parameter is found, the function returns null.
Both approaches mentioned above are valid ways to extract parameter values from query strings in React. You can choose the one that suits your preference and project requirements.
Best Practices
Related Article: How To Add Days To a Date In Javascript
When working with query strings in React, it's a good practice to:
- Always validate and sanitize the extracted parameter value to ensure it is safe to use in your application.
- Handle cases where the parameter value is missing or not found, and provide appropriate fallback behavior.
- Consider using a library like query-string
or qs
for more advanced query string parsing and manipulation.