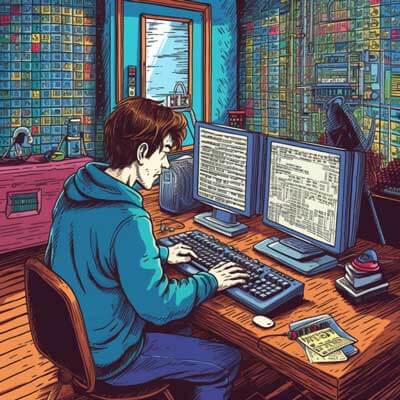
Table of Contents
Overview of List Comprehension
List comprehension allows you to create new lists by iterating over existing ones and applying certain conditions. It provides a way to filter elements from a list based on specific criteria. With list comprehension, you can perform filtering, transformation, and combination of elements in a single line of code, making your code more readable and expressive.
Related Article: Python Priority Queue Tutorial
Syntax
The syntax for list comprehension consists of three parts: the expression, the iteration, and the optional condition.
The expression is the value that will be included in the new list. The iteration defines the source list and the variable that represents each element in the source list. The condition, which is optional, is used to filter elements based on a specific criterion.
The general syntax for list comprehension is as follows:
new_list = [expression for variable in source_list if condition]
Let's break down the syntax:
- new_list
: The new list that will be created.
- expression
: The value or transformation that will be applied to each element in the source list.
- variable
: The variable that represents each element in the source list.
- source_list
: The list from which elements will be selected.
- if condition
: The optional condition that filters elements based on a specific criterion.
Code Snippet
Let's consider an example where we have a list of numbers and we want to create a new list containing only the even numbers from the original list using list comprehension:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = [num for num in numbers if num % 2 == 0] print(even_numbers)
Output:
[2, 4, 6, 8, 10]
In this example, we use list comprehension to iterate over each number in the numbers
list. The condition if num % 2 == 0
filters out the odd numbers, and the expression num
adds the even numbers to the even_numbers
list.
Using the Lambda Function for List Filtering
In addition to list comprehension, you can also use lambda functions to filter elements from a list. Lambda functions are anonymous functions that can be defined in a single line. They are commonly used with higher-order functions like filter()
and map()
.
Using lambda functions for list filtering can provide a more flexible and concise way to specify the filtering condition. It allows you to define the filtering logic inline without the need for a separate function definition.
Related Article: Tutorial: Django + MongoDB, ElasticSearch & Message Brokers
Syntax for the Lambda Function
The syntax for defining a lambda function is as follows:
lambda arguments: expression
Let's break down the syntax:
- lambda
: The keyword used to define a lambda function.
- arguments
: The arguments that the lambda function takes.
- expression
: The expression that is evaluated and returned by the lambda function.
Code Snippet for Lambda Function
Let's consider the same example as before, where we have a list of numbers and we want to filter out the even numbers using a lambda function with the filter()
function:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] even_numbers = list(filter(lambda num: num % 2 == 0, numbers)) print(even_numbers)
Output:
[2, 4, 6, 8, 10]
In this example, we use the filter()
function along with a lambda function to filter out the even numbers from the numbers
list. The lambda function lambda num: num % 2 == 0
defines the filtering condition, and the filter()
function applies this condition to each element in the numbers
list.
The Filter Function
The filter()
function is a built-in function in Python that allows you to filter elements from a sequence based on a specific criterion. It takes two arguments: the filtering function and the sequence. The filtering function defines the condition for filtering, and the sequence is the source of the elements to be filtered.
The filter()
function returns an iterator that contains the filtered elements from the sequence. To obtain a list of the filtered elements, you can convert the iterator to a list using the list()
function.
Applying Predicate with the Filter Function
In order to use the filter()
function, you need to define a filtering function or a lambda function that acts as a predicate. A predicate is a function that returns either True
or False
based on a given input.
The filtering function or lambda function should take an element from the sequence as input and return True
if the element satisfies the filtering condition, or False
otherwise.
Related Article: How to Determine the Length of an Array in Python
Code Snippet for the Filter Function
Let's consider the same example as before, where we have a list of numbers and we want to filter out the even numbers using the filter()
function:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] def is_even(num): return num % 2 == 0 even_numbers = list(filter(is_even, numbers)) print(even_numbers)
Output:
[2, 4, 6, 8, 10]
In this example, we define the is_even()
function as the filtering function. The function checks if a number is even by using the modulo operator %
to divide the number by 2 and checking if the remainder is 0. The filter()
function then applies the is_even()
function to each element in the numbers
list and returns an iterator containing the even numbers. We convert the iterator to a list using the list()
function to obtain the final result.
Additional Resources
- Python List Comprehension: The Complete Guide