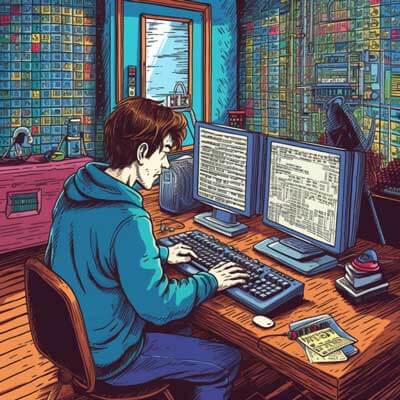
Table of Contents
Angular Webpack Plugin Initialization Error occurs when there are issues during the setup of Angular projects using Webpack. This error can manifest in various forms, often indicating problems with the plugin configuration or environment settings. Developers may encounter messages indicating that the plugin could not be initialized or that certain configurations are missing or incorrect. Resolving these issues is crucial for ensuring that the Angular application builds and runs correctly.
What is the Angular Webpack Plugin
The Angular Webpack Plugin is a tool that integrates Angular applications with Webpack, a module bundler. This plugin facilitates the building of Angular applications by managing the compilation and bundling of TypeScript files, styles, and assets. It enhances the development experience by providing features such as hot module replacement and optimization for production builds. The plugin makes it easier to work with Angular's architecture by automatically configuring Webpack settings tailored to Angular's requirements.
Related Article: How to Fix webpack not recognized as a command error
Common Configuration Issues and Fixes
Configuration issues often lead to the Angular Webpack Plugin Initialization Error. Some common problems include:
1. Incorrect Angular CLI Version: Ensure the Angular CLI version matches the Angular project version. Mismatched versions can lead to compatibility issues.
ng --version
2. Missing Required Packages: Verify that all necessary dependencies, like @angular/compiler-cli
, are installed.
npm install @angular/compiler-cli --save-dev
3. Webpack Configuration Errors: Check the Webpack configuration file for syntax errors or incorrect settings.
// webpack.config.js module.exports = { mode: 'development', entry: './src/main.ts', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') }, resolve: { extensions: ['.ts', '.js'] }, module: { rules: [ { test: /\.ts$/, use: 'ts-loader', exclude: /node_modules/ } ] } };
4. Path Issues: Ensure that paths in your configuration point to the correct files. Incorrect paths can lead to module resolution errors.
Configuring for Angular Projects
Setting up Webpack for Angular projects involves creating a webpack.config.js
file tailored to Angular's needs. The configuration should include entry points, output settings, and module rules for handling TypeScript files. Here’s a basic example:
// webpack.config.js const path = require('path'); module.exports = { entry: './src/main.ts', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') }, resolve: { extensions: ['.ts', '.js'] }, module: { rules: [ { test: /\.ts$/, use: 'ts-loader', exclude: /node_modules/ } ] }, devtool: 'source-map', };
This setup specifies the entry point as main.ts
, directs the output to the dist
folder, and tells Webpack to use ts-loader
for loading TypeScript files.
Babel Loader Setup for Angular
Incorporating Babel can enhance compatibility with older browsers by transpiling modern JavaScript. To set up Babel in an Angular project, follow these steps:
1. Install Babel and its loader:
npm install --save-dev babel-loader @babel/core @babel/preset-env
2. Update the Webpack configuration to include Babel:
// webpack.config.js module.exports = { // ... other settings ... module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env'] } } } ] } };
This configuration tells Webpack to use Babel for transpiling JavaScript files, making your application more compatible across different browsers.
Related Article: How to Configure Webpack for Expo Projects
Hot Module Replacement
Hot Module Replacement (HMR) allows modules to be updated in real time without a full page reload. This is crucial for improving development speed and efficiency. To enable HMR in an Angular project, follow these steps:
1. Install the necessary dependencies:
npm install --save-dev webpack-dev-server
2. Update the Webpack configuration to enable HMR:
// webpack.config.js module.exports = { // ... other settings ... devServer: { contentBase: './dist', hot: true, }, };
3. Add HMR support to your main entry file:
// main.ts if (module.hot) { module.hot.accept(); }
With HMR enabled, changes made to your application will be reflected instantly without needing to refresh the browser, significantly accelerating the development process.
Using Source Maps for Debugging
Source maps are essential for debugging in development environments. They map the transformed code back to the original source code, making it easier to trace errors. To enable source maps in your Webpack configuration, add the devtool
property:
// webpack.config.js module.exports = { // ... other settings ... devtool: 'inline-source-map', };
With this setting, if an error occurs, the browser will display the original source files instead of the minified or transpiled versions. This dramatically improves the debugging experience.
Build Optimization Techniques
Optimizing the build process is crucial for enhancing application performance. Some common techniques include:
1. Minification: Remove unnecessary whitespace and comments to reduce file size.
npm install --save-dev terser-webpack-plugin
2. Tree Shaking: Eliminate unused code during the build process. Ensure that your code is written in a module format (ES6 modules) to take full advantage of tree shaking.
3. Code Splitting: Separate code into smaller chunks that can be loaded on demand, reducing the initial load time.
To implement these techniques, update your Webpack configuration as follows:
// webpack.config.js const TerserPlugin = require('terser-webpack-plugin'); module.exports = { optimization: { minimize: true, minimizer: [new TerserPlugin()], splitChunks: { chunks: 'all', }, }, };
These optimizations will enhance the performance of your Angular application, resulting in faster load times and a better user experience.
Tree Shaking for Better Performance
Tree shaking is a feature that allows Webpack to remove unused code from the final bundle. This is essential for reducing the bundle size and improving load times. To enable tree shaking, follow these guidelines:
1. Use ES6 module syntax (import/export) instead of CommonJS (require
).
2. Set the mode to 'production' in your Webpack configuration:
// webpack.config.js module.exports = { mode: 'production', // ... other settings ... };
3. Ensure that your dependencies are also tree-shakable. This typically involves using libraries that support ES modules.
Related Article: How to Use the Clean Webpack Plugin
Code Splitting Strategies
Code splitting allows you to split your application into smaller bundles that can be loaded on demand. This is particularly useful for large applications where loading everything at once can lead to slow performance. Here are some strategies for implementing code splitting:
1. Entry Points: Define multiple entry points in your Webpack configuration to create separate bundles.
// webpack.config.js module.exports = { entry: { app: './src/app.ts', vendor: './src/vendor.ts', }, // ... other settings ... };
2. Dynamic Imports: Use dynamic imports to load modules only when needed.
// example.ts import('./module').then(module => { module.default(); });
3. SplitChunksPlugin: Use this built-in Webpack plugin to automatically split code based on certain criteria.
// webpack.config.js module.exports = { optimization: { splitChunks: { chunks: 'all', }, }, };
Implementing these strategies will lead to improved load times and a better user experience by ensuring that only the necessary code is loaded when needed.
Plugin System
Webpack's plugin system is a useful way to extend its functionality. Plugins can perform a wide range of tasks, from optimizing builds to managing assets. To use a plugin, you need to install it and include it in your Webpack configuration. Here’s an example of how to use the HtmlWebpackPlugin
:
1. Install the plugin:
npm install --save-dev html-webpack-plugin
2. Update the Webpack configuration to include the plugin:
const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ title: 'My App', template: './src/index.html', }), ], };
This setup automatically generates an index.html
file that includes your bundled JavaScript files, simplifying the process of managing HTML files in your project.
Using Angular CLI with Webpack
The Angular CLI abstracts much of the configuration needed for Webpack, making it easier to set up and manage Angular projects. When you create a new Angular project using the CLI, it automatically configures Webpack for you. To create a new project, use the following command:
ng new my-angular-app
The CLI handles the Webpack configuration behind the scenes, allowing you to focus on developing your application. You can still extend or modify the Webpack configuration if needed by using custom builders or ejecting the configuration.
Troubleshooting Plugin Errors
When dealing with plugin errors in Webpack, consider the following approaches to troubleshoot:
1. Check Compatibility: Ensure that the plugin version is compatible with your Webpack version. Mismatched versions can lead to unexpected behavior.
2. Review Documentation: Consult the plugin's documentation for any specific configuration requirements or known issues.
3. Examine Error Messages: Pay close attention to the error messages in the console. They often provide valuable clues about what went wrong.
4. Simplify Configuration: Temporarily remove other plugins or complex configurations to isolate the issue and determine if a specific plugin is causing the problem.
Related Article: How to Bypass a Router with Webpack Proxy
The Dependency Graph
The dependency graph is a representation of all modules and their dependencies in a project. Webpack builds this graph to understand how modules relate to each other. When configuring your Angular project, it's essential to have a clear understanding of how dependencies are structured. This helps in optimizing the build process and ensuring that modules are loaded in the correct order.
You can visualize the dependency graph using tools like webpack-bundle-analyzer
:
npm install --save-dev webpack-bundle-analyzer
Then, add it to your Webpack configuration:
const BundleAnalyzerPlugin = require('webpack-bundle-analyzer').BundleAnalyzerPlugin; module.exports = { plugins: [ new BundleAnalyzerPlugin(), ], };
Running your build will generate a visual representation of your application's dependency graph, allowing you to identify large or unnecessary dependencies.
Module Federation Concepts
Module Federation is a feature introduced in Webpack 5 that allows multiple Webpack builds to share code dynamically at runtime. This is particularly useful for micro-frontend architectures. To set up Module Federation, you need to define a ModuleFederationPlugin
configuration in your Webpack setup.
1. Install the required dependencies:
npm install --save-dev webpack@5
2. Configure the ModuleFederationPlugin
in your Webpack configuration:
const ModuleFederationPlugin = require('webpack/lib/container/ModuleFederationPlugin'); module.exports = { plugins: [ new ModuleFederationPlugin({ name: 'app1', filename: 'remoteEntry.js', exposes: { './Component': './src/Component', }, shared: { react: { singleton: true }, 'react-dom': { singleton: true }, }, }), ], };
This setup allows app1
to expose its Component
module for use in other applications. Understanding Module Federation can significantly enhance how applications interact and share code.
Running the Development Server
To run the development server for your Angular application, you typically use the Angular CLI. The command is simple and straightforward:
ng serve
This command compiles the application and starts a development server, allowing you to view your application in the browser. The development server supports features like live reloading, which automatically refreshes the page when you make changes to your code.
In cases where you are using a custom Webpack setup, you can run the Webpack development server using:
webpack serve --config webpack.config.js
This command starts the Webpack development server, allowing you to see your changes in real time while benefiting from the configuration specified in your webpack.config.js
file.
Additional Resources
- Fixing Emit Errors in Webpack